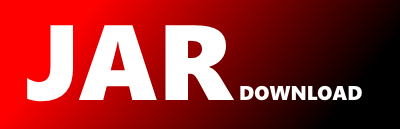
kilim.Pausable Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of kilim Show documentation
Show all versions of kilim Show documentation
Coroutines, continuations, fibers, actors and message passing for the JVM
/* Copyright (c) 2006, Sriram Srinivasan
*
* You may distribute this software under the terms of the license
* specified in the file "License"
*/
package kilim;
public class Pausable extends Exception {
private static final long serialVersionUID = 1L;
private Pausable() {}
private Pausable(String msg) {}
public interface Spawn {
TT execute() throws Pausable, Exception;
}
public interface Spawn10 extends Spawn {
TT execute(Fiber fiber) throws Pausable, Exception;
default TT execute() throws Pausable, Exception { return null; }
}
public interface Fork {
void execute() throws Pausable, Exception;
}
/**
* java 10 rejects woven lambdas similar to Fork, ie one's without the fiber argument.
* this subclass is compatible with java 10.
* the fiber argument should not be accessed in the lambda body,
* and invoking the lambda should use the `execute()` method
*/
public interface Fork10 extends Fork {
/**
* a functional interface for pausable code with a dummy fiber argument to support java 10.
* this method should never be invoked and the fiber argument should never be accessed in the lambda body
* @param dummy a dummy argument to enable weaving - don't access this argument
* @throws Pausable
* @throws Exception
*/
void execute(Fiber dummy) throws Pausable, Exception;
/**
* use this method to access the lambda - the weaver will delegate to the woven lambda
* @throws Pausable
* @throws Exception
*/
default void execute() throws Pausable, Exception {}
}
public interface Fork1 {
void execute(AA arg1) throws Pausable, Exception;
}
public interface Pfun { YY apply(XX obj) throws Pausable, EE; }
public interface Psumer { void apply(XX obj) throws Pausable, EE; }
public static
YY chain(XX obj,Pfun function) throws Pausable, EE {
return function.apply(obj);
}
public static
ZZ chain(X1 obj,
Pfun function1,
Pfun function2) throws Pausable, E1, E2 {
X2 obj2 = function1.apply(obj);
return function2.apply(obj2);
}
public static
X4 chain(X1 obj,
Pfun function1,
Pfun function2,
Pfun function3) throws Pausable, E1, E2, E3 {
X2 obj2 = function1.apply(obj);
X3 obj3 = function2.apply(obj2);
return function3.apply(obj3);
}
public static XX apply(XX obj,Psumer func) throws Pausable, EE {
func.apply(obj);
return obj;
}
public static
XX apply(XX obj,Psumer func1,Psumer func2) throws Pausable, E1, E2 {
func1.apply(obj);
func2.apply(obj);
return obj;
}
public static
XX apply(XX obj,
Psumer func1,
Psumer func2,
Psumer func3)
throws Pausable, E1, E2, E3 {
func1.apply(obj);
func2.apply(obj);
return obj;
}
public static XX applyAll(XX obj,Psumer ... funcs) throws Pausable, EE {
for (Psumer func : funcs)
func.apply(obj);
return obj;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy