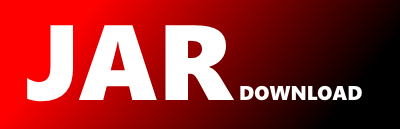
org.databene.edifatto.gui.compare.DiffView Maven / Gradle / Ivy
/*
* Copyright (C) 2013-2015 Volker Bergmann ([email protected]).
* All rights reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
* http://www.apache.org/licenses/LICENSE-2.0
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.databene.edifatto.gui.compare;
import java.awt.BorderLayout;
import javax.swing.JEditorPane;
import javax.swing.JFrame;
import javax.swing.JPanel;
import javax.swing.JScrollPane;
import javax.swing.SwingUtilities;
import javax.swing.text.html.HTMLEditorKit;
import org.databene.commons.ui.swing.SwingUtil;
import org.databene.edifatto.EdiChecker;
import org.databene.edifatto.Edifatto;
import org.databene.edifatto.compare.HTMLDiffFormatter;
import org.databene.edifatto.model.Interchange;
import org.databene.edifatto.xml.NameBasedEdiToXMLConverter;
import org.databene.formats.compare.AggregateDiff;
import org.databene.formats.xml.compare.DefaultXMLComparisonModel;
import org.databene.formats.xml.compare.XMLComparisonSettings;
/**
* Displays the diff between two Edifact documents.
* Created: 14.06.2014 07:16:08
* @since 1.2.1
* @author Volker Bergmann
*/
public class DiffView extends JPanel {
private static final long serialVersionUID = 1L;
private static final String CSS_PATH = "org/databene/edifatto/edifatto-gui.css";
private JEditorPane htmlPane;
private JScrollPane scroller;
public DiffView() {
super(new BorderLayout());
this.htmlPane = createHTMLPane();
this.scroller = new JScrollPane(htmlPane);
add(scroller, BorderLayout.CENTER);
}
public void setDiff(AggregateDiff diff) {
String html = new HTMLDiffFormatter(CSS_PATH).formatDiffAsHtml(diff);
this.htmlPane.setText(html);
// scroll to top of pane
SwingUtilities.invokeLater(new Runnable() {
@Override
public void run() {
scroller.getVerticalScrollBar().setValue(0);
}
});
}
// private helper methods ------------------------------------------------------------------------------------------
private JEditorPane createHTMLPane() {
JEditorPane pane = new JEditorPane();
pane.setEditorKit(new HTMLEditorKit());
pane.setEditable(false);
putClientProperty(JEditorPane.HONOR_DISPLAY_PROPERTIES, Boolean.TRUE);
return pane;
}
public static void main(String[] args) throws Exception {
JFrame frame = new JFrame();
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
Interchange ic1 = Edifatto.parseEdiFile("IFTDGN_1.edi");
//Interchange ic2 = Edifatto.parseEdiFile("IFTDGN_1_reordered_CNI.edi");
Interchange ic2 = Edifatto.parseEdiFile("IFTDGN_2.edi");
DefaultXMLComparisonModel model = new DefaultXMLComparisonModel();
model.addKeyExpression("L-CNI_25", ".//E-1004_25_02.01");
XMLComparisonSettings settings = new XMLComparisonSettings(model);
NameBasedEdiToXMLConverter converter = new NameBasedEdiToXMLConverter();
EdiChecker checker = new EdiChecker(settings, converter);
AggregateDiff diffs = checker.diff(ic1, ic2);
DiffView diffPane = new DiffView();
diffPane.setDiff(diffs);
frame.getContentPane().add(diffPane, BorderLayout.CENTER);
frame.setSize(800, 600);
SwingUtil.center(frame);
frame.setVisible(true);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy