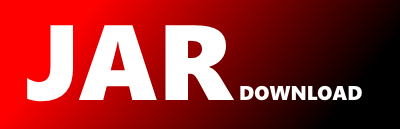
org.dbflute.cbean.chelper.HpColQyOperand Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of dbflute-runtime Show documentation
Show all versions of dbflute-runtime Show documentation
The runtime library of DBFlute
/*
* Copyright 2014-2021 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND,
* either express or implied. See the License for the specific language
* governing permissions and limitations under the License.
*/
package org.dbflute.cbean.chelper;
import org.dbflute.cbean.ConditionBean;
import org.dbflute.cbean.ckey.ConditionKey;
import org.dbflute.cbean.dream.ColumnCalculator;
import org.dbflute.cbean.scoping.SpecifyQuery;
/**
* @param The type of condition-bean.
* @author jflute
*/
public class HpColQyOperand {
// ===================================================================================
// Attribute
// =========
protected final HpColQyHandler _handler;
// ===================================================================================
// Constructor
// ===========
public HpColQyOperand(HpColQyHandler handler) {
_handler = handler;
}
// ===================================================================================
// Comparison
// ==========
/**
* Equal(=).
*
* // where FOO = BAR
* cb.columnQuery(colCB -> {
* colCB.specify().columnFoo(); // left column
* }).equal(colCB -> {
* colCB.specify().columnBar(); // right column
* }); // you can calculate for right column like '}).plus(3);'
*
* @param colCBLambda The callback for specify-query of right column. (NotNull)
* @return The calculator for right column. (NotNull)
*/
public ColumnCalculator equal(SpecifyQuery colCBLambda) {
return _handler.handle(colCBLambda, ConditionKey.CK_EQUAL.getOperand());
}
/**
* NotEqual(<>).
*
* // where FOO <> BAR
* cb.columnQuery(colCB -> {
* colCB.specify().columnFoo(); // left column
* }).notEqual(colCB -> {
* colCB.specify().columnBar(); // right column
* }); // you can calculate for right column like '}).plus(3);'
*
* @param colCBLambda The callback for specify-query of right column. (NotNull)
* @return The calculator for right column. (NotNull)
*/
public ColumnCalculator notEqual(SpecifyQuery colCBLambda) {
return _handler.handle(colCBLambda, ConditionKey.CK_NOT_EQUAL_STANDARD.getOperand());
}
/**
* GreaterThan(>).
*
* // where FOO > BAR
* cb.columnQuery(colCB -> {
* colCB.specify().columnFoo(); // left column
* }).greaterThan(colCB -> {
* colCB.specify().columnBar(); // right column
* }); // you can calculate for right column like '}).plus(3);'
*
* @param colCBLambda The callback for specify-query of right column. (NotNull)
* @return The calculator for right column. (NotNull)
*/
public ColumnCalculator greaterThan(SpecifyQuery colCBLambda) {
return _handler.handle(colCBLambda, ConditionKey.CK_GREATER_THAN.getOperand());
}
/**
* LessThan(<).
*
* // where FOO < BAR
* cb.columnQuery(colCB -> {
* colCB.specify().columnFoo(); // left column
* }).lessThan(colCB -> {
* colCB.specify().columnBar(); // right column
* }); // you can calculate for right column like '}).plus(3);'
*
* @param colCBLambda The callback for specify-query of right column. (NotNull)
* @return The calculator for right column. (NotNull)
*/
public ColumnCalculator lessThan(SpecifyQuery colCBLambda) {
return _handler.handle(colCBLambda, ConditionKey.CK_LESS_THAN.getOperand());
}
/**
* GreaterEqual(>=).
*
* // where FOO >= BAR
* cb.columnQuery(colCB -> {
* colCB.specify().columnFoo(); // left column
* }).greaterEqual(colCB -> {
* colCB.specify().columnBar(); // right column
* }); // you can calculate for right column like '}).plus(3);'
*
* @param colCBLambda The callback for specify-query of right column. (NotNull)
* @return The calculator for right column. (NotNull)
*/
public ColumnCalculator greaterEqual(SpecifyQuery colCBLambda) {
return _handler.handle(colCBLambda, ConditionKey.CK_GREATER_EQUAL.getOperand());
}
/**
* LessThan(<=).
*
* // where FOO <= BAR
* cb.columnQuery(colCB -> {
* colCB.specify().columnFoo(); // left column
* }).lessEqual(colCB -> {
* colCB.specify().columnBar(); // right column
* }); // you can calculate for right column like '}).plus(3);'
*
* @param colCBLambda The callback for specify-query of right column. (NotNull)
* @return The calculator for right column. (NotNull)
*/
public ColumnCalculator lessEqual(SpecifyQuery colCBLambda) {
return _handler.handle(colCBLambda, ConditionKey.CK_LESS_EQUAL.getOperand());
}
// ===================================================================================
// DBMS Dependency
// ===============
public static class HpExtendedColQyOperandMySql extends HpColQyOperand {
public HpExtendedColQyOperandMySql(HpColQyHandler handler) {
super(handler);
}
/**
* BitAnd(&).
* @param rightSpecifyQuery The specify-query for right column. (NotNull)
* @return The calculator for right column. (NotNull)
*/
public ColumnCalculator bitAnd(SpecifyQuery rightSpecifyQuery) {
return _handler.handle(rightSpecifyQuery, "&");
}
/**
* BitOr(|).
* @param rightSpecifyQuery The specify-query for right column. (NotNull)
* @return The calculator for right column. (NotNull)
*/
public ColumnCalculator bitOr(SpecifyQuery rightSpecifyQuery) {
return _handler.handle(rightSpecifyQuery, "|");
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy