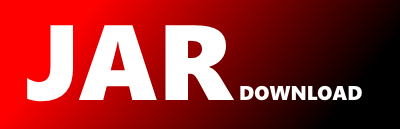
org.dbflute.cbean.result.ListResultBean Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of dbflute-runtime Show documentation
Show all versions of dbflute-runtime Show documentation
The runtime library of DBFlute
/*
* Copyright 2014-2019 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND,
* either express or implied. See the License for the specific language
* governing permissions and limitations under the License.
*/
package org.dbflute.cbean.result;
import java.io.Serializable;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Iterator;
import java.util.LinkedHashMap;
import java.util.LinkedHashSet;
import java.util.List;
import java.util.ListIterator;
import java.util.Map;
import java.util.NoSuchElementException;
import java.util.Set;
import org.dbflute.Entity;
import org.dbflute.cbean.result.grouping.GroupingListDeterminer;
import org.dbflute.cbean.result.grouping.GroupingListRowResource;
import org.dbflute.cbean.result.grouping.GroupingMapDeterminer;
import org.dbflute.cbean.result.mapping.EntityColumnExtractor;
import org.dbflute.cbean.result.mapping.EntityDtoMapper;
import org.dbflute.cbean.sqlclause.orderby.OrderByClause;
import org.dbflute.util.DfTypeUtil;
/**
* The result bean for list.
* You can treat it as normal List, because this implements java.util.List.
*
* [Extension Method]
* mappingList() : mapping to other class, and returns as list
* groupingList() : grouping the list per category, and returns as list
* groupingMap() : grouping the list per category, and returns as map
* extractColumnList() : extract one column as value list
* extractColumnSet() : extract one column as value list
*
* @param The type of entity for the element of selected list.
* @author jflute
*/
public class ListResultBean implements List, Serializable {
// ===================================================================================
// Definition
// ==========
/** The serial version UID for object serialization. (Default) */
private static final long serialVersionUID = 1L;
// ===================================================================================
// Attribute
// =========
/** The DB name of table. (NullAllowed: if null, means not-selected-yet) */
protected String _tableDbName;
/** The count of all record. */
protected int _allRecordCount;
/** The clause of order-by. (NullAllowed: lazy-loaded) */
protected transient OrderByClause _orderByClause; // #later: removed
/** The list of selected entity. (NullAllowed: lazy-loaded) */
protected List _selectedList;
// ===================================================================================
// Mapping
// =======
/**
* Map the entity list to the list of other object.
*
* ListResultBean<MemberWebBean> beanList = memberList.mappingList(member -> {
* MemberWebBean bean = new MemberWebBean();
* bean.setMemberId(member.getMemberId());
* bean.setMemberName(member.getMemberName());
* ...
* return bean;
* });
*
* This method needs the property 'selectedList' only.
* @param The type of DTO.
* @param entityLambda The callback for mapping of entity and DTO. (NotNull)
* @return The new mapped list as result bean. (NotNull)
*/
public ListResultBean mappingList(EntityDtoMapper entityLambda) {
final List mappingList;
if (hasWrappedListInstance()) {
final List selectedList = getSelectedList();
mappingList = new ArrayList(selectedList.size());
for (ENTITY entity : selectedList) {
mappingList.add(entityLambda.map(entity));
}
} else {
mappingList = null;
}
return createInheritedResultBean(mappingList);
}
// ===================================================================================
// Grouping
// ========
/**
* Return grouping list (split the list per group).
* This method needs the property 'selectedList' only.
*
* // e.g. grouping per three records
* List<ListResultBean<Member>> groupingList = memberList.groupingList(new GroupingListDeterminer<Member>() {
* public boolean isBreakRow(GroupingListRowResource<Member> rowResource, Member nextEntity) {
* return rowResource.getNextIndex() >= 3;
* }
* }, groupingOption);
*
* // e.g. grouping per initial character of MEMBER_NAME
* List<ListResultBean<Member>> groupingList = memberList.groupingList(new GroupingListDeterminer<Member>() {
* public boolean isBreakRow(GroupingListRowResource<Member> rowResource, Member nextEntity) {
* Member currentEntity = rowResource.getCurrentEntity();
* String currentInitChar = currentEntity.getMemberName().substring(0, 1);
* String nextInitChar = nextEntity.getMemberName().substring(0, 1);
* return !currentInitChar.equalsIgnoreCase(nextInitChar);
* }
* });
*
* @param determiner The determiner of grouping list. (NotNull)
* @return The grouping list that has grouped rows. (NotNull)
*/
public List> groupingList(GroupingListDeterminer determiner) {
final List> groupingRowList = new ArrayList>();
if (!hasWrappedListInstance()) {
return groupingRowList; // not read-only
}
GroupingListRowResource rowResource = new GroupingListRowResource();
int rowElementIndex = 0;
int wholeElementIndex = 0;
final List selectedList = getSelectedList();
for (ENTITY entity : selectedList) {
// set up row resource
rowResource.addGroupingEntity(entity);
rowResource.setCurrentIndex(rowElementIndex);
if (selectedList.size() == (wholeElementIndex + 1)) { // last loop
groupingRowList.add(createInheritedResultBean(rowResource.getGroupingEntityList()));
break;
}
// not last loop so the nextElement must exist
final ENTITY nextElement = selectedList.get(wholeElementIndex + 1);
// do at row end
if (determiner.isBreakRow(rowResource, nextElement)) { // determine the row end
groupingRowList.add(createInheritedResultBean(rowResource.getGroupingEntityList()));
rowResource = new GroupingListRowResource(); // for the next row
rowElementIndex = 0;
++wholeElementIndex;
continue;
}
++rowElementIndex;
++wholeElementIndex;
}
return groupingRowList;
}
/**
* Return grouping map (split the list per group key).
* This method needs the property 'selectedList' only.
*
* // e.g. grouping per initial character of MEMBER_NAME
* Map<String, ListResultBean<Member>> groupingMap = memberList.groupingMap(member -> {
* return member.getMemberName().substring(0, 1);
* });
*
* @param entityLambda The callback for determiner of grouping map. (NotNull)
* @return The grouping map that has grouped rows, the key is provided. (NotNull)
*/
public Map> groupingMap(GroupingMapDeterminer entityLambda) {
final Map> groupingListMap = new LinkedHashMap>();
if (!hasWrappedListInstance()) {
return groupingListMap; // not read-only
}
for (ENTITY entity : getSelectedList()) {
final String groupingKey = entityLambda.provideKey(entity);
ListResultBean rowList = groupingListMap.get(groupingKey);
if (rowList == null) {
rowList = createInheritedResultBean(null); // no set selectedList
groupingListMap.put(groupingKey, rowList);
}
rowList.add(entity);
}
return groupingListMap;
}
// ===================================================================================
// Extract Column
// ==============
/**
* Extract the value list of the column specified in extractor.
*
* List<Integer> memberIdList = memberList.extractColumnList(member -> {
* return entity.getMemberId();
* });
*
* This method needs the property 'selectedList' only.
* @param The type of COLUMN.
* @param entityLambda The callback for value extractor of entity column. (NotNull)
* @return The value list of the entity column. (NotNull, NotNullElement)
*/
public List extractColumnList(EntityColumnExtractor entityLambda) {
if (!hasWrappedListInstance()) {
return new ArrayList(2); // not read-only
}
final List selectedList = getSelectedList();
final List columnList = new ArrayList(selectedList.size());
for (ENTITY entity : selectedList) {
final COLUMN column = entityLambda.extract(entity);
if (column != null) {
columnList.add(column);
}
}
return columnList;
}
/**
* Extract the value set of the column specified in extractor.
*
* Set<Integer> memberIdList = memberList.extractColumnSet(member -> {
* return entity.getMemberId();
* });
*
* This method needs the property 'selectedList' only.
* @param The type of COLUMN.
* @param entityLambda The callback for value extractor of entity column. (NotNull)
* @return The value set of the entity column. (NotNull, NotNullElement)
*/
public Set extractColumnSet(EntityColumnExtractor entityLambda) {
if (!hasWrappedListInstance()) {
return new LinkedHashSet(2); // not read-only
}
final List selectedList = getSelectedList();
final Set columnSet = new LinkedHashSet(selectedList.size());
for (ENTITY entity : selectedList) {
COLUMN column = entityLambda.extract(entity);
if (column != null) {
columnSet.add(column);
}
}
return columnSet;
}
// ===================================================================================
// Determination
// =============
/**
* Is this result by selected?
* @return The determination, true or false. {whether table DB name is not null}
*/
public boolean isSelectedResult() {
return _tableDbName != null;
}
// ===================================================================================
// Inherited ResultBean
// ====================
protected ListResultBean createInheritedResultBean(List selectedList) {
final ResultBeanBuilder builder = newResultBeanBuilder(getTableDbName());
return builder.buildListInherited(this, selectedList);
}
protected ResultBeanBuilder newResultBeanBuilder(String tableDbName) {
return new ResultBeanBuilder(tableDbName);
}
// ===================================================================================
// Basic Override
// ==============
/**
* @return Hash-code from primary-keys.
*/
public int hashCode() {
int result = 17;
if (hasWrappedListInstance()) {
result = (31 * result) + getSelectedList().hashCode();
}
return result;
}
/**
* @param other Other entity. (NullAllowed)
* @return Comparing result. If other is null, returns false.
*/
public boolean equals(Object other) {
if (other == null) {
return false;
}
if (!(other instanceof List>)) {
return false;
}
if (!hasWrappedListInstance()) {
return false; // basically unreachable
}
if (other instanceof ListResultBean>) {
return getSelectedList().equals(((ListResultBean>) other).getSelectedList());
} else {
return getSelectedList().equals(other);
}
}
/**
* @return The view string of all attribute values. (NotNull)
*/
@Override
public String toString() {
final StringBuilder sb = new StringBuilder();
sb.append("{").append(_tableDbName).append(", ").append(_allRecordCount).append(", ").append(_selectedList).append("}");
return sb.toString();
}
/**
* @return The rich string of all record values. (NotNull)
*/
public String toRichString() {
final StringBuilder sb = new StringBuilder();
sb.append(DfTypeUtil.toClassTitle(this)).append(" Show:");
final StringBuilder headerSb = new StringBuilder();
buildRichStringHeader(headerSb);
final String header = headerSb.toString();
sb.append(!header.isEmpty() ? (header.contains("\n") ? "\n" : " ") : "").append(headerSb);
if (!isEmpty()) {
forEach(entity -> {
sb.append("\n");
if (entity instanceof Entity) {
sb.append(((Entity) entity).toStringWithRelation());
} else {
sb.append(entity.toString());
}
});
} else {
sb.append(" ").append("*empty");
}
return sb.toString();
}
protected void buildRichStringHeader(StringBuilder sb) {
}
// ===================================================================================
// List Elements
// =============
public boolean add(ENTITY o) {
return getSelectedList().add(o);
}
public boolean addAll(Collection extends ENTITY> c) {
return getSelectedList().addAll(c);
}
public void clear() {
if (hasWrappedListInstance()) {
getSelectedList().clear();
}
}
public boolean contains(Object o) {
return hasWrappedListInstance() ? getSelectedList().contains(o) : false;
}
public boolean containsAll(Collection> c) {
return hasWrappedListInstance() ? getSelectedList().containsAll(c) : false;
}
public boolean isEmpty() {
return hasWrappedListInstance() ? getSelectedList().isEmpty() : true; // empty if no instance
}
public Iterator iterator() {
return hasWrappedListInstance() ? getSelectedList().iterator() : createEmptyIterator();
}
protected Iterator createEmptyIterator() {
return new Iterator() {
public boolean hasNext() {
return false;
}
public ENTITY next() {
throw new NoSuchElementException();
}
};
}
public boolean remove(Object o) {
return getSelectedList().remove(o);
}
public boolean removeAll(Collection> c) {
return getSelectedList().removeAll(c);
}
public boolean retainAll(Collection> c) {
return getSelectedList().retainAll(c);
}
public int size() {
return getSelectedList().size();
}
public Object[] toArray() {
return getSelectedList().toArray();
}
public TYPE[] toArray(TYPE[] a) {
return getSelectedList().toArray(a);
}
public void add(int index, ENTITY element) {
getSelectedList().add(index, element);
}
public boolean addAll(int index, Collection extends ENTITY> c) {
return getSelectedList().addAll(index, c);
}
public ENTITY get(int index) {
return getSelectedList().get(index);
}
public int indexOf(Object o) {
return getSelectedList().indexOf(o);
}
public int lastIndexOf(Object o) {
return getSelectedList().lastIndexOf(o);
}
public ListIterator listIterator() {
return getSelectedList().listIterator();
}
public ListIterator listIterator(int index) {
return getSelectedList().listIterator(index);
}
public ENTITY remove(int index) {
return getSelectedList().remove(index);
}
public ENTITY set(int index, ENTITY element) {
return getSelectedList().set(index, element);
}
public List subList(int fromIndex, int toIndex) {
return getSelectedList().subList(fromIndex, toIndex);
}
// ===================================================================================
// Accessor
// ========
/**
* Get the value of tableDbName.
* @return The DB name of table. (NullAllowed: if null, it means not-selected-yet)
*/
public String getTableDbName() {
return _tableDbName;
}
/**
* Set the value of tableDbName.
* @param tableDbName The DB name of table. (NotNull)
*/
public void setTableDbName(String tableDbName) {
_tableDbName = tableDbName;
}
/**
* Get the value of allRecordCount.
* @return The count of all records.
*/
public int getAllRecordCount() {
return _allRecordCount;
}
/**
* Set the value of allRecordCount.
* @param allRecordCount The count of all records.
*/
public void setAllRecordCount(int allRecordCount) {
_allRecordCount = allRecordCount;
}
/**
* Get the value of orderByClause.
* @return The value of orderByClause. (NotNull)
* @deprecated don't use this
*/
public OrderByClause getOrderByClause() {
if (_orderByClause == null) {
_orderByClause = new OrderByClause();
}
return _orderByClause;
}
/**
* Set the value of orderByClause.
* @param orderByClause The value of orderByClause. (NullAllowed)
*/
public void setOrderByClause(OrderByClause orderByClause) {
_orderByClause = orderByClause;
}
/**
* Get the value of selectedList.
* @return Selected list. (NotNull)
*/
public List getSelectedList() {
if (_selectedList == null) {
_selectedList = new ArrayList();
}
return _selectedList;
}
/**
* Set the value of selectedList.
* @param selectedList Selected list. (NullAllowed: if null, clear the list)
*/
public void setSelectedList(List selectedList) {
_selectedList = selectedList;
}
protected boolean hasWrappedListInstance() {
return _selectedList != null;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy