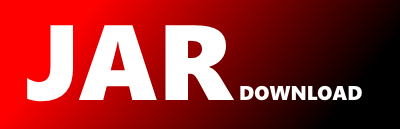
org.dbflute.cbean.sqlclause.query.OrScopeQueryInfo Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of dbflute-runtime Show documentation
Show all versions of dbflute-runtime Show documentation
The runtime library of DBFlute
/*
* Copyright 2014-2019 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND,
* either express or implied. See the License for the specific language
* governing permissions and limitations under the License.
*/
package org.dbflute.cbean.sqlclause.query;
import java.util.ArrayList;
import java.util.LinkedHashMap;
import java.util.List;
import java.util.Map;
/**
* @author jflute
*/
public class OrScopeQueryInfo {
// ===================================================================================
// Attribute
// =========
protected List _tmpOrWhereList;
protected List _tmpOrBaseTableInlineWhereList;
protected Map> _tmpOrAdditionalOnClauseListMap;
protected Map> _tmpOrOuterJoinInlineClauseListMap;
protected OrScopeQueryInfo _parentInfo; // null means base point
protected List _childInfoList;
// ===================================================================================
// Tmp List
// ========
public List getTmpOrAdditionalOnClauseList(String aliasName) {
List orClauseList = getTmpOrAdditionalOnClauseListMap().get(aliasName);
if (orClauseList != null) {
return orClauseList;
}
orClauseList = new ArrayList();
_tmpOrAdditionalOnClauseListMap.put(aliasName, orClauseList);
return orClauseList;
}
public List getTmpOrOuterJoinInlineClauseList(String aliasName) {
List orClauseList = getTmpOrOuterJoinInlineClauseListMap().get(aliasName);
if (orClauseList != null) {
return orClauseList;
}
orClauseList = new ArrayList();
_tmpOrOuterJoinInlineClauseListMap.put(aliasName, orClauseList);
return orClauseList;
}
public List getTmpOrWhereList() {
if (_tmpOrWhereList == null) {
_tmpOrWhereList = new ArrayList(4);
}
return _tmpOrWhereList;
}
public void setTmpOrWhereList(List tmpOrWhereList) {
this._tmpOrWhereList = tmpOrWhereList;
}
public List getTmpOrBaseTableInlineWhereList() {
if (_tmpOrBaseTableInlineWhereList == null) {
_tmpOrBaseTableInlineWhereList = new ArrayList(2);
}
return _tmpOrBaseTableInlineWhereList;
}
public void setTmpOrBaseTableInlineWhereList(List tmpOrBaseTableInlineWhereList) {
this._tmpOrBaseTableInlineWhereList = tmpOrBaseTableInlineWhereList;
}
public Map> getTmpOrAdditionalOnClauseListMap() {
if (_tmpOrAdditionalOnClauseListMap == null) {
_tmpOrAdditionalOnClauseListMap = new LinkedHashMap>(2);
}
return _tmpOrAdditionalOnClauseListMap;
}
public void setTmpOrAdditionalOnClauseListMap(Map> tmpOrAdditionalOnClauseListMap) {
this._tmpOrAdditionalOnClauseListMap = tmpOrAdditionalOnClauseListMap;
}
public Map> getTmpOrOuterJoinInlineClauseListMap() {
if (_tmpOrOuterJoinInlineClauseListMap == null) {
_tmpOrOuterJoinInlineClauseListMap = new LinkedHashMap>(2);
}
return _tmpOrOuterJoinInlineClauseListMap;
}
public void setTmpOrOuterJoinInlineClauseListMap(Map> tmpOrOuterJoinInlineClauseListMap) {
this._tmpOrOuterJoinInlineClauseListMap = tmpOrOuterJoinInlineClauseListMap;
}
// ===================================================================================
// Child Parent Info
// =================
public boolean hasChildInfo() {
return _childInfoList != null && !_childInfoList.isEmpty();
}
public OrScopeQueryInfo getParentInfo() {
return _parentInfo;
}
public void setParentInfo(OrScopeQueryInfo parentInfo) {
_parentInfo = parentInfo;
}
public List getChildInfoList() {
if (_childInfoList == null) {
_childInfoList = new ArrayList();
}
return _childInfoList;
}
public void addChildInfo(OrScopeQueryInfo childInfo) {
childInfo.setParentInfo(this);
getChildInfoList().add(childInfo);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy