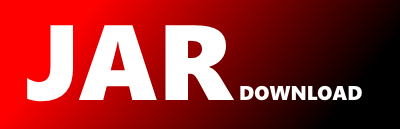
org.dbflute.cbean.chelper.HpSLCFunction Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of dbflute-runtime Show documentation
Show all versions of dbflute-runtime Show documentation
The runtime library of DBFlute
/*
* Copyright 2014-2021 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND,
* either express or implied. See the License for the specific language
* governing permissions and limitations under the License.
*/
package org.dbflute.cbean.chelper;
import org.dbflute.cbean.ConditionBean;
import org.dbflute.cbean.coption.FunctionFilterOptionCall;
import org.dbflute.cbean.coption.ScalarConditionOption;
import org.dbflute.cbean.scoping.SubQuery;
/**
* The function for ScalarCondition (the old name: ScalarSubQuery).
* @param The type of condition-bean.
* @author jflute
*/
public class HpSLCFunction {
// ===================================================================================
// Attribute
// =========
protected final HpSLCSetupper _setupper;
// ===================================================================================
// Constructor
// ===========
public HpSLCFunction(HpSLCSetupper setupper) {
_setupper = setupper;
}
// ===================================================================================
// Function
// ========
// -----------------------------------------------------
// Maximum
// -------
/**
* Set up the sub query of myself for the scalar 'max'.
*
* cb.query().scalar_Equal().max(purchaseCB -> {
* purchaseCB.specify().columnPurchasePrice(); // *Point!
* purchaseCB.query().setPaymentCompleteFlg_Equal_True();
* });
*
* @param scalarCBLambda The callback for sub-query of myself. (NotNull)
* @return The decorator of ScalarCondition. e.g. you can use partition-by. (NotNull)
*/
public HpSLCDecorator max(SubQuery scalarCBLambda) {
return doMax(scalarCBLambda, null);
}
/**
* Set up the sub query of myself for the scalar 'max'.
*
* cb.query().scalar_Equal().max(purchaseCB -> {
* purchaseCB.specify().columnPurchasePrice(); // *Point!
* purchaseCB.query().setPaymentCompleteFlg_Equal_True();
* }, op -> op.coalesce(0));
*
* @param scalarCBLambda The callback for sub-query of myself. (NotNull)
* @param opLambda The callback for option of scalar. (NotNull)
* @return The decorator of ScalarCondition. e.g. you can use partition-by. (NotNull)
*/
public HpSLCDecorator max(SubQuery scalarCBLambda, FunctionFilterOptionCall opLambda) {
return doMax(scalarCBLambda, prepareOption(opLambda));
}
protected HpSLCDecorator doMax(SubQuery scalarCBLambda, ScalarConditionOption option) {
final HpSLCCustomized customized = createCustomized();
setupScalarCondition("max", scalarCBLambda, customized, option);
return createDecorator(customized);
}
// -----------------------------------------------------
// Minimum
// -------
/**
* Set up the sub query of myself for the scalar 'min'.
*
* cb.query().scalar_Equal().min(purchaseCB -> {
* purchaseCB.specify().columnPurchasePrice(); // *Point!
* purchaseCB.query().setPaymentCompleteFlg_Equal_True();
* });
*
* @param scalarCBLambda The callback for sub-query of myself. (NotNull)
* @return The decorator of ScalarCondition. e.g. you can use partition-by. (NotNull)
*/
public HpSLCDecorator min(SubQuery scalarCBLambda) {
return doMin(scalarCBLambda, null);
}
/**
* Set up the sub query of myself for the scalar 'min'.
*
* cb.query().scalar_Equal().min(purchaseCB -> {
* purchaseCB.specify().columnPurchasePrice(); // *Point!
* purchaseCB.query().setPaymentCompleteFlg_Equal_True();
* }, op -> op.coalesce(0));
*
* @param scalarCBLambda The callback for sub-query of myself. (NotNull)
* @param opLambda The callback for option of scalar. (NotNull)
* @return The decorator of ScalarCondition. e.g. you can use partition-by. (NotNull)
*/
public HpSLCDecorator min(SubQuery scalarCBLambda, FunctionFilterOptionCall opLambda) {
return doMin(scalarCBLambda, prepareOption(opLambda));
}
protected HpSLCDecorator doMin(SubQuery scalarCBLambda, ScalarConditionOption option) {
final HpSLCCustomized customized = createCustomized();
setupScalarCondition("min", scalarCBLambda, customized, option);
return createDecorator(customized);
}
// -----------------------------------------------------
// Summary
// -------
/**
* Set up the sub query of myself for the scalar 'sum'.
*
* cb.query().scalar_Equal().sum(purchaseCB -> {
* purchaseCB.specify().columnPurchasePrice(); // *Point!
