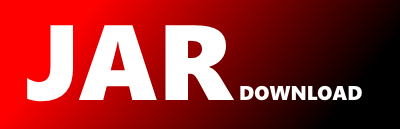
org.daisy.pipeline.client.http.WS Maven / Gradle / Ivy
package org.daisy.pipeline.client.http;
import java.io.BufferedWriter;
import java.io.File;
import java.io.FileWriter;
import java.io.IOException;
import java.io.InputStream;
import java.io.StringWriter;
import java.net.InetAddress;
import java.net.NetworkInterface;
import java.net.SocketException;
import java.net.URI;
import java.net.URISyntaxException;
import java.net.UnknownHostException;
import java.nio.file.Files;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import javax.xml.transform.Transformer;
import javax.xml.transform.TransformerConfigurationException;
import javax.xml.transform.TransformerException;
import javax.xml.transform.TransformerFactory;
import javax.xml.transform.TransformerFactoryConfigurationError;
import javax.xml.transform.dom.DOMSource;
import javax.xml.transform.stream.StreamResult;
import org.daisy.pipeline.client.Pipeline2Exception;
import org.daisy.pipeline.client.Pipeline2Logger;
import org.daisy.pipeline.client.models.*;
import org.daisy.pipeline.client.utils.XPath;
import org.w3c.dom.Document;
/**
* Methods for communicating with the Pipeline 2 API.
*
* @see http://code.google.com/p/daisy-pipeline/wiki/WebServiceAPI
*/
public class WS implements WSInterface {
private String endpoint;
private String username;
private String secret;
private String shutDownKey;
private boolean isLocal;
public WS() {
endpoint = "http://localhost:8181/ws";
username = "clientid";
secret = "supersecret";
try {
List shutDownKeyFileLines = Files.readAllLines(new File(System.getProperty("java.io.tmpdir")+File.separator+"dp2key.txt").toPath());
shutDownKey = shutDownKeyFileLines.get(0);
} catch (IOException e) {/*ignore*/}
checkIfLocal();
}
/** Set which Pipeline 2 Web API endpoint to use. Defaults to: "http://localhost:8181/ws" */
@Override
public void setEndpoint(String endpoint) {
this.endpoint = endpoint;
checkIfLocal();
}
@Override
public String getEndpoint() {
return endpoint;
}
/** Set the credentials to use for the Pipeline 2 Web API. Defaults to "clientid" and "supersecret". */
@Override
public void setCredentials(String username, String secret) {
this.username = username;
this.secret = secret;
}
@Override
public String getUsername() {
return username;
}
/** Set the key used when invoking /admin/halt to shut down the engine.
*
* Defaults to the first line if the "dp2key.txt" file in the systems temporary directory. */
@Override
public void setShutDownKey(String shutDownKey) {
this.shutDownKey = shutDownKey;
}
@Override
public String getShutDownKey() {
return shutDownKey;
}
@Override
public Alive alive() {
try {
WSResponse response = Pipeline2HttpClient.get(endpoint, "/alive", null, null, null);
if (response.status >= 200 && response.status < 300) {
return new Alive(response.asXml());
} else {
error(response);
return null;
}
} catch (Pipeline2Exception e) {
Pipeline2Logger.logger().error("failed to parse /alive response", e);
return null;
}
}
@Override
public boolean halt() {
try {
WSResponse response = Pipeline2HttpClient.get(endpoint, "/admin/halt/"+shutDownKey, username, secret, null);
if (response.status >= 200 && response.status < 300) {
return true;
} else {
error(response);
return false;
}
} catch (Pipeline2Exception e) {
Pipeline2Logger.logger().error("failed to parse /admin/halt/"+shutDownKey+" response", e);
return false;
}
}
@Override
public List getProperties() {
try {
WSResponse response = Pipeline2HttpClient.get(endpoint, "/admin/properties", username, secret, null);
if (response.status >= 200 && response.status < 300) {
return Property.parsePropertiesXml(response.asXml());
} else {
error(response);
return null;
}
} catch (Pipeline2Exception e) {
Pipeline2Logger.logger().error("failed to parse /admin/properties response", e);
return null;
}
}
@Override
public List