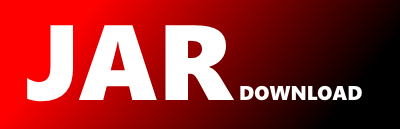
io.bit3.jsass.Options Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of io.bit3.jsass Show documentation
Show all versions of io.bit3.jsass Show documentation
SASS compiler using libsass.
The newest version!
package io.bit3.jsass;
import io.bit3.jsass.importer.Importer;
import java.io.File;
import java.io.Serializable;
import java.net.URI;
import java.util.Collection;
import java.util.LinkedList;
import java.util.List;
/**
* The compiler options.
*/
public class Options implements Serializable {
private static final long serialVersionUID = -3258968135964372076L;
/**
* Custom import functions.
*/
private transient List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy