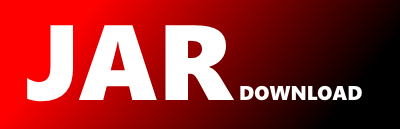
org.dbflute.cbean.chelper.HpSSQFunction Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of dbflute-runtime Show documentation
Show all versions of dbflute-runtime Show documentation
The runtime library of DBFlute
/*
* Copyright 2014-2015 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND,
* either express or implied. See the License for the specific language
* governing permissions and limitations under the License.
*/
package org.dbflute.cbean.chelper;
import org.dbflute.cbean.ConditionBean;
import org.dbflute.cbean.scoping.SubQuery;
/**
* The function for ScalarCondition (the old name: ScalarSubQuery).
* @param The type of condition-bean.
* @author jflute
*/
public class HpSSQFunction {
// ===================================================================================
// Attribute
// =========
protected final HpSSQSetupper _setupper;
// ===================================================================================
// Constructor
// ===========
public HpSSQFunction(HpSSQSetupper setupper) {
_setupper = setupper;
}
// ===================================================================================
// Function
// ========
/**
* Set up the sub query of myself for the scalar 'max'.
*
* cb.query().scalar_Equal().max(purchaseCB -> {
* public void query(PurchaseCB subCB) {
* purchaseCB.specify().columnPurchasePrice(); // *Point!
* purchaseCB.query().setPaymentCompleteFlg_Equal_True();
* }
* });
*
* @param scalarCBLambda The callback for sub-query of myself. (NotNull)
* @return The decorator of ScalarCondition. e.g. you can use partition-by. (NotNull)
*/
public HpSSQDecorator max(SubQuery scalarCBLambda) {
assertSubQuery(scalarCBLambda);
final HpSSQOption option = createOption();
_setupper.setup("max", scalarCBLambda, option);
return createDecorator(option);
}
/**
* Set up the sub query of myself for the scalar 'min'.
*
* cb.query().scalar_Equal().min(purchaseCB -> {
* public void query(PurchaseCB subCB) {
* purchaseCB.specify().columnPurchasePrice(); // *Point!
* purchaseCB.query().setPaymentCompleteFlg_Equal_True();
* }
* });
*
* @param scalarCBLambda The callback for sub-query of myself. (NotNull)
* @return The decorator of ScalarCondition. e.g. you can use partition-by. (NotNull)
*/
public HpSSQDecorator min(SubQuery scalarCBLambda) {
assertSubQuery(scalarCBLambda);
final HpSSQOption option = createOption();
_setupper.setup("min", scalarCBLambda, option);
return createDecorator(option);
}
/**
* Set up the sub query of myself for the scalar 'sum'.
*
* cb.query().scalar_Equal().sum(purchaseCB -> {
* purchaseCB.specify().columnPurchasePrice(); // *Point!
* purchaseCB.query().setPaymentCompleteFlg_Equal_True();
* });
*
* @param scalarCBLambda The callback for sub-query of myself. (NotNull)
* @return The decorator of ScalarCondition. e.g. you can use partition-by. (NotNull)
*/
public HpSSQDecorator sum(SubQuery scalarCBLambda) {
assertSubQuery(scalarCBLambda);
final HpSSQOption option = createOption();
_setupper.setup("sum", scalarCBLambda, option);
return createDecorator(option);
}
/**
* Set up the sub query of myself for the scalar 'avg'.
*
* cb.query().scalar_Equal().avg(purchaseCB -> {
* purchaseCB.specify().columnPurchasePrice(); // *Point!
* purchaseCB.query().setPaymentCompleteFlg_Equal_True();
* });
*
* @param scalarCBLambda The callback for sub-query of myself. (NotNull)
* @return The decorator of ScalarCondition. e.g. you can use partition-by. (NotNull)
*/
public HpSSQDecorator avg(SubQuery scalarCBLambda) {
assertSubQuery(scalarCBLambda);
final HpSSQOption option = createOption();
_setupper.setup("avg", scalarCBLambda, option);
return createDecorator(option);
}
// ===================================================================================
// Assist Helper
// =============
protected HpSSQOption createOption() {
return new HpSSQOption();
}
protected HpSSQDecorator createDecorator(HpSSQOption option) {
return new HpSSQDecorator(option);
}
protected void assertSubQuery(SubQuery> scalarCBLambda) {
if (scalarCBLambda == null) {
String msg = "The argument 'scalarCBLambda' for ScalarCondition should not be null.";
throw new IllegalArgumentException(msg);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy