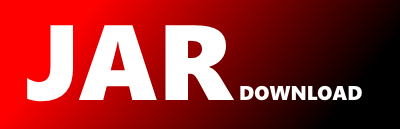
org.datanucleus.store.rdbms.key.ColumnOrderedKey Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of datanucleus-rdbms Show documentation
Show all versions of datanucleus-rdbms Show documentation
Plugin for DataNucleus providing persistence to RDBMS datastores.
/**********************************************************************
Copyright (c) 2018 Andy Jefferson and others. All rights reserved.
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
Contributors:
...
**********************************************************************/
package org.datanucleus.store.rdbms.key;
import java.util.ArrayList;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import java.util.StringTokenizer;
import org.datanucleus.exceptions.NucleusException;
import org.datanucleus.store.rdbms.table.Column;
import org.datanucleus.store.rdbms.table.Table;
import org.datanucleus.util.NucleusLogger;
import org.datanucleus.util.StringUtils;
/**
* Representation of a key that has columns with specified ordering (ascending/descending) for each column (if required).
*/
public abstract class ColumnOrderedKey extends Key
{
/** Column ordering. True implies ascending order. */
protected List columnOrdering = new ArrayList<>();
protected Map extensions = null;
public ColumnOrderedKey(Table table, Map extensions)
{
super(table);
this.extensions = extensions;
}
public String getValueForExtension(String key)
{
return extensions != null ? extensions.get(key) : null;
}
/**
* Class to add a column to the key
* @param col The column to add
*/
public void addColumn(Column col)
{
assertSameDatastoreObject(col);
columns.add(col);
columnOrdering.add(null);
}
public void setColumnOrdering(String ordering)
{
if (StringUtils.isWhitespace(ordering))
{
return;
}
StringTokenizer tokeniser = new StringTokenizer(ordering, ",");
if (tokeniser.countTokens() != columns.size())
{
NucleusLogger.DATASTORE_SCHEMA.warn("Attempt to specify orderings of index with name=" + name +
" but incorrect number of orderings (" + tokeniser.countTokens() + ") for columns (" +columns.size() + "). IGNORED");
return;
}
Iterator colIter = columns.iterator();
int i = 0;
while (tokeniser.hasMoreTokens())
{
String orderingToken = tokeniser.nextToken();
colIter.next();
columnOrdering.set(i, orderingToken.equalsIgnoreCase("ASC") ? Boolean.TRUE : orderingToken.equalsIgnoreCase("DESC") ? Boolean.FALSE : null);
i++;
}
}
/**
* Sets a column in a specified position seq
for this index.
* @param seq the specified position for the col
* @param col the Column
*/
public void setColumn(int seq, Column col)
{
assertSameDatastoreObject(col);
setMinSize(columns, seq + 1);
setMinSize(columnOrdering, seq + 1);
if (columns.get(seq) != null)
{
throw new NucleusException("Index/candidate part #" + seq + " for " + table + " already set").setFatal();
}
columns.set(seq, col);
columnOrdering.set(seq, null);
}
/**
* Method to return the list of columns which the key applies to.
* @param includeOrdering Whether to include ordering in the column list when it is specified
* @return The column list.
*/
public String getColumnList(boolean includeOrdering)
{
StringBuilder s = new StringBuilder("(");
Iterator colIter = columns.iterator();
Iterator colOrderIter = columnOrdering.iterator();
while (colIter.hasNext())
{
Column col = colIter.next();
s.append(col != null ? col.getIdentifier() : "?");
if (includeOrdering)
{
Boolean colOrder = colOrderIter.next();
if (colOrder != null)
{
s.append(colOrder ? " ASC" : " DESC");
}
}
if (colIter.hasNext())
{
s.append(',');
}
}
s.append(')');
return s.toString();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy