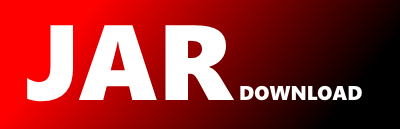
org.darkphoenixs.pool.hbase.HbaseSharedConnPool Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of connectionpool-client Show documentation
Show all versions of connectionpool-client Show documentation
A simple multi-purpose connection pool client (Kafka & Hbase & Redis & RMDB & Socket & Http)
The newest version!
package org.darkphoenixs.pool.hbase;
import org.apache.hadoop.conf.Configuration;
import org.apache.hadoop.hbase.client.Connection;
import org.apache.hadoop.hbase.client.ConnectionFactory;
import org.darkphoenixs.pool.ConnectionException;
import org.darkphoenixs.pool.ConnectionPool;
import java.io.IOException;
import java.util.Map;
import java.util.Properties;
import java.util.concurrent.atomic.AtomicReference;
/**
* Title: HbaseSharedConnPool
* Description: Hbase共享连接池(单例)
*
* @author Victor.Zxy
* @version 1.2.3
* @see ConnectionPool
* @since 2016 /8/25
*/
public class HbaseSharedConnPool implements ConnectionPool {
private static final AtomicReference pool = new AtomicReference();
private final Connection connection;
private HbaseSharedConnPool(Configuration configuration) throws IOException {
this.connection = ConnectionFactory.createConnection(configuration);
}
/**
* Gets instance.
*
* @param host the host
* @param port the port
* @param master the master
* @param rootdir the rootdir
* @return the instance
*/
public synchronized static HbaseSharedConnPool getInstance(final String host, final String port, final String master, final String rootdir) {
Properties properties = new Properties();
if (host == null)
throw new ConnectionException("[" + HbaseConfig.ZOOKEEPER_QUORUM_PROPERTY + "] is required !");
properties.setProperty(HbaseConfig.ZOOKEEPER_QUORUM_PROPERTY, host);
if (port == null)
throw new ConnectionException("[" + HbaseConfig.ZOOKEEPER_CLIENTPORT_PROPERTY + "] is required !");
properties.setProperty(HbaseConfig.ZOOKEEPER_CLIENTPORT_PROPERTY, port);
if (master != null)
properties.setProperty(HbaseConfig.MASTER_PROPERTY, master);
if (rootdir != null)
properties.setProperty(HbaseConfig.ROOTDIR_PROPERTY, rootdir);
return getInstance(properties);
}
/**
* Gets instance.
*
* @param properties the properties
* @return the instance
*/
public synchronized static HbaseSharedConnPool getInstance(final Properties properties) {
Configuration configuration = new Configuration();
for (Map.Entry
© 2015 - 2025 Weber Informatics LLC | Privacy Policy