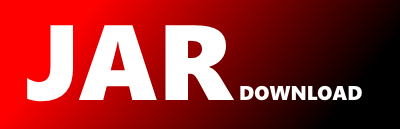
org.dbflute.bhv.referrer.LoadReferrerOption Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of dbflute-runtime Show documentation
Show all versions of dbflute-runtime Show documentation
The runtime library of DBFlute
/*
* Copyright 2014-2015 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND,
* either express or implied. See the License for the specific language
* governing permissions and limitations under the License.
*/
package org.dbflute.bhv.referrer;
import java.util.List;
import org.dbflute.Entity;
import org.dbflute.cbean.ConditionBean;
/**
* The class of load referrer option. #beforejava8
* This option is basically for loading second or more level referrer.
* @param The type of referrer condition-bean.
* @param The type of referrer entity.
* @author jflute
*/
public class LoadReferrerOption {
// ===================================================================================
// Attribute
// =========
protected ReferrerConditionSetupper _referrerConditionSetupper;
protected ConditionBeanSetupper _conditionBeanSetupper;
protected EntityListSetupper _entityListSetupper;
protected REFERRER_CB _referrerConditionBean;
// ===================================================================================
// Constructor
// ===========
/**
* Constructor.
* This option is basically for loading second or more level referrer like this:
*
* // base point table is MEMBER
* MemberCB cb = new MemberCB();
* ListResultBean<Member> memberList = memberBhv.selectList(cb);
*
* LoadReferrerOption option = new LoadReferrerOption();
*
* // PURCHASE (first level referrer from MEMBER)
* option.setReferrerConditionSetupper(new ReferrerConditionSetupper<PurchaseCB>() {
* public void setup(PurchaseCB cb) {
* cb.query().addOrderBy_PurchaseDatetime_Desc();
* }
* });
*
* // PURCHASE_DETAIL (second level referrer from PURCHASE)
* option.setEntityListSetupper(new EntityListSetupper<Purchase>() {
* public void setup(List<Purchase> entityList) {
* purchaseBhv.loadPurchaseDetailList(entityList, new ConditionBeanSetupper<PurchaseDetailCB>() {
* public void setup(PurchaseDetailCB cb) {
* ...
* }
* });
* }
* });
*
* memberStatusBhv.loadMemberList(memberList, option);
*
*/
public LoadReferrerOption() {
}
public LoadReferrerOption xinit(ReferrerConditionSetupper referrerConditionSetupper) { // internal
setReferrerConditionSetupper(referrerConditionSetupper);
return this;
}
public LoadReferrerOption xinit(ConditionBeanSetupper conditionBeanSetupper) { // internal
setConditionBeanSetupper(conditionBeanSetupper);
return this;
}
// ===================================================================================
// Easy-to-Use
// ===========
public void delegateConditionBeanSettingUp(REFERRER_CB cb) { // internal
if (_referrerConditionSetupper != null) {
_referrerConditionSetupper.setup(cb);
} else if (_conditionBeanSetupper != null) {
_conditionBeanSetupper.setup(cb);
}
}
public void delegateEntitySettingUp(List entityList) { // internal
if (_entityListSetupper != null) {
_entityListSetupper.setup(entityList);
}
}
// ===================================================================================
// Accessor
// ========
public ReferrerConditionSetupper getReferrerConditionSetupper() {
return _conditionBeanSetupper;
}
/**
* Set the set-upper of condition-bean for first level referrer.
*
* LoadReferrerOption option = new LoadReferrerOption();
*
* // PURCHASE (first level referrer from MEMBER)
* option.setReferrerConditionSetupper(new ReferrerConditionSetupper<PurchaseCB>() {
* public void setup(PurchaseCB cb) {
* cb.query().addOrderBy_PurchaseDatetime_Desc();
* }
* });
* ...
*
* @param referrerConditionSetupper The set-upper of condition-bean for referrer. (NullAllowed: if null, means no condition for a first level referrer)
*/
public void setReferrerConditionSetupper(ReferrerConditionSetupper referrerConditionSetupper) {
_referrerConditionSetupper = referrerConditionSetupper;
}
public ConditionBeanSetupper getConditionBeanSetupper() {
return _conditionBeanSetupper;
}
/**
* Set the set-upper of condition-bean for first level referrer.
*
* LoadReferrerOption option = new LoadReferrerOption();
*
* // PURCHASE (first level referrer from MEMBER)
* option.setConditionBeanSetupper(new ConditionBeanSetupper<PurchaseCB>() {
* public void setup(PurchaseCB cb) {
* cb.query().addOrderBy_PurchaseDatetime_Desc();
* }
* });
* ...
*
* @param conditionBeanSetupper The set-upper of condition-bean. (NullAllowed: if null, means no condition for a first level referrer)
*/
public void setConditionBeanSetupper(ConditionBeanSetupper conditionBeanSetupper) {
_conditionBeanSetupper = conditionBeanSetupper;
}
public EntityListSetupper getEntityListSetupper() {
return _entityListSetupper;
}
/**
* Set the set-upper of entity list for second or more level referrer.
*
* LoadReferrerOption loadReferrerOption = new LoadReferrerOption();
* ...
* // PURCHASE (second level referrer)
* loadReferrerOption.setEntityListSetupper(new EntityListSetupper<Member>() {
* public void setup(List<Member> entityList) {
* memberBhv.loadPurchaseList(entityList, new ConditionBeanSetupper<PurchaseCB>() {
* public void setup(PurchaseCB cb) {
* cb.query().addOrderBy_PurchaseCount_Desc();
* cb.query().addOrderBy_ProductId_Desc();
* }
* });
* }
* });
*
* @param entityListSetupper The set-upper of entity list. (NullAllowed: if null, means no loading for second level referrer)
*/
public void setEntityListSetupper(EntityListSetupper entityListSetupper) {
this._entityListSetupper = entityListSetupper;
}
public REFERRER_CB getReferrerConditionBean() {
return _referrerConditionBean;
}
/**
* Set the original instance of condition-bean for first level referrer.
* use this, if you want to set the original instance.
* @param referrerConditionBean The original instance of condition-bean. (NullAllowed: if null, means normal)
*/
public void setReferrerConditionBean(REFERRER_CB referrerConditionBean) {
this._referrerConditionBean = referrerConditionBean;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy