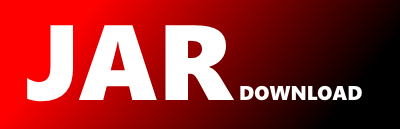
dafny.Array Maven / Gradle / Ivy
// Copyright by the contributors to the Dafny Project
// SPDX-License-Identifier: MIT
package dafny;
import java.util.List;
/**
* A wrapper for an array that may be of primitive type. Essentially acts as
* one "big box" for many primitives, as an alternative to putting each in their
* own box. Much faster than using {@link java.lang.reflect.Array} operations
* to operate on possibly-primitive arrays.
*
* This isn't used by generated Dafny code, which directly uses values of type
* Object when the element type isn't known, relying on a {@link TypeDescriptor} passed
* in. It's much more pleasant to use, and more type-safe, than the bare
* {@link TypeDescriptor} operations, however, so extern implementors may be interested.
* It is also used to implement {@link DafnySequence}.
*
* @param The type of the elements in the array, or if that type is
* primitive, the boxed version of that type (e.g., {@link Integer}
* for int).
*/
public final class Array implements Cloneable {
private final TypeDescriptor eltType;
private final Object array;
private Array(TypeDescriptor eltType, Object array) {
this.eltType = eltType;
this.array = array;
}
public static Array newArray(TypeDescriptor eltType, int length) {
return new Array(eltType, eltType.newArray(length));
}
public static Array fromList(TypeDescriptor eltType, List elements) {
return new Array(eltType, eltType.toArray(elements));
}
public TypeDescriptor elementType() {
return eltType;
}
public Object unwrap() {
return array;
}
public T get(int index) {
return eltType.getArrayElement(array, index);
}
public void set(int index, T value) {
eltType.setArrayElement(array, index, value);
}
public int length() {
return eltType.getArrayLength(array);
}
public void fill(T value) {
eltType.fillArray(array, value);
}
public Array copy() {
return new Array(eltType, eltType.cloneArray(array));
}
@SuppressWarnings("SuspiciousSystemArraycopy")
public void copy(int offset, Array to, int toOffset, int length) {
System.arraycopy(this.array, offset, to.array, toOffset, length);
}
public Array copyOfRange(int lo, int hi) {
Array newArray = newArray(eltType, hi - lo);
copy(lo, newArray, 0, hi - lo);
return newArray;
}
public boolean shallowEquals(Array other) {
return eltType.arrayShallowEquals(this.array, other.array);
}
public static Array wrap(TypeDescriptor eltType, Object array) {
return new Array(eltType, array);
}
// Note: We need the element type passed in here because otherwise the
// actual type of the array might not be T[] but S[] where S is a subclass
// of T.
public static Array wrap(TypeDescriptor eltType, T[] array) {
return new Array(eltType, array);
}
public static Array wrap(byte[] array) {
return new Array(TypeDescriptor.BYTE, array);
}
public static Array wrap(short[] array) {
return new Array(TypeDescriptor.SHORT, array);
}
public static Array wrap(int[] array) {
return new Array(TypeDescriptor.INT, array);
}
public static Array wrap(long[] array) {
return new Array(TypeDescriptor.LONG, array);
}
public static Array wrap(boolean[] array) {
return new Array(TypeDescriptor.BOOLEAN, array);
}
public static Array wrap(char[] array) {
return new Array(TypeDescriptor.CHAR, array);
}
public static Object unwrap(Array> array) {
return array.unwrap();
}
@SuppressWarnings("unchecked")
public static T[] unwrapObjects(Array array) {
return (T[]) array.array;
}
public static byte[] unwrapBytes(Array array) {
return (byte[]) array.array;
}
public static short[] unwrapShorts(Array array) {
return (short[]) array.array;
}
public static int[] unwrapInts(Array array) {
return (int[]) array.array;
}
public static long[] unwrapLongs(Array array) {
return (long[]) array.array;
}
public static boolean[] unwrapBooleans(Array array) {
return (boolean[]) array.array;
}
public static char[] unwrapChars(Array array) {
return (char[]) array.array;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy