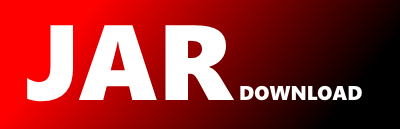
org.d2ab.sequence.ListSequence Maven / Gradle / Ivy
/*
* Copyright 2016 Daniel Skogquist Åborg
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.d2ab.sequence;
import org.d2ab.collection.ChainedList;
import org.d2ab.collection.FilteredList;
import org.d2ab.collection.MappedList;
import org.d2ab.collection.ReverseList;
import java.util.*;
import java.util.function.Function;
import java.util.function.Predicate;
import java.util.stream.Stream;
import static java.util.Collections.emptyList;
import static java.util.Collections.singletonList;
/**
* A {@link Sequence} backed by a {@link List}. Implements certain operations on {@link Sequence} in a more performant
* way due to the {@link List} backing. This class should normally not be used directly as e.g.
* {@link Sequence#from(Iterable)} and other methods return this class directly where appropriate.
*/
public class ListSequence implements Sequence {
private List list;
public static Sequence empty() {
return from(emptyList());
}
public static Sequence of(T item) {
return from(singletonList(item));
}
@SuppressWarnings("unchecked")
public static Sequence of(T... items) {
return from(Arrays.asList(items));
}
public static Sequence from(List list) {
return new ListSequence<>(list);
}
public static Sequence concat(List... lists) {
return from(ChainedList.from(lists));
}
public static Sequence concat(List> lists) {
return from(ChainedList.from(lists));
}
private ListSequence(List list) {
this.list = list;
}
@Override
public Iterator iterator() {
return list.iterator();
}
@Override
public List toList() {
return new ArrayList<>(list);
}
@Override
public List asList() {
return list;
}
@Override
public > U collectInto(U collection) {
collection.addAll(list);
return collection;
}
@Override
public void clear() {
list.clear();
}
@Override
public long size() {
return list.size();
}
@Override
public Stream stream() {
return list.stream();
}
@Override
public boolean isEmpty() {
return list.isEmpty();
}
@Override
public boolean contains(T item) {
return list.contains(item);
}
@Override
public Optional at(long index) {
if (index >= list.size())
return Optional.empty();
return Optional.of(list.get((int) index));
}
@Override
public Optional last() {
if (list.size() < 1)
return Optional.empty();
return Optional.of(list.get(list.size() - 1));
}
@Override
public Sequence reverse() {
return from(ReverseList.from(list));
}
@Override
public Sequence filter(Predicate super T> predicate) {
return from(FilteredList.from(list, predicate));
}
@Override
public Sequence map(Function super T, ? extends U> mapper) {
return from(MappedList.from(list, mapper));
}
@SuppressWarnings("unchecked")
@Override
public Sequence append(Iterable iterable) {
if (iterable instanceof List)
return from(ChainedList.from(list, (List) iterable));
return Sequence.concat(this, iterable);
}
@SuppressWarnings("unchecked")
@Override
public Sequence append(T... items) {
return append(Arrays.asList(items));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy