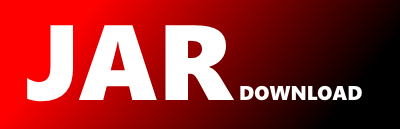
org.dspace.autoversioning.AutoVersioningConsumer Maven / Gradle / Ivy
/**
* The contents of this file are subject to the license and copyright
* detailed in the LICENSE and NOTICE files at the root of the source
* tree and available online at
*
* http://www.dspace.org/license/
*/
package org.dspace.autoversioning;
import com.google.common.base.Throwables;
import org.apache.commons.collections.map.MultiValueMap;
import org.apache.log4j.Logger;
import org.dspace.content.Bitstream;
import org.dspace.content.DSpaceObject;
import org.dspace.content.Item;
import org.dspace.core.Constants;
import org.dspace.core.Context;
import org.dspace.event.Consumer;
import org.dspace.event.Event;
import org.dspace.utils.DSpace;
import org.dspace.versioning.VersioningService;
import java.util.Collection;
import java.util.Date;
/**
*
*
* @author Fabio Bolognesi (fabio at atmire dot com)
* @author Mark Diggory (markd at atmire dot com)
* @author Ben Bosman (ben at atmire dot com)
*/
public class AutoVersioningConsumer implements Consumer {
/** log4j category */
private static Logger log = Logger.getLogger(AutoVersioningConsumer.class);
private MultiValueMap itemsToProcess;
public void initialize() throws Exception {}
public void finish(Context ctx) throws Exception {}
public void consume(Context ctx, Event event) throws Exception {
if(itemsToProcess == null){
itemsToProcess = new MultiValueMap();
}
int st = event.getSubjectType();
int et = event.getEventType();
if(st == Constants.ITEM && (et == Event.INSTALL||et == Event.MODIFY||et == Event.MODIFY_METADATA)){
Item item = (Item) event.getSubject(ctx);
itemsToProcess.put(item,event);
}
else if(st==Constants.BITSTREAM&&(et==Event.MODIFY||et==Event.MODIFY_METADATA))
{
Bitstream bitstream = (Bitstream) event.getSubject(ctx);
DSpaceObject dso = bitstream.getParentObject();
if(dso!=null&&dso.getType()==Constants.ITEM)
{
Item item = (Item)dso;
itemsToProcess.put(item,event);
}
}
}
public void end(Context ctx) throws Exception {
if(itemsToProcess != null){
AutoVersioningService versioningService = (AutoVersioningService) new DSpace().getSingletonService(VersioningService.class);
// TODO : We should probably use separate context here and don't dirty the existing one with our work.
for(Object obj : itemsToProcess.keySet()){
Collection events = itemsToProcess.getCollection(obj);
ctx.turnOffAuthorisationSystem();
try {
Item item = (Item) obj;
if (item != null && item.isArchived()) {
//case 1: submit a new item without entering workflow
//case 2: approve an item in workflow
String summary = "";
if(eventsToString(events).contains("INSTALL"))
{
summary = "Create New Item" ;
}
else
{
summary = new DSpace().getRequestService().getCurrentRequest().getHttpServletRequest().getParameter("summary");
if (summary == null) {
summary = "Modify Item";
}
}
Integer versionId = (Integer) new DSpace().getRequestService().getCurrentRequest().getAttribute("versionID");
if (versionId != null) {
versioningService.updateVersionHistory(ctx, item, versionId, summary, eventsToString(events), new Date());
} else {
versioningService.updateVersionHistory(ctx, item, summary, eventsToString(events), new Date());
}
ctx.getDBConnection().commit();
}
// TODO : Why are you updating the Item here? Leave it upto the service to decide.
//item.update();
// TODO : We should commit here and catch all exceptions?
} catch (Exception e) {
Throwables.propagate(e);
}
finally
{
ctx.restoreAuthSystemState();
}
}
}
itemsToProcess = null;
}
private String eventsToString(Collection events){
String s = "";
for(Event event : events)
{
s += event.toString() + "\n";
}
return s;
}
private static AutoVersionHistory retrieveVersionHistory(Context c, Item item) {
AutoVersioningService versioningService = (AutoVersioningService) new DSpace().getSingletonService(VersioningService.class);
return versioningService.findAutoVersionHistory(c, item.getID());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy