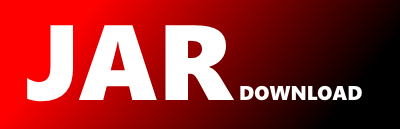
org.dbflute.bhv.logging.invoke.ByPassInvokeNameExtractor Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of dbflute-runtime Show documentation
Show all versions of dbflute-runtime Show documentation
The runtime library of DBFlute
/*
* Copyright 2014-2019 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND,
* either express or implied. See the License for the specific language
* governing permissions and limitations under the License.
*/
package org.dbflute.bhv.logging.invoke;
import java.util.List;
import org.dbflute.helper.stacktrace.InvokeNameExtractingResource;
import org.dbflute.helper.stacktrace.InvokeNameResult;
/**
* @author jflute
* @since 1.0.4D (2013/06/30 Sunday)
*/
public class ByPassInvokeNameExtractor {
// ===================================================================================
// Attribute
// =========
protected final List _suffixList;
protected final int _startIndex;
protected final int _loopSize;
protected final InvokeNameExtractingCoinLogic _coinLogic = createInvokeNameExtractingCoinLogic();
protected InvokeNameExtractingCoinLogic createInvokeNameExtractingCoinLogic() {
return new InvokeNameExtractingCoinLogic();
}
// ===================================================================================
// Constructor
// ===========
public ByPassInvokeNameExtractor(List suffixList, int startIndex, int loopSize) {
_suffixList = suffixList;
_startIndex = startIndex;
_loopSize = loopSize;
}
// ===================================================================================
// Extract ByPass
// ==============
public ByPassInvokeNameResult extractByPassInvoke(StackTraceElement[] stackTrace) {
final InvokeNameExtractingResource resource = new InvokeNameExtractingResource() {
public boolean isTargetElement(String className, String methodName) {
return isClassNameEndsWith(className, _suffixList);
}
public String filterSimpleClassName(String simpleClassName) {
return simpleClassName;
}
public boolean isUseAdditionalInfo() {
return true;
}
public int getStartIndex() {
return _startIndex;
}
public int getLoopSize() {
return _loopSize >= 0 ? _loopSize : getInvocationExtractingMaxLoopSize();
}
};
final List invokeNameResultList = extractInvokeName(resource, stackTrace);
return new ByPassInvokeNameResult(invokeNameResultList);
}
protected int getInvocationExtractingMaxLoopSize() {
return 15;
}
// ===================================================================================
// Extract Invoke Name
// ===================
/**
* @param resource the call-back resource for invoke-name-extracting. (NotNull)
* @param stackTrace Stack log. (NotNull)
* @return The list of result of invoke name. (NotNull: If not found, returns empty string.)
*/
protected List extractInvokeName(InvokeNameExtractingResource resource, StackTraceElement[] stackTrace) {
return _coinLogic.extractInvokeName(resource, stackTrace);
}
// ===================================================================================
// Assist Helper
// =============
protected boolean isClassNameEndsWith(String className, List suffixList) {
return _coinLogic.isClassNameEndsWith(className, suffixList);
}
protected boolean isClassNameContains(String className, List keywordList) {
return _coinLogic.isClassNameContains(className, keywordList);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy