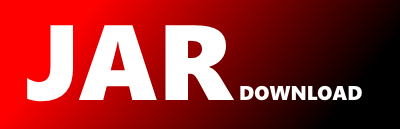
org.ctoolkit.restapi.client.agent.model.MigrationSetKindOperations Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ctoolkit-client-agent Show documentation
Show all versions of ctoolkit-client-agent Show documentation
CtoolkiT REST API Client Implementation of Cloud Toolkit Migration Agent
package org.ctoolkit.restapi.client.agent.model;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlElement;
import java.io.Serializable;
import java.util.ArrayList;
import java.util.Iterator;
import java.util.List;
/**
* The bean holding migration set kind operations
*
* @author Jozef Pohorelec
*/
@XmlAccessorType( XmlAccessType.FIELD )
public class MigrationSetKindOperations
implements Serializable
{
@XmlElement( name = "add" )
private List add;
@XmlElement( name = "change" )
private List change;
@XmlElement( name = "remove" )
private List remove;
public List getAdd()
{
if ( add == null )
{
add = new ArrayList();
}
return add;
}
public void setAdd( List add )
{
this.add = add;
}
public List getChange()
{
if ( change == null )
{
change = new ArrayList();
}
return change;
}
public void setChange( List change )
{
this.change = change;
}
public List getRemove()
{
if ( remove == null )
{
remove = new ArrayList();
}
return remove;
}
public void setRemove( List remove )
{
this.remove = remove;
}
public void addOperationRemove( String kind, String property )
{
removeOperationRemove( kind, property );
MigrationSetKindOperationRemove op = new MigrationSetKindOperationRemove();
op.setKind( kind );
op.setProperty( property );
getRemove().add( op );
}
public void removeOperationRemove( String kind, String property )
{
Iterator it = getRemove().iterator();
while ( it.hasNext() )
{
MigrationSetKindOperationRemove op = it.next();
if ( kind.equals( op.getKind() ) && property.equals( op.getProperty() ) )
{
it.remove();
}
}
}
@Override
public String toString()
{
return "MigrationSetKindOperations{" +
"add=" + add +
", change=" + change +
", remove=" + remove +
'}';
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy