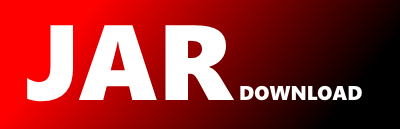
org.dbflute.helper.jprop.JavaPropertiesReader Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of dbflute-runtime Show documentation
Show all versions of dbflute-runtime Show documentation
The runtime library of DBFlute
/*
* Copyright 2014-2019 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND,
* either express or implied. See the License for the specific language
* governing permissions and limitations under the License.
*/
package org.dbflute.helper.jprop;
import java.io.BufferedReader;
import java.io.ByteArrayInputStream;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.io.UnsupportedEncodingException;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Collections;
import java.util.Comparator;
import java.util.HashMap;
import java.util.LinkedHashMap;
import java.util.List;
import java.util.Map;
import java.util.Map.Entry;
import java.util.Properties;
import java.util.Set;
import java.util.stream.Collectors;
import org.dbflute.helper.jprop.exception.JavaPropertiesImplicitOverrideException;
import org.dbflute.helper.jprop.exception.JavaPropertiesLonelyOverrideException;
import org.dbflute.helper.jprop.exception.JavaPropertiesReadFailureException;
import org.dbflute.helper.jprop.exception.JavaPropertiesStreamNotFoundException;
import org.dbflute.helper.message.ExceptionMessageBuilder;
import org.dbflute.util.DfCollectionUtil;
import org.dbflute.util.DfCollectionUtil.AccordingToOrderIdExtractor;
import org.dbflute.util.DfCollectionUtil.AccordingToOrderResource;
import org.dbflute.util.Srl;
import org.dbflute.util.Srl.ScopeInfo;
/**
* @author jflute
* @since 1.0.1 (2012/12/15 Saturday)
*/
public class JavaPropertiesReader {
// ===================================================================================
// Definition
// ==========
public static final String OVERRIDE_ANNOTATION = "@Override";
public static final String SECURE_ANNOTATION = "@Secure";
// ===================================================================================
// Attribute
// =========
// -----------------------------------------------------
// Basic
// -----
protected final String _title;
protected final JavaPropertiesStreamProvider _streamProvider;
// -----------------------------------------------------
// Option
// ------
protected final Map _extendsProviderMap = newLinkedHashMapSized(4);
protected boolean _checkImplicitOverride;
protected String _streamEncoding; // used if set
protected boolean _useNonNumberVariable;
protected Set _variableExceptSet; // used if set
protected boolean _suppressVariableOrder; // for compatible
// to avoid Java11 warning
//// -----------------------------------------------------
//// Reflection
//// ----------
//protected Method _convertMethod; // cached
//protected boolean _convertMethodNotFound;
//protected final Properties _reflectionProperties = new Properties();
// ===================================================================================
// Constructor
// ===========
public JavaPropertiesReader(String title, JavaPropertiesStreamProvider streamProvider) {
_title = title;
_streamProvider = streamProvider;
}
// -----------------------------------------------------
// Option
// ------
public JavaPropertiesReader extendsProperties(String title, JavaPropertiesStreamProvider noArgLambda) {
if (_extendsProviderMap.containsKey(title)) {
String msg = "The argument 'title' has already been registered:";
msg = msg + " title=" + title + " registered=" + _extendsProviderMap.keySet();
throw new IllegalArgumentException(msg);
}
_extendsProviderMap.put(title, noArgLambda);
return this;
}
public JavaPropertiesReader checkImplicitOverride() {
_checkImplicitOverride = true;
return this;
}
public JavaPropertiesReader encodeAsUTF8() {
_streamEncoding = "UTF-8";
return this;
}
public JavaPropertiesReader encodeAs(String encoding) {
_streamEncoding = encoding;
return this;
}
public JavaPropertiesReader useNonNumberVariable() {
_useNonNumberVariable = true;
return this;
}
public JavaPropertiesReader useVariableExcept(Set variableExceptSet) {
if (variableExceptSet == null) {
throw new IllegalArgumentException("The argument 'variableExceptSet' should not be null.");
}
_variableExceptSet = variableExceptSet;
return this;
}
public JavaPropertiesReader suppressVariableOrder() { // for compatible
_suppressVariableOrder = true;
return this;
}
// ===================================================================================
// Read
// ====
public JavaPropertiesResult read() {
final List propertyList = newArrayList();
final List duplicateKeyList = newArrayList();
final Map keyCommentMap = readKeyCommentMap(duplicateKeyList);
final Properties prop = readPlainProperties();
final List keyList = orderKeyList(prop, keyCommentMap);
for (String key : keyList) {
final String value = prop.getProperty(key);
final String comment = keyCommentMap.get(key);
final JavaPropertiesProperty property = new JavaPropertiesProperty(key, value);
final String defName = Srl.replace(key, ".", "_").toUpperCase();
property.setDefName(defName);
final String camelizedName = Srl.camelize(defName);
property.setCamelizedName(camelizedName);
property.setCapCamelName(Srl.initCap(camelizedName));
property.setUncapCamelName(Srl.initUncap(camelizedName));
final List variableScopeList = analyzeVariableScopeList(value);
final List variableNumberList = DfCollectionUtil.newArrayListSized(variableScopeList.size());
final List variableStringList = DfCollectionUtil.newArrayListSized(variableScopeList.size());
reflectToVariableList(key, variableScopeList, variableNumberList, variableStringList);
property.setVariableNumberList(variableNumberList);
property.setVariableStringList(variableStringList);
final List variableArgNameList = prepareVariableArgNameList(variableStringList);
property.setVariableArgNameList(variableArgNameList);
property.setVariableArgDef(buildVariableArgDef(variableArgNameList));
property.setVariableArgSet(buildVariableArgSet(variableArgNameList));
property.setComment(comment);
if (containsSecureAnnotation(property)) {
property.toBeSecure();
}
propertyList.add(property);
}
return prepareResult(prop, propertyList, duplicateKeyList);
}
protected List analyzeVariableScopeList(String value) {
final List variableScopeList = newArrayList();
{
final List scopeList;
if (Srl.is_NotNull_and_NotTrimmedEmpty(value)) {
scopeList = extractVariableScopeList(value);
} else {
scopeList = DfCollectionUtil.emptyList();
}
for (ScopeInfo scopeInfo : scopeList) {
final String content = scopeInfo.getContent();
if (isUnsupportedVariableContent(content)) {
continue;
}
variableScopeList.add(scopeInfo);
}
}
orderVariableScopeList(variableScopeList);
return variableScopeList;
}
protected List extractVariableScopeList(String value) {
return Srl.extractScopeList(value, "{", "}"); // e.g. {0}, {1}
}
protected boolean isUnsupportedVariableContent(String content) {
if (content.contains(" ")) { // e.g. {sea land}
return true;
}
if (!_useNonNumberVariable && !Srl.isNumberHarfAll(content)) { // number only but non-number
// e.g. {sea}, {land}
return true;
}
if (_variableExceptSet != null && _variableExceptSet.contains(content)) { // e.g. {item} in LastaFlute
return true;
}
return false;
}
protected void orderVariableScopeList(List variableScopeList) {
if (_suppressVariableOrder) { // for compatible
return;
}
// should be ordered for MessageFormat by jflute (2017/08/19)
final VariableOrderAgent orderAgent = createVariableOrderAgent();
orderAgent.orderScopeList(variableScopeList);
}
protected VariableOrderAgent createVariableOrderAgent() {
return new VariableOrderAgent();
}
public static class VariableOrderAgent { // can be used other libraries
public void orderScopeList(List variableScopeList) {
Collections.sort(variableScopeList, (o1, o2) -> { // ...after all, split indexed and named
return Srl.isNumberHarfAll(o1.getContent()) ? -1 : 0;
});
orderIndexedOnly(variableScopeList); // e.g. {2}-{sea}-{0}-{land}-{1} to {0}-{sea}-{1}-{land}-{2}
orderNamedOnly(variableScopeList); // e.g. {2}-{sea}-{0}-{land}-{1} to {0}-{land}-{1}-{sea}-{2}
}
protected void orderIndexedOnly(List variableScopeList) {
final Map namedMap = new LinkedHashMap();
for (int i = 0; i < variableScopeList.size(); i++) {
final ScopeInfo element = variableScopeList.get(i);
if (!Srl.isNumberHarfAll(element.getContent())) {
namedMap.put(i, element);
}
}
final List sortedList = variableScopeList.stream()
.filter(el -> Srl.isNumberHarfAll(el.getContent()))
.sorted(Comparator.comparing(el -> filterNumber(el.getContent()), Comparator.naturalOrder()))
.collect(Collectors.toList());
namedMap.forEach((key, value) -> {
sortedList.add(key, value);
});
variableScopeList.clear();
variableScopeList.addAll(sortedList);
}
protected String filterNumber(String el) {
final String ltrimmed = Srl.ltrim(el, "0"); // zero suppressed e.g. "007" to "7"
if (ltrimmed.isEmpty() && el.contains("0")) { // e.g. "000"
return "0";
} else {
return ltrimmed;
}
}
protected void orderNamedOnly(List variableScopeList) {
final Map indexedMap = new LinkedHashMap();
for (int i = 0; i < variableScopeList.size(); i++) {
final ScopeInfo element = variableScopeList.get(i);
if (Srl.isNumberHarfAll(element.getContent())) {
indexedMap.put(i, element);
}
}
final List sortedList = variableScopeList.stream().filter(el -> {
return !Srl.isNumberHarfAll(el.getContent());
}).sorted((o1, o2) -> {
final String v1 = o1.getContent();
final String v2 = o2.getContent();
if (isSpecialNamedOrder(v1, v2)) {
return -1;
} else if (isSpecialNamedOrder(v2, v1)) {
return 1;
}
return v1.compareTo(v2);
}).collect(Collectors.toList());
indexedMap.forEach((key, value) -> {
sortedList.add(key, value);
});
variableScopeList.clear();
variableScopeList.addAll(sortedList);
}
protected boolean isSpecialNamedOrder(String v1, String v2) {
return v1.equals("min") && v2.equals("max") // used by e.g. Hibernate Validator
|| v1.equals("minimum") && v2.equals("maximum") //
|| v1.equals("start") && v2.equals("end") //
|| v1.equals("before") && v2.equals("after") //
;
}
}
protected void reflectToVariableList(String key, List variableScopeList, List variableNumberList,
List variableStringList) {
for (ScopeInfo scopeInfo : variableScopeList) {
final String content = scopeInfo.getContent();
final Integer variableNumber = valueOfVariableNumber(key, content);
if (variableNumber != null) { // for non-number option
variableNumberList.add(variableNumber);
}
variableStringList.add(content); // contains all elements
}
}
protected List prepareVariableArgNameList(List variableStringList) {
final List variableArgNameList = DfCollectionUtil.newArrayListSized(variableStringList.size());
for (Object name : variableStringList) {
variableArgNameList.add(doBuildVariableArgName(name));
}
return variableArgNameList;
}
protected String doBuildVariableArgName(Object name) {
return startsWithNumberVariable(name) ? ("arg" + name) : name.toString();
}
protected boolean startsWithNumberVariable(Object variable) {
return "1234567890".contains(Srl.cut(variable.toString(), 1)); // sorry, simple logic
}
protected boolean containsSecureAnnotation(JavaPropertiesProperty property) {
final String comment = property.getComment();
return comment != null && Srl.containsIgnoreCase(comment, SECURE_ANNOTATION);
}
// -----------------------------------------------------
// Order Key
// ---------
protected List orderKeyList(Properties prop, final Map keyCommentMap) {
final List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy