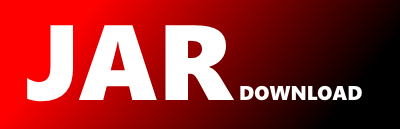
org.daisy.pipeline.client.http.WSInterface Maven / Gradle / Ivy
package org.daisy.pipeline.client.http;
import java.io.File;
import java.io.InputStream;
import java.util.List;
import java.util.Map;
import org.daisy.pipeline.client.models.*;
/**
* Methods for communicating with the Pipeline 2 API.
*
* @see http://code.google.com/p/daisy-pipeline/wiki/WebServiceAPI
*/
public interface WSInterface {
// ---------- Configuration ----------
/** Set which Pipeline 2 Web API endpoint to use. For instance: "http://localhost:8181/ws" */
public void setEndpoint(String endpoint);
/** Return which Pipeline 2 Web API endpoint is currently used */
public String getEndpoint();
/** Set the credentials to use for the Pipeline 2 Web API */
public void setCredentials(String username, String secret);
/** Get the username used to authenticate with the Pipeline 2 Web API */
public String getUsername();
/** Set the key used when invoking /admin/halt to shut down the engine */
public void setShutDownKey(String key);
/** Get the key meant to be used when invoking /admin/halt */
public String getShutDownKey();
// ---------- Engine ----------
/** Get information about the framework */
public Alive alive();
/** Stop the web service */
public boolean halt();
/** Get the properties used in the Pipeline 2 engine */
public List getProperties();
// ---------- Scripts ----------
/** Get a single script */
public Script getScript(String scriptId);
/** Get all scripts */
public List