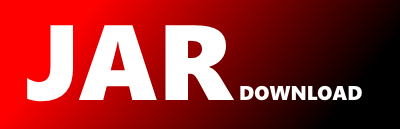
org.dbflute.outsidesql.executor.OutsideSqlAutoPagingExecutor Maven / Gradle / Ivy
/*
* Copyright 2014-2020 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND,
* either express or implied. See the License for the specific language
* governing permissions and limitations under the License.
*/
package org.dbflute.outsidesql.executor;
import org.dbflute.bhv.core.BehaviorCommandInvoker;
import org.dbflute.cbean.result.ListResultBean;
import org.dbflute.cbean.result.PagingResultBean;
import org.dbflute.dbway.DBDef;
import org.dbflute.jdbc.StatementConfig;
import org.dbflute.outsidesql.OutsideSqlOption;
import org.dbflute.outsidesql.factory.OutsideSqlExecutorFactory;
import org.dbflute.outsidesql.typed.AutoPagingHandlingPmb;
/**
* The auto-paging (cursor-skip) executor of outside-SQL.
* @param The type of behavior.
* @author jflute
*/
public class OutsideSqlAutoPagingExecutor extends AbstractOutsideSqlPagingExecutor {
// ===================================================================================
// Constructor
// ===========
public OutsideSqlAutoPagingExecutor(BehaviorCommandInvoker behaviorCommandInvoker, String tableDbName, DBDef currentDBDef,
OutsideSqlOption outsideSqlOption, OutsideSqlExecutorFactory outsideSqlExecutorFactory) {
super(behaviorCommandInvoker, tableDbName, currentDBDef, outsideSqlOption, outsideSqlExecutorFactory);
}
// ===================================================================================
// Select
// ======
/**
* Select page by the outside-SQL. {Typed Interface}
* (both count-select and paging-select are executed)
* You can call this method by only a typed parameter-bean
* which is related to its own (outside-SQL) path and entity-type.
*
* SimpleMemberPmb pmb = new SimpleMemberPmb();
* pmb.setMemberName_PrefixSearch("S");
* pmb.paging(20, 3); // 20 records per a page and current page number is 3
* PagingResultBean<SimpleMember> page
* = memberBhv.outsideSql().manualPaging().selectPage(pmb);
* int allRecordCount = page.getAllRecordCount();
* int allPageCount = page.getAllPageCount();
* boolean isExistPrePage = page.isExistPrePage();
* boolean isExistNextPage = page.isExistNextPage();
* ...
* for (SimpleMember member : page) {
* ... = member.get...();
* }
*
* The parameter-bean needs to extend SimplePagingBean.
* The way to generate it is following:
*
* -- !df:pmb extends SPB!
* -- !!Integer memberId!!
* -- !!...!!
*
* You can realize by pagingBean's isPaging() method on your 'Parameter Comment'.
* It returns false when it executes Count. And it returns true when it executes Paging.
*
* e.g. ManualPaging and MySQL
* /*IF pmb.isPaging()*/
* select member.MEMBER_ID
* , member.MEMBER_NAME
* , memberStatus.MEMBER_STATUS_NAME
* -- ELSE select count(*)
* /*END*/
* from MEMBER member
* /*IF pmb.isPaging()*/
* left outer join MEMBER_STATUS memberStatus
* on member.MEMBER_STATUS_CODE = memberStatus.MEMBER_STATUS_CODE
* /*END*/
* /*BEGIN*/
* where
* /*IF pmb.memberId != null*/
* member.MEMBER_ID = /*pmb.memberId*/'123'
* /*END*/
* /*IF pmb.memberName != null*/
* and member.MEMBER_NAME like /*pmb.memberName*/'Billy%'
* /*END*/
* /*END*/
* /*IF pmb.isPaging()*/
* order by member.UPDATE_DATETIME desc
* /*END*/
* /*IF pmb.isPaging()*/
* limit /*$pmb.pageStartIndex*/80, /*$pmb.fetchSize*/20
* /*END*/
*
* @param The type of entity.
* @param pmb The typed parameter-bean for auto-paging handling. (NotNull)
* @return The result bean of paging. (NotNull)
* @throws org.dbflute.exception.OutsideSqlNotFoundException When the outside-SQL is not found.
* @throws org.dbflute.exception.DangerousResultSizeException When the result size is over the specified safety size.
*/
public PagingResultBean selectPage(AutoPagingHandlingPmb pmb) {
if (pmb == null) {
String msg = "The argument 'pmb' (typed parameter-bean) should not be null.";
throw new IllegalArgumentException(msg);
}
return doSelectPage(pmb.getOutsideSqlPath(), pmb, pmb.getEntityType());
}
/**
* Select list with paging by the outside-SQL. {Typed Interface}
* (count-select is not executed, only paging-select)
* You can call this method by only a typed parameter-bean
* which is related to its own (outside-SQL) path and entity-type.
*
* SimpleMemberPmb pmb = new SimpleMemberPmb();
* pmb.setMemberName_PrefixSearch("S");
* pmb.paging(20, 3); // 20 records per a page and current page number is 3
* ListResultBean<SimpleMember> memberList
* = memberBhv.outsideSql().manualPaging().selectList(pmb);
* for (SimpleMember member : memberList) {
* ... = member.get...();
* }
*
* The parameter-bean needs to extend SimplePagingBean.
* The way to generate it is following:
*
* -- !df:pmb extends SPB!
* -- !!Integer memberId!!
* -- !!...!!
*
* You don't need to use pagingBean's isPaging() method on your 'Parameter Comment'.
*
* e.g. ManualPaging and MySQL
* select member.MEMBER_ID
* , member.MEMBER_NAME
* , memberStatus.MEMBER_STATUS_NAME
* from MEMBER member
* left outer join MEMBER_STATUS memberStatus
* on member.MEMBER_STATUS_CODE = memberStatus.MEMBER_STATUS_CODE
* /*BEGIN*/
* where
* /*IF pmb.memberId != null*/
* member.MEMBER_ID = /*pmb.memberId*/'123'
* /*END*/
* /*IF pmb.memberName != null*/
* and member.MEMBER_NAME like /*pmb.memberName*/'Billy%'
* /*END*/
* /*END*/
* order by member.UPDATE_DATETIME desc
* limit /*$pmb.pageStartIndex*/80, /*$pmb.fetchSize*/20
*
* @param The type of entity.
* @param pmb The typed parameter-bean for auto-paging handling. (NotNull)
* @return The result bean of paged list. (NotNull)
* @throws org.dbflute.exception.OutsideSqlNotFoundException When the outside-SQL is not found.
* @throws org.dbflute.exception.DangerousResultSizeException When the result size is over the specified safety size.
*/
public ListResultBean selectList(AutoPagingHandlingPmb pmb) {
return doSelectList(pmb.getOutsideSqlPath(), pmb, pmb.getEntityType());
}
// ===================================================================================
// Option
// ======
/**
* {@inheritDoc}
*/
@Override
public OutsideSqlAutoPagingExecutor removeBlockComment() {
return (OutsideSqlAutoPagingExecutor) super.removeBlockComment();
}
/**
* {@inheritDoc}
*/
@Override
public OutsideSqlAutoPagingExecutor removeLineComment() {
return (OutsideSqlAutoPagingExecutor) super.removeLineComment();
}
/**
* {@inheritDoc}
*/
@Override
public OutsideSqlAutoPagingExecutor formatSql() {
return (OutsideSqlAutoPagingExecutor) super.formatSql();
}
/**
* {@inheritDoc}
*/
@Override
public OutsideSqlAutoPagingExecutor configure(StatementConfig statementConfig) {
return (OutsideSqlAutoPagingExecutor) super.configure(statementConfig);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy