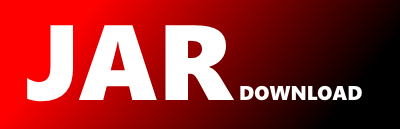
org.cthul.objects.instance.Instances Maven / Gradle / Ivy
The newest version!
package org.cthul.objects.instance;
import java.lang.reflect.Array;
import java.lang.reflect.Constructor;
import java.lang.reflect.Field;
import java.lang.reflect.Method;
import java.lang.reflect.Modifier;
import java.util.Arrays;
import org.cthul.objects.Boxing;
import org.cthul.objects.reflection.Signatures;
/**
*
*/
public class Instances {
public static Object newInstance(Instance inst) {
return getInstance(null, inst);
}
public static Object getInstance(InstanceMap map, Instance inst) {
return getInstance(map, inst.key(), inst.impl(), inst.factory(), null);
}
public static Object[] getInstances(InstanceMap map, Instance[] inst) {
Object[] result = new Object[inst.length];
for (int i = 0; i < result.length; i++) {
result[i] = getInstance(map, inst[i]);
}
return result;
}
public static Object newInstance(Class> impl, String factory, Arg[] args) {
return getInstance(null, null, impl, factory, args);
}
public static Object newInstance(Class> impl, String factory, Arg[] args, Object[] moreValues) {
return getInstance(null, null, impl, factory, args, moreValues);
}
public static Object getInstance(InstanceMap map, String key, Class> impl, String factory, Arg[] args) {
return getInstance(map, key, impl, factory, args, null);
}
public static Object getInstance(InstanceMap map, String key, Class> impl, String factory, Arg[] args, Object[] moreValues) {
if (map != null) {
if (!isDefault(key, "")) {
Object o = map.get(key);
if (o != null) return o;
} else if (isDefault(factory, "") && isEmpty(args)) {
Object o = map.get(impl);
if (o != null) return o;
}
}
if (args == null) {
return newInstance(map, impl, factory, null, moreValues);
}
Object[] argValues = new Object[lengthOf(args)];
Class[] argTypes = new Class[argValues.length];
fillArgs(map, args, argValues, argTypes, moreValues);
return newInstance(map, impl, factory, argTypes, argValues);
}
public static void fillArgs(InstanceMap map, Arg[] args, Object[] values, Class[] types, Object[] moreValues) {
if (isEmpty(args)) return;
for (int i = 0; i < args.length; i++) {
fillArg(map, args, i, values, types, moreValues);
}
}
private static void fillArg(InstanceMap map, Arg[] args, int i, Object[] values, Class[] types, Object[] moreValues) {
Arg a = args[i];
Object value = null;
Class> type = null;
boolean storeValue = false;
if (!isDefault(a.key(), "")) {
if (map != null) {
value = map.get(a.key());
if (value != null) {
type = value.getClass();
storeValue = true;
}
} else if (moreValues != null) {
String[] keys = a.key().split(",");
Object[] v = new Object[keys.length];
try {
for (int j = 0; j < keys.length; j++) {
int index = Integer.parseInt(keys[j]);
v[j] = moreValues[index];
}
value = v;
type = Object[].class;
} catch (NumberFormatException e) {
}
}
}
Object[] prims = {a.x(), a.b(), a.c(), a.d(), a.f(), a.i(), a.l(), a.s(), a.str(), a.o()};
for (Object p: prims) {
if (!isEmpty(p)) {
if (type != null) {
throw new IllegalArgumentException(
"Ambiguous @Arg, got "
+ type.getSimpleName()
+ " and " + p.getClass().getSimpleName());
}
if (p instanceof Instance[]) {
p = getInstances(map, (Instance[]) p);
}
value = p;
type = value.getClass();
}
}
Class> compType = a.arrayOf();
if (compType == void.class && value != null) {
int l = lengthOf(value);
if (l == 1) {
value = Array.get(value, 0);
type = value != null ? value.getClass() : null;
}
} else if (value == null) {
value = Array.newInstance(compType, 0);
type = value.getClass();
} else if (Boxing.boxingType(compType) != null) {
type = Array.newInstance(compType, 0).getClass();
value = Boxing.as(value, type);
} else {
Class
© 2015 - 2025 Weber Informatics LLC | Privacy Policy