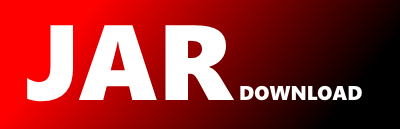
org.dbflute.outsidesql.executor.OutsideSqlTraditionalExecutor Maven / Gradle / Ivy
/*
* Copyright 2014-2020 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND,
* either express or implied. See the License for the specific language
* governing permissions and limitations under the License.
*/
package org.dbflute.outsidesql.executor;
import org.dbflute.bhv.exception.BehaviorExceptionThrower;
import org.dbflute.cbean.paging.PagingBean;
import org.dbflute.cbean.result.ListResultBean;
import org.dbflute.cbean.result.PagingResultBean;
import org.dbflute.jdbc.CursorHandler;
import org.dbflute.optional.OptionalEntity;
import org.dbflute.outsidesql.typed.AutoPagingHandlingPmb;
import org.dbflute.outsidesql.typed.ManualPagingHandlingPmb;
/**
* The traditional executor of outside-SQL.
* @param The type of behavior.
* @author jflute
* @since 1.1.0 (2014/10/13 Monday)
*/
public class OutsideSqlTraditionalExecutor {
// ===================================================================================
// Attribute
// =========
/** The basic executor. (NotNull) */
protected final OutsideSqlBasicExecutor _basicExecutor;
// ===================================================================================
// Constructor
// ===========
public OutsideSqlTraditionalExecutor(OutsideSqlBasicExecutor basicExecutor) {
_basicExecutor = basicExecutor;
}
// ===================================================================================
// Entity Select
// =============
/**
* Select entity by the outside-SQL. {FreeStyle Interface}
* This method can accept each element: path, parameter-bean(Object type), entity-type.
*
* String path = MemberBhv.PATH_selectSimpleMember;
* SimpleMemberPmb pmb = new SimpleMemberPmb();
* pmb.setMemberId(3);
* Class<SimpleMember> entityType = SimpleMember.class;
* SimpleMember member
* = memberBhv.outsideSql().entityHandling().selectEntity(path, pmb, entityType);
* if (member != null) {
* ... = member.get...();
* } else {
* ...
* }
*
* @param The type of entity.
* @param path The path of SQL file. (NotNull)
* @param pmb The object as parameter-bean. Allowed types are Bean object and Map object. (NullAllowed)
* @param entityType The type of entity. (NotNull)
* @return The selected entity. (NullAllowed)
* @throws org.dbflute.exception.OutsideSqlNotFoundException When the outside-SQL is not found.
* @throws org.dbflute.exception.EntityDuplicatedException When the entity is duplicated.
*/
public OptionalEntity selectEntity(String path, Object pmb, Class entityType) {
return OptionalEntity.ofNullable(_basicExecutor.entityHandling().selectEntity(path, pmb, entityType), () -> {
createBhvExThrower().throwSelectEntityAlreadyDeletedException(pmb);
});
}
// ===================================================================================
// List Select
// ===========
/**
* Select the list of the entity by the outsideSql. {FreeStyle Interface}
* This method can accept each element: path, parameter-bean(Object type), entity-type.
*
* String path = MemberBhv.PATH_selectSimpleMember;
* SimpleMemberPmb pmb = new SimpleMemberPmb();
* pmb.setMemberName_PrefixSearch("S");
* Class<SimpleMember> entityType = SimpleMember.class;
* ListResultBean<SimpleMember> memberList
* = memberBhv.outsideSql().selectList(path, pmb, entityType);
* for (SimpleMember member : memberList) {
* ... = member.get...();
* }
*
* It needs to use customize-entity and parameter-bean.
* The way to generate them is following:
*
* -- #df:entity#
* -- !df:pmb!
* -- !!Integer memberId!!
* -- !!String memberName!!
* -- !!...!!
*
* @param The type of entity for element.
* @param path The path of SQL file. (NotNull)
* @param pmb The object as parameter-bean. Allowed types are Bean object and Map object. (NullAllowed)
* @param entityType The element type of entity. (NotNull)
* @return The result bean of selected list. (NotNull)
* @throws org.dbflute.exception.OutsideSqlNotFoundException When the outsideSql is not found.
* @throws org.dbflute.exception.DangerousResultSizeException When the result size is over the specified safety size.
*/
public ListResultBean selectList(String path, Object pmb, Class entityType) {
return _basicExecutor.selectList(path, pmb, entityType);
}
// ===================================================================================
// Paging Select
// =============
/**
* Select page by the outside-SQL. {FreeStyle Interface}
* (both count-select and paging-select are executed)
* This method can accept each element: path, parameter-bean(Object type), entity-type.
*
* String path = MemberBhv.PATH_selectSimpleMember;
* SimpleMemberPmb pmb = new SimpleMemberPmb();
* pmb.setMemberName_PrefixSearch("S");
* pmb.paging(20, 3); // 20 records per a page and current page number is 3
* Class<SimpleMember> entityType = SimpleMember.class;
* PagingResultBean<SimpleMember> page
* = memberBhv.outsideSql().manualPaging().selectPage(path, pmb, entityType);
* int allRecordCount = page.getAllRecordCount();
* int allPageCount = page.getAllPageCount();
* boolean isExistPrePage = page.isExistPrePage();
* boolean isExistNextPage = page.isExistNextPage();
* ...
* for (SimpleMember member : page) {
* ... = member.get...();
* }
*
* The parameter-bean needs to extend SimplePagingBean.
* The way to generate it is following:
*
* -- !df:pmb extends Paging!
* -- !!Integer memberId!!
* -- !!...!!
*
* You can realize by pagingBean's isPaging() method on your 'Parameter Comment'.
* It returns false when it executes Count. And it returns true when it executes Paging.
*
* e.g. ManualPaging and MySQL
* /*IF pmb.isPaging()*/
* select member.MEMBER_ID
* , member.MEMBER_NAME
* , memberStatus.MEMBER_STATUS_NAME
* -- ELSE select count(*)
* /*END*/
* from MEMBER member
* /*IF pmb.isPaging()*/
* left outer join MEMBER_STATUS memberStatus
* on member.MEMBER_STATUS_CODE = memberStatus.MEMBER_STATUS_CODE
* /*END*/
* /*BEGIN*/
* where
* /*IF pmb.memberId != null*/
* member.MEMBER_ID = /*pmb.memberId*/'123'
* /*END*/
* /*IF pmb.memberName != null*/
* and member.MEMBER_NAME like /*pmb.memberName*/'Billy%'
* /*END*/
* /*END*/
* /*IF pmb.isPaging()*/
* order by member.UPDATE_DATETIME desc
* /*END*/
* /*IF pmb.isPaging()*/
* limit /*$pmb.pageStartIndex*/80, /*$pmb.fetchSize*/20
* /*END*/
*
* @param The type of entity.
* @param path The path of SQL that executes count and paging. (NotNull)
* @param pmb The bean of paging parameter. (NotNull)
* @param entityType The type of result entity. (NotNull)
* @return The result bean of paging. (NotNull)
* @throws org.dbflute.exception.OutsideSqlNotFoundException When the outside-SQL is not found.
* @throws org.dbflute.exception.DangerousResultSizeException When the result size is over the specified safety size.
*/
public PagingResultBean selectPage(String path, PagingBean pmb, Class entityType) {
if (pmb instanceof ManualPagingHandlingPmb) {
return _basicExecutor.manualPaging().selectPage(path, pmb, entityType);
} else if (pmb instanceof AutoPagingHandlingPmb) {
return _basicExecutor.autoPaging().selectPage(path, pmb, entityType);
} else {
String msg = "Unknown paging handling parameter-bean: " + pmb;
throw new IllegalStateException(msg);
}
}
/**
* Select list with paging by the outside-SQL. {FreeStyle Interface}
* (count-select is not executed, only paging-select)
* This method can accept each element: path, parameter-bean(Object type), entity-type.
*
* String path = MemberBhv.PATH_selectSimpleMember;
* SimpleMemberPmb pmb = new SimpleMemberPmb();
* pmb.setMemberName_PrefixSearch("S");
* pmb.paging(20, 3); // 20 records per a page and current page number is 3
* Class<SimpleMember> entityType = SimpleMember.class;
* ListResultBean<SimpleMember> memberList
* = memberBhv.outsideSql().manualPaging().selectList(path, pmb, entityType);
* for (SimpleMember member : memberList) {
* ... = member.get...();
* }
*
* The parameter-bean needs to extend SimplePagingBean.
* The way to generate it is following:
*
* -- !df:pmb extends Paging!
* -- !!Integer memberId!!
* -- !!...!!
*
* You don't need to use pagingBean's isPaging() method on your 'Parameter Comment'.
*
* e.g. ManualPaging and MySQL
* select member.MEMBER_ID
* , member.MEMBER_NAME
* , memberStatus.MEMBER_STATUS_NAME
* from MEMBER member
* left outer join MEMBER_STATUS memberStatus
* on member.MEMBER_STATUS_CODE = memberStatus.MEMBER_STATUS_CODE
* /*BEGIN*/
* where
* /*IF pmb.memberId != null*/
* member.MEMBER_ID = /*pmb.memberId*/'123'
* /*END*/
* /*IF pmb.memberName != null*/
* and member.MEMBER_NAME like /*pmb.memberName*/'Billy%'
* /*END*/
* /*END*/
* order by member.UPDATE_DATETIME desc
* limit /*pmb.pageStartIndex*/80, /*pmb.fetchSize*/20
*
* @param The type of entity.
* @param path The path of SQL that executes count and paging. (NotNull)
* @param pmb The bean of paging parameter. (NotNull)
* @param entityType The type of result entity. (NotNull)
* @return The result bean of paged list. (NotNull)
* @throws org.dbflute.exception.OutsideSqlNotFoundException When the outside-SQL is not found.
* @throws org.dbflute.exception.DangerousResultSizeException When the result size is over the specified safety size.
*/
public ListResultBean selectPagedListOnly(String path, PagingBean pmb, Class entityType) {
if (pmb instanceof ManualPagingHandlingPmb) {
return _basicExecutor.manualPaging().selectPage(path, pmb, entityType);
} else if (pmb instanceof AutoPagingHandlingPmb) {
return _basicExecutor.autoPaging().selectPage(path, pmb, entityType);
} else {
String msg = "Unknown paging handling parameter-bean: " + pmb;
throw new IllegalStateException(msg);
}
}
// ===================================================================================
// Cursor Select
// =============
/**
* Select the cursor of the entity by outside-SQL. {FreeStyle Interface}
* This method can accept each element: path, parameter-bean(Object type), cursor-handler.
*
* String path = MemberBhv.PATH_selectSimpleMember;
* SimpleMemberPmb pmb = new SimpleMemberPmb();
* pmb.setMemberName_PrefixSearch("S");
* memberBhv.outsideSql().cursorHandling()
* .selectCursor(path, pmb, new PurchaseSummaryMemberCursorHandler() {
* protected Object fetchCursor(PurchaseSummaryMemberCursor cursor) throws SQLException {
* while (cursor.next()) {
* Integer memberId = cursor.getMemberId();
* String memberName = cursor.getMemberName();
* ...
* }
* return null;
* }
* });
*
* It needs to use type-safe-cursor instead of customize-entity.
* The way to generate it is following:
*
* -- #df:entity#
* -- +cursor+
*
* @param path The path of SQL file. (NotNull)
* @param pmb The object as parameter-bean. Allowed types are Bean object and Map object. (NullAllowed)
* @param handler The handler of cursor called back with result set. (NotNull)
* @return The result object that the cursor handler returns. (NullAllowed)
* @throws org.dbflute.exception.OutsideSqlNotFoundException When the outside-SQL is not found.
*/
public Object selectCursor(String path, Object pmb, CursorHandler handler) {
return _basicExecutor.cursorHandling().selectCursor(path, pmb, handler);
}
// ===================================================================================
// Execute
// =======
/**
* Execute the outsideSql. (insert, update, delete, etc...) {FreeStyle Interface}
* This method can accept each element: path, parameter-bean(Object type).
*
* String path = MemberBhv.PATH_selectSimpleMember;
* SimpleMemberPmb pmb = new SimpleMemberPmb();
* pmb.setMemberId(3);
* int count = memberBhv.outsideSql().execute(path, pmb);
*
* @param path The path of SQL file. (NotNull)
* @param pmb The parameter-bean. Allowed types are Bean object and Map object. (NullAllowed)
* @return The count of execution.
* @throws org.dbflute.exception.OutsideSqlNotFoundException When the outsideSql is not found.
*/
public int execute(String path, Object pmb) {
return _basicExecutor.execute(path, pmb);
}
// ===================================================================================
// Exception Helper
// ================
protected BehaviorExceptionThrower createBhvExThrower() {
return _basicExecutor.createBhvExThrower();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy