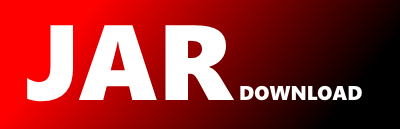
org.darkphoenixs.pool.PoolBase Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of connectionpool-client Show documentation
Show all versions of connectionpool-client Show documentation
A simple multi-purpose connection pool client (Kafka & Hbase & Redis & RMDB & Socket & Http)
The newest version!
/*
* Copyright 2015-2016 Dark Phoenixs (Open-Source Organization).
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.darkphoenixs.pool;
import org.apache.commons.pool2.PooledObjectFactory;
import org.apache.commons.pool2.impl.GenericObjectPool;
import org.apache.commons.pool2.impl.GenericObjectPoolConfig;
import java.io.Closeable;
import java.io.Serializable;
/**
* Title: PoolBase
* Description: 池基类
*
* @author Victor
* @version 1.0
* @see Closeable
* @see Serializable
* @since 2015年9月19日
*/
public abstract class PoolBase implements Closeable, Serializable {
/**
* serialVersionUID
*/
private static final long serialVersionUID = 536428799879058482L;
/**
* internalPool
*/
protected GenericObjectPool internalPool;
/**
* Title: ConnectionPoolBase
* Description: 构造方法
*/
public PoolBase() {
}
/**
* Title: ConnectionPoolBase
* Description: 构造方法
*
* @param poolConfig 池配置
* @param factory 池对象工厂
*/
public PoolBase(final GenericObjectPoolConfig poolConfig,
PooledObjectFactory factory) {
this.initPool(poolConfig, factory);
}
/**
* Title: initPool
* Description: 初始化对象池
*
* @param poolConfig 池配置
* @param factory 池对象工厂
*/
protected void initPool(final GenericObjectPoolConfig poolConfig,
PooledObjectFactory factory) {
if (this.internalPool != null)
this.destroy();
this.internalPool = new GenericObjectPool(factory, poolConfig);
}
/**
* Title: destroy
* Description: 销毁对象池
*/
protected void destroy() {
this.close();
}
/**
* Title: getResource
* Description: 获得池对象
*
* @return 池对象
*/
protected T getResource() {
try {
return internalPool.borrowObject();
} catch (Exception e) {
throw new ConnectionException(
"Could not get a resource from the pool", e);
}
}
/**
* Title: returnResource
* Description: 返回池对象
*
* @param resource 池对象
*/
protected void returnResource(final T resource) {
if (null != resource)
try {
internalPool.returnObject(resource);
} catch (Exception e) {
throw new ConnectionException(
"Could not return the resource to the pool", e);
}
}
/**
* Title: invalidateResource
* Description: 废弃池对象
*
* @param resource 池对象
*/
protected void invalidateResource(final T resource) {
if (null != resource)
try {
internalPool.invalidateObject(resource);
} catch (Exception e) {
throw new ConnectionException(
"Could not invalidate the resource to the pool", e);
}
}
/**
* Title: getNumActive
* Description: 获得池激活数
*
* @return 激活数
*/
public int getNumActive() {
if (isInactived()) {
return -1;
}
return this.internalPool.getNumActive();
}
/**
* Title: getNumIdle
* Description: 获得池空闲数
*
* @return 空闲数
*/
public int getNumIdle() {
if (isInactived()) {
return -1;
}
return this.internalPool.getNumIdle();
}
/**
* Title: getNumWaiters
* Description: 获得池等待数
*
* @return 等待数
*/
public int getNumWaiters() {
if (isInactived()) {
return -1;
}
return this.internalPool.getNumWaiters();
}
/**
* Title: getMeanBorrowWaitTimeMillis
* Description: 获得平均等待时间
*
* @return 平均等待时间
*/
public long getMeanBorrowWaitTimeMillis() {
if (isInactived()) {
return -1;
}
return this.internalPool.getMeanBorrowWaitTimeMillis();
}
/**
* Title: getMaxBorrowWaitTimeMillis
* Description: 获得最大等待时间
*
* @return 最大等待时间
*/
public long getMaxBorrowWaitTimeMillis() {
if (isInactived()) {
return -1;
}
return this.internalPool.getMaxBorrowWaitTimeMillis();
}
/**
* Title: isClosed
* Description: 池是否关闭
*
* @return 是否关闭
*/
public boolean isClosed() {
try {
return this.internalPool.isClosed();
} catch (Exception e) {
throw new ConnectionException(
"Could not check closed from the pool", e);
}
}
/**
* Title: isInactived
* Description: 池是否失效
*
* @return 是否失效
*/
private boolean isInactived() {
try {
return this.internalPool == null || this.internalPool.isClosed();
} catch (Exception e) {
throw new ConnectionException(
"Could not check inactived from the pool", e);
}
}
/**
* Title: addObjects
* Description: 添加池对象
*
* @param count 池对象数量
*/
protected void addObjects(final int count) {
try {
for (int i = 0; i < count; i++) {
this.internalPool.addObject();
}
} catch (Exception e) {
throw new ConnectionException("Error trying to add idle objects", e);
}
}
/**
* Title: clear
* Description: 清除对象池
*/
public void clear() {
try {
this.internalPool.clear();
} catch (Exception e) {
throw new ConnectionException("Could not clear the pool", e);
}
}
/**
* Title: close
* Description: 关闭对象池
*/
public void close() {
try {
this.internalPool.close();
} catch (Exception e) {
throw new ConnectionException("Could not destroy the pool", e);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy