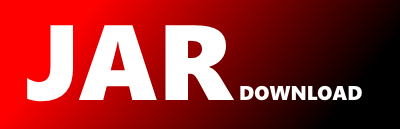
org.databene.edifatto.template.FtlTemplateWriter Maven / Gradle / Ivy
/*
* Copyright (C) 2013-2015 Volker Bergmann ([email protected]).
* All rights reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
* http://www.apache.org/licenses/LICENSE-2.0
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.databene.edifatto.template;
import java.io.IOException;
import java.io.OutputStream;
import java.nio.charset.Charset;
import java.util.ArrayDeque;
import java.util.Deque;
import org.databene.commons.Assert;
import org.databene.commons.Encodings;
import org.databene.commons.ProgrammerError;
import org.databene.edifatto.EdiFormatSymbols;
import org.databene.edifatto.SegmentFormatter;
import org.databene.edifatto.gui.ParameterizationHelper;
import org.databene.edifatto.model.Component;
import org.databene.edifatto.model.Composite;
import org.databene.edifatto.model.EdiGroup;
import org.databene.edifatto.model.EdiItem;
import org.databene.edifatto.model.Interchange;
import org.databene.edifatto.model.Segment;
import org.databene.edifatto.model.SegmentItem;
import org.databene.edifatto.util.Parent;
/**
* Creates FTL templates from an annotated {@link Interchange}.
* Created: 23.07.2014 18:02:34
* @since 2.0.0
* @author Volker Bergmann
*/
public class FtlTemplateWriter {
private Charset charset;
private String linefeed;
public FtlTemplateWriter() {
this(Encodings.ASCII, "\n");
}
public FtlTemplateWriter(String encoding, String linefeed) {
this.charset = Charset.forName(encoding);
this.linefeed = linefeed;
}
public void write(Interchange interchange, OutputStream out) throws IOException {
Context context = new Context(out, interchange.getSymbols());
context.pushPathNode(ParameterizationHelper.getItemName(interchange));
context.writeTextLine("<#escape x as ediEscape(x)>");
writeParent(interchange, context);
context.writeText("#escape>");
}
@SuppressWarnings({ "unchecked", "rawtypes" })
private void writeParent(Parent extends EdiItem> parent, Context context) throws IOException {
EdiItem parentEdiItem = (EdiItem) parent;
String parameterName = ParameterizationHelper.getPropertyName(parentEdiItem);
boolean isList = (parameterName != null && (parentEdiItem instanceof EdiGroup));
String itemName = null;
if (isList) {
itemName = ParameterizationHelper.getItemName(parentEdiItem);
context.writeTextLine("<#list " + context.peekNode() + '.' + parameterName + " as " + itemName + ">");
context.pushPathNode(itemName);
}
for (int i = 0; i < parent.getChildCount(); i++) {
EdiItem child = parent.getChild(i);
if (child instanceof Segment)
writeSegment((Segment) child, context);
else if (child instanceof Parent)
writeParent((Parent) child, context);
else
throw new ProgrammerError("Unexpected element type: " + parent.getClass().getName());
}
if (isList) {
context.popPathNode(itemName);
context.writeTextLine("#list>");
}
}
private static void writeSegment(Segment segment, Context context) throws IOException {
if (segment.getTag().equals("UNA")) {
context.writeUNASegment(segment);
} else {
context.writeText(segment.getTag() + segment.getElementSeparator());
for (int i = 0; i < segment.getChildCount(); i++) {
SegmentItem child = segment.getChild(i);
if (i > 0)
context.writeElementSeparator();
if (child instanceof Composite)
writeComposite((Composite) child, context);
else
writeComponent((Component) child, i, context);
}
context.writeTextLine(String.valueOf(segment.getSegmentSeparator()));
}
}
private static void writeComposite(Composite composite, Context context) throws IOException {
for (int i = 0; i < composite.getChildCount(); i++) {
if (i > 0)
context.writeComponentSeparator();
writeComponent(composite.getChild(i), i, context);
}
}
private static void writeComponent(Component component, int index, Context context) throws IOException {
String text;
if (component.getSegment().getTag().equals("UNT") && index == 0) {
text = "${" + context.peekNode() + '.' + "recursiveSegmentCount}";
} else {
String parameterName = ParameterizationHelper.getPropertyName(component);
if (parameterName != null)
text = "${" + context.peekNode() + '.' + parameterName + "}";
else
text = component.getData();
}
context.writeText(text);
}
private class Context {
OutputStream out;
private EdiFormatSymbols symbols;
Deque path;
Context(OutputStream out, EdiFormatSymbols symbols) {
this.out = out;
this.symbols = symbols;
this.path = new ArrayDeque();
}
public void writeComponentSeparator() throws IOException {
writeText(String.valueOf(symbols.componentSeparator));
}
public void writeElementSeparator() throws IOException {
writeText(String.valueOf(symbols.elementSeparator));
}
public void writeUNASegment(Segment segment) throws IOException {
writeTextLine(SegmentFormatter.formatUNASegment(segment));
}
void pushPathNode(String token) {
this.path.push(token);
}
void popPathNode(String token) {
String tmp = this.path.pop();
Assert.equals(tmp, token, "Inconsistent state, exptected '" + tmp + "' but found + '" + token + "'");
}
String peekNode() {
return this.path.peek();
}
void writeTextLine(String text) throws IOException {
writeText(text);
writeText(linefeed);
}
void writeText(String text) throws IOException {
out.write(text.getBytes(charset));
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy