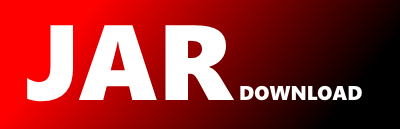
org.daisy.pipeline.client.http.WSInterface Maven / Gradle / Ivy
package org.daisy.pipeline.client.http;
import java.io.File;
import java.io.InputStream;
import java.util.List;
import java.util.Map;
import org.daisy.pipeline.client.models.*;
/**
* Methods for communicating with the Pipeline 2 API.
*
* @see http://code.google.com/p/daisy-pipeline/wiki/WebServiceAPI
*/
public interface WSInterface {
// ---------- Configuration ----------
/**
* Set which Pipeline 2 Web API endpoint to use. For instance: "http://localhost:8181/ws"
*
* @param endpoint the endpoint to use
*/
public void setEndpoint(String endpoint);
/**
* Return which Pipeline 2 Web API endpoint is currently used
*
* @return the endpoint used
*/
public String getEndpoint();
/**
* Set the credentials to use for the Pipeline 2 Web API
*
* @param username the username to use
* @param secret the secret to use
*/
public void setCredentials(String username, String secret);
/**
* Get the username used to authenticate with the Pipeline 2 Web API
*
* @return the username
*/
public String getUsername();
/**
* Set the key used when invoking /admin/halt to shut down the engine
*
* @param key the shutdown key to use
*/
public void setShutDownKey(String key);
/**
* Get the key meant to be used when invoking /admin/halt
*
* @return the shutdown key in use
*/
public String getShutDownKey();
// ---------- Engine ----------
/**
* Get information about the engine
*
* @return information about the engine
*/
public Alive alive();
/**
* Stop the web service
*
* @return whether or not halting the engine succeeded
*/
public boolean halt();
/**
* Get the properties used in the Pipeline 2 engine
*
* @return the list of properties
*/
public List getProperties();
// ---------- Scripts ----------
/**
* Get a single script
*
* @param scriptId the ID of the script
* @return the script
*/
public Script getScript(String scriptId);
/**
* Get all scripts
*
* @return the list of scripts
*/
public List