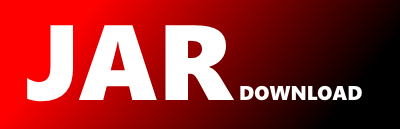
net.sf.saxon.s9api.XsltCompiler Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of saxon-he Show documentation
Show all versions of saxon-he Show documentation
An OSGi bundle for Saxon-HE
Please wait ...
© 2015 - 2025 Weber Informatics LLC | Privacy Policy