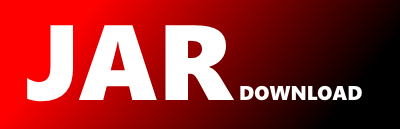
org.dbflute.cbean.chelper.HpQDRFunction Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of dbflute-runtime Show documentation
Show all versions of dbflute-runtime Show documentation
The runtime library of DBFlute
/*
* Copyright 2014-2023 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND,
* either express or implied. See the License for the specific language
* governing permissions and limitations under the License.
*/
package org.dbflute.cbean.chelper;
import org.dbflute.cbean.ConditionBean;
import org.dbflute.cbean.coption.DerivedReferrerOption;
import org.dbflute.cbean.coption.DerivedReferrerOptionFactory;
import org.dbflute.cbean.coption.FunctionFilterOptionCall;
import org.dbflute.cbean.scoping.SubQuery;
/**
* The function of (Query)DerivedReferrer.
* @param The type of condition-bean.
* @author jflute
*/
public class HpQDRFunction {
// ===================================================================================
// Attribute
// =========
protected final HpQDRSetupper _setupper;
protected final DerivedReferrerOptionFactory _derivedReferrerOptionFactory;
// ===================================================================================
// Constructor
// ===========
public HpQDRFunction(HpQDRSetupper setupper, DerivedReferrerOptionFactory derivedReferrerOptionFactory) {
_setupper = setupper;
_derivedReferrerOptionFactory = derivedReferrerOptionFactory;
}
// ===================================================================================
// Function
// ========
/**
* Set up the sub query of referrer for the scalar 'count'.
*
* cb.query().derivedPurchaseList().count(purchaseCB -> {
* purchaseCB.specify().columnPurchaseId(); // *Point
* purchaseCB.query().setPaymentCompleteFlg_Equal_True();
* }).greaterEqual(123); // *Don't forget the parameter
*
* @param derivedCBLambda The callback for sub-query of referrer. (NotNull)
* @return The parameter for comparing with scalar. (NotNull)
*/
public HpQDRParameter count(SubQuery derivedCBLambda) {
return doCount(derivedCBLambda, null);
}
/**
* An overload method for count(). So refer to the method's java-doc about basic info.
*
* cb.query().derivedPurchaseList().count(purchaseCB -> {
* purchaseCB.specify().columnPurchasePrice(); // *Point
* purchaseCB.query().setPaymentCompleteFlg_Equal_True();
* }).greaterEqual(123, op -> op.coalesce(0));
*
* @param derivedCBLambda The callback for sub-query of referrer. (NotNull)
* @param opLambda The callback for option of DerivedReferrer. For example, you can use a coalesce function. (NotNull)
* @return The parameter for comparing with scalar. (NotNull)
*/
public HpQDRParameter count(SubQuery derivedCBLambda, FunctionFilterOptionCall opLambda) {
assertDerivedReferrerOption(opLambda);
final DerivedReferrerOption option = createDerivedReferrerOption();
opLambda.callback(option);
return doCount(derivedCBLambda, option);
}
protected HpQDRParameter doCount(SubQuery subQuery, DerivedReferrerOption option) {
assertSubQuery(subQuery);
return createQDRParameter("count", subQuery, option);
}
/**
* Set up the sub query of referrer for the scalar 'count(with distinct)'.
*
* cb.query().derivedPurchaseList().countDistinct(purchaseCB -> {
* purchaseCB.specify().columnPurchasePrice(); // *Point
* purchaseCB.query().setPaymentCompleteFlg_Equal_True();
* }).greaterEqual(123); // *Don't forget the parameter
*
* @param derivedCBLambda The callback for sub-query of referrer. (NotNull)
* @return The parameter for comparing with scalar. (NotNull)
*/
public HpQDRParameter countDistinct(SubQuery derivedCBLambda) {
return doCountDistinct(derivedCBLambda, null);
}
/**
* An overload method for countDistinct(). So refer to the method's java-doc about basic info.
*
* cb.query().derivedPurchaseList().countDistinct(purchaseCB -> {
* purchaseCB.specify().columnPurchasePrice(); // *Point
* purchaseCB.query().setPaymentCompleteFlg_Equal_True();
* }).greaterEqual(123, op -> op.coalesce(0));
*
* @param derivedCBLambda The callback for sub-query of referrer. (NotNull)
* @param opLambda The callback for option of DerivedReferrer. For example, you can use a coalesce function. (NotNull)
* @return The parameter for comparing with scalar. (NotNull)
*/
public HpQDRParameter countDistinct(SubQuery derivedCBLambda,
FunctionFilterOptionCall opLambda) {
assertDerivedReferrerOption(opLambda);
final DerivedReferrerOption option = createDerivedReferrerOption();
opLambda.callback(option);
return doCountDistinct(derivedCBLambda, option);
}
protected HpQDRParameter doCountDistinct(SubQuery subQuery, DerivedReferrerOption option) {
assertSubQuery(subQuery);
return createQDRParameter("count(distinct", subQuery, option);
}
/**
* Set up the sub query of referrer for the scalar 'max'.
*
* cb.query().derivedPurchaseList().max(purchaseCB -> {
* purchaseCB.specify().columnPurchasePrice(); // *Point
* purchaseCB.query().setPaymentCompleteFlg_Equal_True();
* }).greaterEqual(123); // *Don't forget the parameter
*
* @param derivedCBLambda The callback for sub-query of referrer. (NotNull)
* @return The parameter for comparing with scalar. (NotNull)
*/
public HpQDRParameter max(SubQuery derivedCBLambda) {
return doMax(derivedCBLambda, null);
}
/**
* An overload method for max(). So refer to the method's java-doc about basic info.
*
* cb.query().derivedPurchaseList().max(purchaseCB -> {
* purchaseCB.specify().columnPurchasePrice(); // *Point
* purchaseCB.query().setPaymentCompleteFlg_Equal_True();
* }).greaterEqual(123, op -> op.coalesce(0));
*
* @param derivedCBLambda The callback for sub-query of referrer. (NotNull)
* @param opLambda The callback for option of DerivedReferrer. For example, you can use a coalesce function. (NotNull)
* @return The parameter for comparing with scalar. (NotNull)
*/
public HpQDRParameter max(SubQuery derivedCBLambda, FunctionFilterOptionCall opLambda) {
assertDerivedReferrerOption(opLambda);
final DerivedReferrerOption option = createDerivedReferrerOption();
opLambda.callback(option);
return doMax(derivedCBLambda, option);
}
protected HpQDRParameter doMax(SubQuery subQuery, DerivedReferrerOption option) {
assertSubQuery(subQuery);
return createQDRParameter("max", subQuery, option);
}
/**
* Set up the sub query of referrer for the scalar 'min'.
*
* cb.query().derivedPurchaseList().min(purchaseCB -> {
* purchaseCB.specify().columnPurchasePrice(); // *Point
* purchaseCB.query().setPaymentCompleteFlg_Equal_True();
* }).greaterEqual(123); // *Don't forget the parameter
*
* @param derivedCBLambda The callback for sub-query of referrer. (NotNull)
* @return The parameter for comparing with scalar. (NotNull)
*/
public HpQDRParameter min(SubQuery derivedCBLambda) {
return doMin(derivedCBLambda, null);
}
/**
* An overload method for min(). So refer to the method's java-doc about basic info.
*
* cb.query().derivedPurchaseList().min(purchaseCB -> {
* purchaseCB.specify().columnPurchasePrice(); // *Point
* purchaseCB.query().setPaymentCompleteFlg_Equal_True();
* }).greaterEqual(123, op -> op.coalesce(0));
*
* @param derivedCBLambda The callback for sub-query of referrer. (NotNull)
* @param opLambda The callback for option of DerivedReferrer. For example, you can use a coalesce function. (NotNull)
* @return The parameter for comparing with scalar. (NotNull)
*/
public HpQDRParameter min(SubQuery derivedCBLambda, FunctionFilterOptionCall opLambda) {
assertDerivedReferrerOption(opLambda);
final DerivedReferrerOption option = createDerivedReferrerOption();
opLambda.callback(option);
return doMin(derivedCBLambda, option);
}
protected HpQDRParameter doMin(SubQuery subQuery, DerivedReferrerOption option) {
assertSubQuery(subQuery);
return createQDRParameter("min", subQuery, option);
}
/**
* Set up the sub query of referrer for the scalar 'sum'.
*
* cb.query().derivedPurchaseList().sum(purchaseCB -> {
* purchaseCB.specify().columnPurchasePrice(); // *Point
* purchaseCB.query().setPaymentCompleteFlg_Equal_True();
* }).greaterEqual(123); // *Don't forget the parameter
*
* @param derivedCBLambda The callback for sub-query of referrer. (NotNull)
* @return The parameter for comparing with scalar. (NotNull)
*/
public HpQDRParameter sum(SubQuery derivedCBLambda) {
return doSum(derivedCBLambda, null);
}
/**
* An overload method for sum(). So refer to the method's java-doc about basic info.
*
* cb.query().derivedPurchaseList().sum(purchaseCB -> {
* purchaseCB.specify().columnPurchasePrice(); // *Point
* purchaseCB.query().setPaymentCompleteFlg_Equal_True();
* }).greaterEqual(123, op -> op.coalesce(0));
*
* @param derivedCBLambda The callback for sub-query of referrer. (NotNull)
* @param opLambda The callback for option of DerivedReferrer. For example, you can use a coalesce function. (NotNull)
* @return The parameter for comparing with scalar. (NotNull)
*/
public HpQDRParameter sum(SubQuery derivedCBLambda, FunctionFilterOptionCall opLambda) {
assertDerivedReferrerOption(opLambda);
final DerivedReferrerOption option = createDerivedReferrerOption();
opLambda.callback(option);
return doSum(derivedCBLambda, option);
}
protected HpQDRParameter doSum(SubQuery subQuery, DerivedReferrerOption option) {
assertSubQuery(subQuery);
return createQDRParameter("sum", subQuery, option);
}
/**
* Set up the sub query of referrer for the scalar 'avg'.
*
* cb.query().derivedPurchaseList().avg(purchaseCB -> {
* purchaseCB.specify().columnPurchasePrice(); // *Point
* purchaseCB.query().setPaymentCompleteFlg_Equal_True();
* }).greaterEqual(123); // *Don't forget the parameter
*
* @param derivedCBLambda The callback for sub-query of referrer. (NotNull)
* @return The parameter for comparing with scalar. (NotNull)
*/
public HpQDRParameter avg(SubQuery derivedCBLambda) {
return doAvg(derivedCBLambda, null);
}
/**
* An overload method for avg(). So refer to the method's java-doc about basic info.
*
* cb.query().derivedPurchaseList().avg(purchaseCB -> {
* purchaseCB.specify().columnPurchasePrice(); // *Point
* purchaseCB.query().setPaymentCompleteFlg_Equal_True();
* }).greaterEqual(123, op -> op.coalesce(0));
*
* @param derivedCBLambda The callback for sub-query of referrer. (NotNull)
* @param opLambda The callback for option of DerivedReferrer. For example, you can use a coalesce function. (NotNull)
* @return The parameter for comparing with scalar. (NotNull)
*/
public HpQDRParameter avg(SubQuery derivedCBLambda, FunctionFilterOptionCall opLambda) {
assertDerivedReferrerOption(opLambda);
final DerivedReferrerOption option = createDerivedReferrerOption();
opLambda.callback(option);
return doAvg(derivedCBLambda, option);
}
protected HpQDRParameter doAvg(SubQuery subQuery, DerivedReferrerOption option) {
assertSubQuery(subQuery);
return createQDRParameter("avg", subQuery, option);
}
// ===================================================================================
// Assist Helper
// =============
protected DerivedReferrerOption createDerivedReferrerOption() {
return _derivedReferrerOptionFactory.create();
}
protected HpQDRParameter createQDRParameter(String fuction, SubQuery subQuery,
DerivedReferrerOption option) {
return new HpQDRParameter(fuction, subQuery, option, _setupper);
}
protected void assertSubQuery(SubQuery> subQuery) {
if (subQuery == null) {
String msg = "The argument 'subQuery' for DerivedReferrer should not be null.";
throw new IllegalArgumentException(msg);
}
}
protected void assertDerivedReferrerOption(FunctionFilterOptionCall opLambda) {
if (opLambda == null) {
String msg = "The argument 'opLambda' for DerivedReferrer should not be null.";
throw new IllegalArgumentException(msg);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy