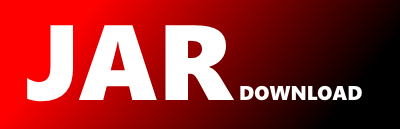
org.cthul.matchers.object.InstanceOf Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cthul-matchers Show documentation
Show all versions of cthul-matchers Show documentation
Provides hamcrest.org matchers for strings and exceptions,
allows matching code blocks, and provides several utilities for
combining matchers.
The newest version!
package org.cthul.matchers.object;
import org.cthul.matchers.chain.AndChainMatcher;
import org.cthul.matchers.diagnose.QuickDiagnosingMatcherBase;
import org.hamcrest.*;
import org.hamcrest.core.Is;
import org.hamcrest.core.IsInstanceOf;
/**
* Example:
* {@code
* Object o = "foobar;
* assertThat(o, isA(String.class).thatIs(foo()).and(bar());
* }
*/
public class InstanceOf extends QuickDiagnosingMatcherBase
© 2015 - 2025 Weber Informatics LLC | Privacy Policy