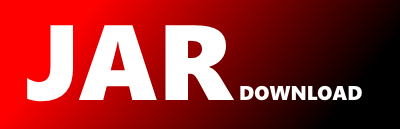
org.cthul.objects.instance.UniqueValueCache Maven / Gradle / Ivy
The newest version!
package org.cthul.objects.instance;
import java.lang.ref.Reference;
import java.lang.ref.ReferenceQueue;
import java.lang.ref.SoftReference;
import java.lang.ref.WeakReference;
import java.util.concurrent.ConcurrentHashMap;
import java.util.concurrent.ConcurrentMap;
public abstract class UniqueValueCache {
private final ReferenceQueue
© 2015 - 2025 Weber Informatics LLC | Privacy Policy