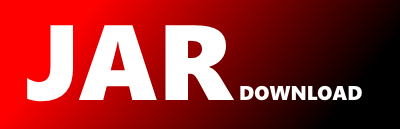
org.databene.model.data.InstanceDescriptor Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of databene-benerator Show documentation
Show all versions of databene-benerator Show documentation
benerator is a framework for creating realistic and valid high-volume test data, used for
testing (unit/integration/load) and showcase setup.
Metadata constraints are imported from systems and/or configuration files. Data can imported from
and exported to files and systems, anonymized or generated from scratch. Domain packages provide
reusable generators for creating domain-specific data as names and addresses internationalizable
in language and region. It is strongly customizable with plugins and configuration options.
The newest version!
/*
* (c) Copyright 2008-2011 by Volker Bergmann. All rights reserved.
*
* Redistribution and use in source and binary forms, with or without
* modification, is permitted under the terms of the
* GNU General Public License.
*
* For redistributing this software or a derivative work under a license other
* than the GPL-compatible Free Software License as defined by the Free
* Software Foundation or approved by OSI, you must first obtain a commercial
* license to this software product from Volker Bergmann.
*
* THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS"
* WITHOUT A WARRANTY OF ANY KIND. ALL EXPRESS OR IMPLIED CONDITIONS,
* REPRESENTATIONS AND WARRANTIES, INCLUDING ANY IMPLIED WARRANTY OF
* MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE OR NON-INFRINGEMENT, ARE
* HEREBY EXCLUDED. IN NO EVENT SHALL THE COPYRIGHT OWNER OR CONTRIBUTORS BE
* LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR
* CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF
* SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS
* INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN
* CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE)
* ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE
* POSSIBILITY OF SUCH DAMAGE.
*/
package org.databene.model.data;
import org.databene.benerator.engine.expression.ScriptExpression;
import org.databene.commons.operation.AndOperation;
import org.databene.commons.operation.OrOperation;
import org.databene.script.Expression;
import org.databene.script.expression.ConstantExpression;
import org.databene.script.expression.TypeConvertingExpression;
/**
* Describes generation of (several) entities of a type by uniqueness,
* nullability and count characteristics.
* Created: 03.03.2008 07:55:45
* @since 0.5.0
* @author Volker Bergmann
*/
public class InstanceDescriptor extends FeatureDescriptor {
public static final String TYPE = "type";
// constraints
public static final String UNIQUE = "unique";
public static final String NULLABLE = "nullable";
public static final String MIN_COUNT = "minCount";
public static final String MAX_COUNT = "maxCount";
public static final String CONTAINER = "container";
// configs
public static final String COUNT_GRANULARITY = "countGranularity";
public static final String COUNT_DISTRIBUTION = "countDistribution";
public static final String COUNT = "count";
public static final String NULL_QUOTA = "nullQuota";
public static final String MODE = "mode";
private InstanceDescriptor parent;
private TypeDescriptor localType;
// constructors ----------------------------------------------------------------------------------------------------
public InstanceDescriptor(String name, DescriptorProvider provider) {
this(name, provider, null, null);
}
public InstanceDescriptor(String name, DescriptorProvider provider, String typeName) {
this(name, provider, typeName, null);
}
public InstanceDescriptor(String name, DescriptorProvider provider, TypeDescriptor localType) {
this(name, provider, null, localType);
}
protected InstanceDescriptor(String name, DescriptorProvider provider, String typeName, TypeDescriptor localType) {
super(name, provider);
this.localType = localType;
addConstraint(TYPE, String.class, null);
setType(typeName);
// constraints
addConstraint(UNIQUE, Boolean.class, new OrOperation());
addConstraint(NULLABLE, Boolean.class, new AndOperation());
addConstraint(MIN_COUNT, Expression.class, null);
addConstraint(MAX_COUNT, Expression.class, null);
addConstraint(CONTAINER, String.class, null);
// configs
addConfig(COUNT, Expression.class);
addConfig(COUNT_GRANULARITY, Expression.class);
addConfig(COUNT_DISTRIBUTION, String.class);
addConfig(NULL_QUOTA, Double.class);
addConfig(MODE, Mode.class);
}
// properties ------------------------------------------------------------------------------------------------------
public void setParent(InstanceDescriptor parent) {
this.parent = parent;
}
@Override
public String getName() {
String result = super.getName();
return (result != null ? result : getType());
}
public String getType() {
String type = (String) getDetailValue(TYPE);
return (type == null && parent != null ? parent.getType() : type);
}
public void setType(String type) {
setDetailValue(TYPE, type);
}
public TypeDescriptor getTypeDescriptor() {
if (getLocalType() != null)
return getLocalType();
TypeDescriptor type = null;
if (getType() != null)
type = getDataModel().getTypeDescriptor(getType());
return type;
}
public TypeDescriptor getLocalType() {
if (localType == null && parent != null && parent.getLocalType() != null)
localType = getLocalType(parent.getLocalType() instanceof ComplexTypeDescriptor);
return localType;
}
public TypeDescriptor getLocalType(boolean complexType) {
if (localType != null)
return localType;
if (complexType)
localType = new ComplexTypeDescriptor(getName(), provider, getType());
else
localType = new SimpleTypeDescriptor(getName(), provider, getType());
setType(null);
return localType;
}
public void setLocalType(TypeDescriptor localType) {
this.localType = localType;
if (localType != null)
setType(null);
}
public Boolean isUnique() {
return (Boolean) getDetailValue(UNIQUE);
}
public void setUnique(Boolean unique) {
setDetailValue(UNIQUE, unique);
}
public Uniqueness getUniqueness() {
Boolean unique = isUnique();
return (unique != null ? (unique ? Uniqueness.SIMPLE : Uniqueness.NONE) : null);
}
public Boolean isNullable() {
Boolean value = (Boolean) super.getDetailValue(NULLABLE);
if (value == null && parent != null) {
FeatureDetail> detail = parent.getConfiguredDetail(NULLABLE);
if (detail.getValue() != null && !(Boolean) detail.getValue())
value = (Boolean) detail.getValue();
}
return value;
}
public void setNullable(Boolean nullable) {
setDetailValue(NULLABLE, nullable);
}
@SuppressWarnings("unchecked")
public Expression getMinCount() {
return (Expression) getDetailValue(MIN_COUNT);
}
public void setMinCount(Expression minCount) {
setDetailValue(MIN_COUNT, minCount);
}
@SuppressWarnings("unchecked")
public Expression getMaxCount() {
return (Expression) getDetailValue(MAX_COUNT);
}
public void setMaxCount(Expression maxCount) {
setDetailValue(MAX_COUNT, maxCount);
}
public String getContainer() {
return (String) getDetailValue(CONTAINER);
}
public void setContainer(String container) {
setDetailValue(CONTAINER, container);
}
@SuppressWarnings("unchecked")
public Expression getCount() {
return (Expression) getDetailValue(COUNT);
}
public void setCount(Expression count) {
setDetailValue(COUNT, count);
}
public String getCountDistribution() {
return (String) getDetailValue(COUNT_DISTRIBUTION);
}
public void setCountDistribution(String distribution) {
setDetailValue(COUNT_DISTRIBUTION, distribution);
}
@SuppressWarnings("unchecked")
public Expression getCountGranularity() {
return (Expression) getDetailValue(COUNT_GRANULARITY);
}
public void setCountGranularity(Expression distribution) {
setDetailValue(COUNT_GRANULARITY, distribution);
}
public Double getNullQuota() {
return (Double)getDetailValue(NULL_QUOTA);
}
public void setNullQuota(Double nullQuota) {
setDetailValue(NULL_QUOTA, nullQuota);
}
public Mode getMode() {
return (Mode) getDetailValue(MODE);
}
public void setMode(Mode mode) {
setDetailValue(MODE, mode);
}
@Override
public Object getDetailValue(String name) {
Object value = super.getDetailValue(name);
if (value == null && parent != null && parent.supportsDetail(name)) {
FeatureDetail> detail = parent.getConfiguredDetail(name);
if (detail.isConstraint())
value = detail.getValue();
}
return value;
}
@Override
public void setDetailValue(String detailName, Object detailValue) {
if (COUNT.equals(detailName) || MIN_COUNT.equals(detailName) || MAX_COUNT.equals(detailName) || COUNT_GRANULARITY.equals(detailName)) {
FeatureDetail
© 2015 - 2025 Weber Informatics LLC | Privacy Policy