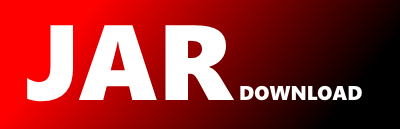
dafny.DafnyMultiset Maven / Gradle / Ivy
// Copyright by the contributors to the Dafny Project
// SPDX-License-Identifier: MIT
package dafny;
import java.math.BigInteger;
import java.util.*;
public class DafnyMultiset {
/*
Invariant: forall x. m.get(x) == null || m.get(x) > 0
As in Java, null is allowed as a key
*/
private Map innerMap;
public DafnyMultiset() {
innerMap = new HashMap<>();
}
// Requires that all values in m are non-negative
public DafnyMultiset(Map m) {
assert m != null : "Precondition Violation";
innerMap = new HashMap<>();
for (Map.Entry e : m.entrySet()) {
BigInteger n = e.getValue();
int cmp = n.compareTo(BigInteger.ZERO);
assert 0 <= cmp : "Precondition Violation";
if (0 < cmp) {
innerMap.put(e.getKey(), n);
}
}
}
public DafnyMultiset(Set s) {
assert s != null : "Precondition Violation";
innerMap = new HashMap<>();
for (T t : s) {
incrementMultiplicity(t, BigInteger.ONE);
}
}
public DafnyMultiset(Collection c) {
assert c != null : "Precondition Violation";
innerMap = new HashMap<>();
for (T t : c) {
incrementMultiplicity(t, BigInteger.ONE);
}
}
// Adds all elements found in the list to a new DafnyMultiSet. The number of occurrences in the list becomes the
// multiplicity in the DafnyMultiset
public DafnyMultiset(List l) {
assert l != null : "Precondition Violation";
innerMap = new HashMap<>();
for (T t : l) {
incrementMultiplicity(t, BigInteger.ONE);
}
}
@SafeVarargs
public static DafnyMultiset of(T ... args) {
return new DafnyMultiset(Arrays.asList(args));
}
private static final DafnyMultiset
© 2015 - 2025 Weber Informatics LLC | Privacy Policy