* purchaseCB.query().setPaymentCompleteFlg_Equal_True();
* });
*
* @param scalarCBLambda The callback for sub-query of myself. (NotNull)
* @return The decorator of ScalarCondition. e.g. you can use partition-by. (NotNull)
*/
public HpSLCDecorator sum(SubQuery scalarCBLambda) {
return doSum(scalarCBLambda, null);
}
/**
* Set up the sub query of myself for the scalar 'sum'.
*
* cb.query().scalar_Equal().sum(purchaseCB -> {
* purchaseCB.specify().columnPurchasePrice(); // *Point!
* purchaseCB.query().setPaymentCompleteFlg_Equal_True();
* }, op -> op.coalesce(0));
*
* @param scalarCBLambda The callback for sub-query of myself. (NotNull)
* @param opLambda The callback for option of scalar. (NotNull)
* @return The decorator of ScalarCondition. e.g. you can use partition-by. (NotNull)
*/
public HpSLCDecorator sum(SubQuery scalarCBLambda, FunctionFilterOptionCall opLambda) {
return doSum(scalarCBLambda, prepareOption(opLambda));
}
protected HpSLCDecorator doSum(SubQuery scalarCBLambda, ScalarConditionOption option) {
final HpSLCCustomized customized = createCustomized();
setupScalarCondition("sum", scalarCBLambda, customized, option);
return createDecorator(customized);
}
// -----------------------------------------------------
// Average
// -------
/**
* Set up the sub query of myself for the scalar 'avg'.
*
* cb.query().scalar_Equal().avg(purchaseCB -> {
* purchaseCB.specify().columnPurchasePrice(); // *Point!
* purchaseCB.query().setPaymentCompleteFlg_Equal_True();
* });
*
* @param scalarCBLambda The callback for sub-query of myself. (NotNull)
* @return The decorator of ScalarCondition. e.g. you can use partition-by. (NotNull)
*/
public HpSLCDecorator avg(SubQuery scalarCBLambda) {
return doAvg(scalarCBLambda, null);
}
/**
* Set up the sub query of myself for the scalar 'avg'.
*
* cb.query().scalar_Equal().avg(purchaseCB -> {
* purchaseCB.specify().columnPurchasePrice(); // *Point!
* purchaseCB.query().setPaymentCompleteFlg_Equal_True();
* }, op -> op.coalesce(0));
*
* @param scalarCBLambda The callback for sub-query of myself. (NotNull)
* @param opLambda The callback for option of scalar. (NotNull)
* @return The decorator of ScalarCondition. e.g. you can use partition-by. (NotNull)
*/
public HpSLCDecorator avg(SubQuery scalarCBLambda, FunctionFilterOptionCall opLambda) {
return doAvg(scalarCBLambda, prepareOption(opLambda));
}
protected HpSLCDecorator doAvg(SubQuery scalarCBLambda, ScalarConditionOption option) {
final HpSLCCustomized customized = createCustomized();
setupScalarCondition("avg", scalarCBLambda, customized, option);
return createDecorator(customized);
}
// ===================================================================================
// Set up
// ======
protected void setupScalarCondition(String function, SubQuery scalarCBLambda, HpSLCCustomized customized,
ScalarConditionOption option) {
assertSubQuery(scalarCBLambda);
_setupper.setup(function, scalarCBLambda, customized, option);
}
// ===================================================================================
// Assist Helper
// =============
protected HpSLCCustomized createCustomized() {
return new HpSLCCustomized();
}
protected HpSLCDecorator createDecorator(HpSLCCustomized option) {
return new HpSLCDecorator(option);
}
protected ScalarConditionOption prepareOption(FunctionFilterOptionCall opLambda) {
assertObjectNotNull("opLambda", opLambda);
final ScalarConditionOption option = createScalarConditionOption();
opLambda.callback(option);
assertScalarConditionOption(option);
return option;
}
protected ScalarConditionOption createScalarConditionOption() {
return newScalarConditionOption();
}
protected ScalarConditionOption newScalarConditionOption() {
return new ScalarConditionOption();
}
protected void assertSubQuery(SubQuery> scalarCBLambda) {
if (scalarCBLambda == null) {
String msg = "The argument 'scalarCBLambda' for ScalarCondition should not be null.";
throw new IllegalArgumentException(msg);
}
}
protected void assertScalarConditionOption(ScalarConditionOption option) {
if (option == null) {
String msg = "The argument 'option' for (Myself)ScalarCondition should not be null.";
throw new IllegalArgumentException(msg);
}
}
protected void assertObjectNotNull(String variableName, Object value) {
if (variableName == null) {
String msg = "The value should not be null: variableName=null value=" + value;
throw new IllegalArgumentException(msg);
}
if (value == null) {
String msg = "The value should not be null: variableName=" + variableName;
throw new IllegalArgumentException(msg);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy