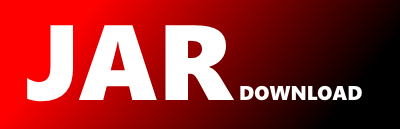
org.databene.platform.fixedwidth.FixedWidthEntitySource Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of databene-benerator Show documentation
Show all versions of databene-benerator Show documentation
benerator is a framework for creating realistic and valid high-volume test data, used for
testing (unit/integration/load) and showcase setup.
Metadata constraints are imported from systems and/or configuration files. Data can imported from
and exported to files and systems, anonymized or generated from scratch. Domain packages provide
reusable generators for creating domain-specific data as names and addresses internationalizable
in language and region. It is strongly customizable with plugins and configuration options.
The newest version!
/*
* (c) Copyright 2008-2014 by Volker Bergmann. All rights reserved.
*
* Redistribution and use in source and binary forms, with or without
* modification, is permitted under the terms of the
* GNU General Public License.
*
* For redistributing this software or a derivative work under a license other
* than the GPL-compatible Free Software License as defined by the Free
* Software Foundation or approved by OSI, you must first obtain a commercial
* license to this software product from Volker Bergmann.
*
* THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS"
* WITHOUT A WARRANTY OF ANY KIND. ALL EXPRESS OR IMPLIED CONDITIONS,
* REPRESENTATIONS AND WARRANTIES, INCLUDING ANY IMPLIED WARRANTY OF
* MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE OR NON-INFRINGEMENT, ARE
* HEREBY EXCLUDED. IN NO EVENT SHALL THE COPYRIGHT OWNER OR CONTRIBUTORS BE
* LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR
* CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF
* SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS
* INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN
* CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE)
* ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE
* POSSIBILITY OF SUCH DAMAGE.
*/
package org.databene.platform.fixedwidth;
import java.text.ParseException;
import java.util.Locale;
import org.databene.benerator.InvalidGeneratorSetupException;
import org.databene.commons.ArrayUtil;
import org.databene.commons.Converter;
import org.databene.commons.Escalator;
import org.databene.commons.LoggerEscalator;
import org.databene.commons.SystemInfo;
import org.databene.commons.bean.ArrayPropertyExtractor;
import org.databene.commons.converter.ArrayConverter;
import org.databene.commons.converter.ConverterChain;
import org.databene.commons.converter.NoOpConverter;
import org.databene.commons.format.PadFormat;
import org.databene.formats.DataIterator;
import org.databene.formats.DataSource;
import org.databene.formats.fixedwidth.FixedWidthColumnDescriptor;
import org.databene.formats.fixedwidth.FixedWidthLineSource;
import org.databene.formats.fixedwidth.FixedWidthRowTypeDescriptor;
import org.databene.formats.fixedwidth.FixedWidthUtil;
import org.databene.formats.util.ConvertingDataIterator;
import org.databene.model.data.ComplexTypeDescriptor;
import org.databene.model.data.Entity;
import org.databene.model.data.FileBasedEntitySource;
import org.databene.platform.array.Array2EntityConverter;
/**
* Reads Entities from a fixed-width file.
*
* Created at 07.11.2008 18:18:24
* @since 0.5.6
* @author Volker Bergmann
*/
public class FixedWidthEntitySource extends FileBasedEntitySource {
private static final Escalator escalator = new LoggerEscalator();
private Locale locale;
private String encoding;
private String entityTypeName;
private ComplexTypeDescriptor entityDescriptor;
private FixedWidthColumnDescriptor[] descriptors;
private String lineFilter;
private boolean initialized;
private Converter preprocessor;
protected DataSource source;
protected Converter converter;
public FixedWidthEntitySource() {
this(null, null, SystemInfo.getFileEncoding(), null);
}
public FixedWidthEntitySource(String uri, ComplexTypeDescriptor entityDescriptor,
String encoding, String lineFilter, FixedWidthColumnDescriptor ... descriptors) {
this(uri, entityDescriptor, new NoOpConverter(), encoding, lineFilter, descriptors);
}
public FixedWidthEntitySource(String uri, ComplexTypeDescriptor entityDescriptor,
Converter preprocessor, String encoding, String lineFilter,
FixedWidthColumnDescriptor ... descriptors) {
super(uri);
this.locale = Locale.getDefault();
this.encoding = encoding;
this.entityDescriptor = entityDescriptor;
this.entityTypeName = (entityDescriptor != null ? entityDescriptor.getName() : null);
this.descriptors = descriptors;
this.preprocessor = preprocessor;
this.initialized = false;
this.lineFilter = lineFilter;
}
// properties ------------------------------------------------------------------------------------------------------
public void setLocale(Locale locale) {
this.locale = locale;
}
public void setEncoding(String encoding) {
this.encoding = encoding;
}
public String getEntity() {
return entityTypeName;
}
public void setEntity(String entity) {
this.entityTypeName = entity;
}
/**
* @throws ParseException
* @deprecated use {@link #setColumns(String)}
*/
@Deprecated
public void setProperties(String properties) throws ParseException {
escalator.escalate("The property 'properties' of class " + getClass() + "' has been renamed to 'columns'. " +
"Please fix the property name in your configuration", this.getClass(), "setProperties()");
setColumns(properties);
}
public void setColumns(String columns) throws ParseException {
FixedWidthRowTypeDescriptor rowTypeDescriptor = FixedWidthUtil.parseBeanColumnsSpec(
columns, entityTypeName, null, this.locale);
this.descriptors = rowTypeDescriptor.getColumnDescriptors();
}
// Iterable interface ----------------------------------------------------------------------------------------------
public void setLineFilter(String lineFilter) {
this.lineFilter = lineFilter;
}
@Override
public Class getType() {
if (!initialized)
init();
return Entity.class;
}
@Override
public DataIterator iterator() {
if (!initialized)
init();
return new ConvertingDataIterator(this.source.iterator(), converter);
}
// private helpers -------------------------------------------------------------------------------------------------
private void init() {
if (this.entityDescriptor == null)
this.entityDescriptor = new ComplexTypeDescriptor(entityTypeName, context.getLocalDescriptorProvider());
if (ArrayUtil.isEmpty(descriptors))
throw new InvalidGeneratorSetupException("Missing column descriptors. " +
"Use the 'columns' property of the " + getClass().getSimpleName() + " to define them.");
this.source = createSource();
this.converter = createConverter();
}
private DataSource createSource() {
PadFormat[] formats = ArrayPropertyExtractor.convert(descriptors, "format", PadFormat.class);
return new FixedWidthLineSource(resolveUri(), formats, true, encoding, lineFilter);
}
@SuppressWarnings("unchecked")
private Converter createConverter() {
String[] featureNames = ArrayPropertyExtractor.convert(descriptors, "name", String.class);
Array2EntityConverter a2eConverter = new Array2EntityConverter(entityDescriptor, featureNames, true);
Converter aConv = new ArrayConverter(String.class, String.class, preprocessor);
Converter converter = new ConverterChain(aConv, a2eConverter);
return converter;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy