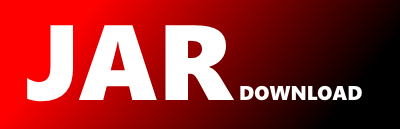
org.databene.edifatto.EdifattoConfig Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of edifatto Show documentation
Show all versions of edifatto Show documentation
'Edifatto' is an open source software library for parsing,
generating and verifying EDIFACT and X12 documents written by Volker Bergmann.
/*
* Copyright (C) 2013-2014 Volker Bergmann ([email protected]).
* All rights reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
* http://www.apache.org/licenses/LICENSE-2.0
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.databene.edifatto;
import java.io.IOException;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import org.databene.commons.Assert;
import org.databene.commons.BeanUtil;
import org.databene.commons.ConfigurationError;
import org.databene.commons.Encodings;
import org.databene.commons.IOUtil;
import org.databene.commons.NullSafeComparator;
import org.databene.commons.ObjectNotFoundException;
import org.databene.commons.StringUtil;
import org.databene.edifatto.definition.MessageDefinition;
import org.databene.edifatto.gui.EdifattoMainPane;
import org.databene.edifatto.gui.browse.InterchangeBrowserPane;
import org.databene.edifatto.gui.compare.DiffPane;
import org.databene.edifatto.gui.def.EdiDefBrowserPane;
import org.databene.edifatto.gui.mapping.MappingPane;
import org.databene.edifatto.gui.param.ParameterizationPane;
import org.databene.edifatto.gui.template.TemplateMainPane;
import org.databene.edifatto.model.EdiProtocol;
import org.databene.edifatto.parser.EdiXsdParser;
/**
* Provides the Edifatto configuration.
* Created: 17.10.2013 10:15:25
* @since 1.0
* @author Volker Bergmann
*/
public class EdifattoConfig {
// public constants for configuration ------------------------------------------------------------------------------
private static final String CONFIG_FILE_NAME = "edifatto.properties";
public static final String DEFAULT_DEFINITION_FOLDER = "org/databene/edifatto";
public static final String EDIFACT_DEFFILENAME_PROPERTY = "edifatto.edifact.deffile";
public static final String DEFAULT_EDIFACT_FILENAME_PATTERN = "${version}${release}_${type}.xsd";
public static final String X12_DEFFILENAME_PROPERTY = "edifatto.x12.deffile";
public static final String DEFAULT_X12_FILENAME_PATTERN = "X12-${version}_${type}.xsd";
// singleton members -----------------------------------------------------------------------------------------------
private static final EdifattoConfig INSTANCE = new EdifattoConfig();
public static EdifattoConfig getInstance() {
return INSTANCE;
}
private String edifactFileNamePattern;
private String x12FileNamePattern;
private String dataClassTemplate;
private String templateTargetFolder;
private String dataClassPackage;
private String dataClassParent;
private int javaTabSize;
private boolean experimentalFeatures;
private List definitionFolders;
private Map definitions;
private String edifattoHome;
// constructor -----------------------------------------------------------------------------------------------------
private EdifattoConfig() {
try {
// set defaults
this.edifactFileNamePattern = DEFAULT_EDIFACT_FILENAME_PATTERN;
this.x12FileNamePattern = DEFAULT_X12_FILENAME_PATTERN;
this.templateTargetFolder = "./generated";
this.dataClassPackage = "com.my.edidata";
this.dataClassTemplate = "org/databene/edifatto/template/javaclass.ftl";
this.dataClassParent = null;
this.javaTabSize = -1;
this.experimentalFeatures = false;
this.definitionFolders = new ArrayList();
this.definitionFolders.add(DEFAULT_DEFINITION_FOLDER);
this.edifattoHome = System.getenv("EDIFATTO_HOME");
if (edifattoHome != null)
this.definitionFolders.add(this.edifattoHome + "/definitions");
this.definitionFolders.add("src/main/definitions");
this.definitionFolders.add("");
this.definitions = new HashMap();
// read config file if exists
if (IOUtil.isURIAvailable(CONFIG_FILE_NAME)) {
Map props = IOUtil.readProperties(CONFIG_FILE_NAME, Encodings.UTF_8);
BeanUtil.setPropertyValues(this, props);
}
// override config file settings with environment settings...
// ...edifactDefpath
String setting = System.getProperty(EDIFACT_DEFFILENAME_PROPERTY);
if (!StringUtil.isEmpty(setting))
this.edifactFileNamePattern = setting;
// ...x12Defpath
setting = System.getProperty(X12_DEFFILENAME_PROPERTY);
if (!StringUtil.isEmpty(setting))
this.x12FileNamePattern = setting;
} catch (IOException e) {
throw new ConfigurationError("Invalid configuration. ", e);
}
}
// interface -------------------------------------------------------------------------------------------------------
public String getDataClassTemplate() {
return dataClassTemplate;
}
public void setDataClassTemplate(String dataClassTemplate) {
this.dataClassTemplate = dataClassTemplate;
}
public String getTemplateTargetFolder() {
return templateTargetFolder;
}
public void setTemplateTargetFolder(String templateTargetFolder) {
this.templateTargetFolder = templateTargetFolder;
}
public String getDataClassPackage() {
return dataClassPackage;
}
public void setDataClassPackage(String dataClassPackage) {
this.dataClassPackage = dataClassPackage;
}
public String getDataClassParent() {
return dataClassParent;
}
public void setDataClassParent(String dataClassParent) {
this.dataClassParent = dataClassParent;
}
public int getJavaTabSize() {
return javaTabSize;
}
public void setJavaTabSize(int javaTabSize) {
this.javaTabSize = javaTabSize;
}
public boolean isExperimentalFeatures() {
return experimentalFeatures;
}
public void setExperimentalFeatures(boolean experimentalFeatures) {
this.experimentalFeatures = experimentalFeatures;
}
public MessageDefinition getMessageDefinition(EdiProtocol protocol, String type, String version, String release) {
EdiDefId defId = new EdiDefId(EdiProtocol.EDIFACT, type, version, release);
MessageDefinition definition = this.definitions.get(defId);
if (definition != null)
return definition;
try {
String definitionFileName = definitionFileName(protocol, type, version, release);
for (String definitionFolder : definitionFolders) {
String defPath = (!StringUtil.isEmpty(definitionFolder) ? definitionFolder + '/' : "") + definitionFileName;
if (IOUtil.isURIAvailable(defPath)) {
definition = EdiXsdParser.parseSchema(defPath, protocol, type, version, release);
this.definitions.put(defId, definition);
return definition;
}
}
throw new ObjectNotFoundException("No message definition found for " + protocol + ":" + type + ":" + version + ":" + release);
} catch (IOException e) {
throw new RuntimeException("Error reading message definition", e);
}
}
public void overrideDefinition(EdiProtocol protocol, String type, String version, String release, String definitionPath) throws IOException {
MessageDefinition definition = EdiXsdParser.parseSchema(definitionPath, protocol, type, version, release);
this.definitions.put(new EdiDefId(protocol, type, version, release), definition);
}
public void resetDefinition(EdiProtocol protocol, String type, String version, String release) {
this.definitions.remove(new EdiDefId(protocol, type, version, release));
}
// private helpers -------------------------------------------------------------------------------------------------
private String definitionFileName(EdiProtocol protocol, String type, String version, String release) {
switch (protocol) {
case EDIFACT : return edifactFileNamePattern.replace("${type}", type).replace("${version}", version).replace("${release}", release);
case X12: return x12FileNamePattern.replace("${type}", type).replace("${version}", version);
default: throw new UnsupportedOperationException("Not a supported protocol: " + protocol);
}
}
private static final class EdiDefId {
public final EdiProtocol protocol;
public final String type;
public final String version;
public final String release;
public EdiDefId(EdiProtocol protocol, String type, String version, String release) {
Assert.notNull(protocol, "protocol");
this.protocol = protocol;
Assert.notNull(type, "type");
this.type = type;
Assert.notNull(version, "version");
this.version = version;
this.release = release;
}
@Override
public int hashCode() {
final int prime = 31;
int result = 1;
result = prime * result + ((protocol == null) ? 0 : protocol.hashCode());
result = prime * result + ((release == null) ? 0 : release.hashCode());
result = prime * result + ((type == null) ? 0 : type.hashCode());
result = prime * result + ((version == null) ? 0 : version.hashCode());
return result;
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null || getClass() != obj.getClass())
return false;
EdiDefId that = (EdiDefId) obj;
return (this.protocol == that.protocol &&
this.type.equals(that.type) &&
this.version.equals(that.version) &&
NullSafeComparator.equals(this.release, that.release)
);
}
}
public List getGUIPanes() {
List panes = new ArrayList();
panes.add(new InterchangeBrowserPane());
panes.add(new EdiDefBrowserPane());
panes.add(new DiffPane());
if (isExperimentalFeatures()) {
panes.add(new ParameterizationPane());
panes.add(new MappingPane());
panes.add(new TemplateMainPane());
}
return panes;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy