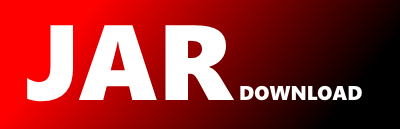
org.dbflute.s2dao.sqlhandler.TnCommandContextHandler Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of dbflute-runtime Show documentation
Show all versions of dbflute-runtime Show documentation
The runtime library of DBFlute
/*
* Copyright 2014-2019 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND,
* either express or implied. See the License for the specific language
* governing permissions and limitations under the License.
*/
package org.dbflute.s2dao.sqlhandler;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.util.ArrayList;
import java.util.List;
import javax.sql.DataSource;
import org.dbflute.jdbc.StatementFactory;
import org.dbflute.jdbc.ValueType;
import org.dbflute.s2dao.metadata.TnPropertyType;
import org.dbflute.twowaysql.context.CommandContext;
/**
* @author modified by jflute (originated in S2Dao)
*/
public class TnCommandContextHandler extends TnAbstractBasicSqlHandler {
// ===================================================================================
// Attribute
// =========
/** The context of command. (NotNull) */
protected final CommandContext _commandContext;
/** The list of bound property type in first scope. (NullAllowed) */
protected List _firstBoundPropTypeList;
/** The process title when SQL failure for update. (NullAllowed) */
protected String _updateSQLFailureProcessTitle;
// ===================================================================================
// Constructor
// ===========
public TnCommandContextHandler(DataSource dataSource, StatementFactory statementFactory, String sql, CommandContext commandContext) {
super(dataSource, statementFactory, sql);
assertObjectNotNull("commandContext", commandContext);
_commandContext = commandContext;
}
// ===================================================================================
// Execute
// =======
public int execute(Object[] args) {
final Connection conn = getConnection();
try {
return doExecute(conn, _commandContext);
} finally {
close(conn);
}
}
protected int doExecute(Connection conn, CommandContext ctx) {
logSql(ctx.getBindVariables(), getArgTypes(ctx.getBindVariables()));
final PreparedStatement ps = prepareStatement(conn);
int ret = -1;
try {
final Object[] bindVariables = ctx.getBindVariables();
final Class>[] bindVariableTypes = ctx.getBindVariableTypes();
if (hasBoundPropertyTypeList()) { // basically for queryUpdate()
final int index = bindFirstScope(conn, ps, bindVariables, bindVariableTypes);
bindSecondScope(conn, ps, bindVariables, bindVariableTypes, index);
} else {
bindArgs(conn, ps, bindVariables, bindVariableTypes);
}
ret = executeUpdate(ps);
} finally {
close(ps);
}
return ret;
}
protected boolean hasBoundPropertyTypeList() {
return _firstBoundPropTypeList != null && !_firstBoundPropTypeList.isEmpty();
}
// ===================================================================================
// Bind Scope
// ==========
protected int bindFirstScope(Connection conn, PreparedStatement ps, Object[] bindVariables, Class>[] bindVariableTypes) {
final List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy