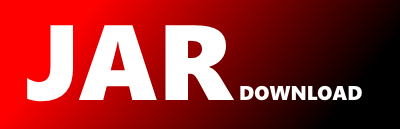
org.databene.commons.converter.AnyConverter Maven / Gradle / Ivy
/*
* Copyright (C) 2004-2014 Volker Bergmann ([email protected]).
* All rights reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
* http://www.apache.org/licenses/LICENSE-2.0
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.databene.commons.converter;
import org.databene.commons.ConversionException;
import org.databene.commons.Converter;
import org.databene.commons.Patterns;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
/**
* Converts any source type to any target type. It also makes use of the ConverterManager.
* Created: 16.06.2007 11:34:42
* @author Volker Bergmann
*/
public class AnyConverter extends FormatHolder implements Converter
© 2015 - 2025 Weber Informatics LLC | Privacy Policy