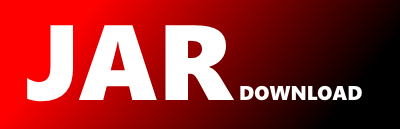
org.cthul.strings.format.MatchResultsBase Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cthul-strings Show documentation
Show all versions of cthul-strings Show documentation
Functions for converting strings from and to various formats,
such as roman numbers, alpha indices, Java identifiers,
and format strings.
The newest version!
package org.cthul.strings.format;
import java.util.*;
import org.cthul.strings.format.ConversionPattern.Intermediate;
/**
*
* @author Arian Treffer
*/
public abstract class MatchResultsBase extends AbstractArgs implements MatchResults {
/**
* Converts an {@link Intermediate} into the final value.
* @param o
* @return final value
*/
protected static Object complete(Object o) {
if (o instanceof Intermediate) {
o = ((Intermediate) o).complete();
}
return o;
}
protected static List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy