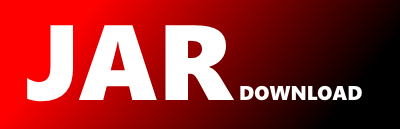
org.dbflute.helper.jprop.JavaPropertiesResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of dbflute-runtime Show documentation
Show all versions of dbflute-runtime Show documentation
The runtime library of DBFlute
/*
* Copyright 2014-2019 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND,
* either express or implied. See the License for the specific language
* governing permissions and limitations under the License.
*/
package org.dbflute.helper.jprop;
import java.util.List;
import java.util.Map;
import java.util.Properties;
import org.dbflute.util.DfCollectionUtil;
import org.dbflute.util.DfTypeUtil;
/**
* @author jflute
* @since 1.0.1 (2012/12/21 Friday)
*/
public class JavaPropertiesResult {
// ===================================================================================
// Attribute
// =========
protected final Properties _plainProp;
protected final List _propertyList; // merged list
protected final Map _propertyMap;
protected final List _propertyBasePointOnlyList;
protected final List _propertyExtendsOnlyList;
protected final List _duplicateKeyList;
protected final JavaPropertiesResult _extendsPropResult;
// ===================================================================================
// Constructor
// ===========
/**
* @param plainProp The plain properties as base point properties. (NotNull)
* @param propertyList The list of property merged with extends-properties. (NotNull)
* @param duplicateKeyList The list of duplicate property keys. (NotNull)
*/
public JavaPropertiesResult(Properties plainProp, List propertyList, List duplicateKeyList) {
this(plainProp, propertyList, duplicateKeyList, null);
}
/**
* @param plainProp The plain properties as base point properties. (NotNull)
* @param propertyList The list of property merged with extends-properties. (NotNull)
* @param duplicateKeyList The list of duplicate property keys. (NotNull)
* @param extendsPropResult The result for extends-properties. (NullAllowed: if null, no extends)
*/
public JavaPropertiesResult(Properties plainProp, List propertyList, List duplicateKeyList,
JavaPropertiesResult extendsPropResult) {
_plainProp = plainProp;
_propertyList = propertyList;
_propertyMap = DfCollectionUtil.newLinkedHashMapSized(propertyList.size());
_propertyBasePointOnlyList = DfCollectionUtil.newArrayList();
_propertyExtendsOnlyList = DfCollectionUtil.newArrayList();
for (JavaPropertiesProperty property : propertyList) {
_propertyMap.put(property.getPropertyKey(), property);
if (property.isExtends()) {
_propertyExtendsOnlyList.add(property);
} else {
_propertyBasePointOnlyList.add(property);
}
}
_duplicateKeyList = duplicateKeyList;
_extendsPropResult = extendsPropResult;
}
// ===================================================================================
// Basic Override
// ==============
@Override
public boolean equals(Object obj) {
if (obj == null || !(obj instanceof JavaPropertiesResult)) {
return false;
}
final JavaPropertiesResult another = (JavaPropertiesResult) obj;
return _propertyList.equals(another._propertyList);
}
@Override
public int hashCode() {
return _propertyList.hashCode();
}
@Override
public String toString() {
return DfTypeUtil.toClassTitle(this) + ":{" + _propertyMap.keySet() + "}";
}
// ===================================================================================
// Accessor
// ========
/**
* Get the plain properties as base point properties.
* @return The properties object of Java standard. (NotNull)
*/
public Properties getPlainProp() {
return _plainProp;
}
/**
* Get the property by the key.
* @param propertyKey The key string of property. (NotNull)
* @return The property object for Java properties. (NullAllowed: if null, means not found)
*/
public JavaPropertiesProperty getProperty(String propertyKey) {
return _propertyMap.get(propertyKey);
}
/**
* Get the property list merged with extends-properties.
* @return The list of property object. (NotNull)
*/
public List getPropertyList() {
return _propertyList;
}
/**
* Get the property map merged with extends-properties.
* @return The map of property object, the key of map is property key. (NotNull)
*/
public Map getPropertyMap() {
return _propertyMap;
}
public List getPropertyBasePointOnlyList() {
return _propertyBasePointOnlyList;
}
public List getPropertyExtendsOnlyList() {
return _propertyExtendsOnlyList;
}
/**
* Get the list of duplicate property keys.
* @return The list of duplicate property keys. (NotNull, EmptyAllowed)
*/
public List getDuplicateKeyList() {
return _duplicateKeyList;
}
public JavaPropertiesResult getExtendsPropResult() {
return _extendsPropResult;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy