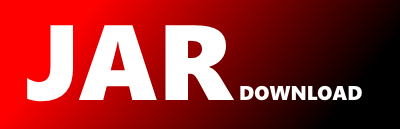
org.dbflute.cbean.paging.numberlink.group.PageGroupBean Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of dbflute-runtime Show documentation
Show all versions of dbflute-runtime Show documentation
The runtime library of DBFlute
/*
* Copyright 2014-2023 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND,
* either express or implied. See the License for the specific language
* governing permissions and limitations under the License.
*/
package org.dbflute.cbean.paging.numberlink.group;
import java.io.Serializable;
import java.util.ArrayList;
import java.util.List;
import org.dbflute.cbean.paging.numberlink.PageNumberLink;
import org.dbflute.cbean.paging.numberlink.PageNumberLinkSetupper;
/**
* The bean of page group.
* @author jflute
*/
public class PageGroupBean implements Serializable {
// ===================================================================================
// Definition
// ==========
/** The serial version UID for object serialization. (Default) */
private static final long serialVersionUID = 1L;
// ===================================================================================
// Attribute
// =========
protected int _currentPageNumber;
protected int _allPageCount;
protected PageGroupOption _pageGroupOption;
protected List _cachedPageNumberList;
// ===================================================================================
// Page Number List
// ================
/**
* Build the list of page number link.
*
* page.setPageGroupSize(10);
* List<PageNumberLink> linkList = page.pageGroup().buildPageNumberLinkList(new PageNumberLinkSetupper<PageNumberLink>() {
* public PageNumberLink setup(int pageNumberElement, boolean current) {
* String href = buildPagingHref(pageNumberElement); // for paging navigation links
* return new PageNumberLink().initialize(pageNumberElement, current, href);
* }
* });
*
* @param The type of link.
* @param manyArgLambda Page number link set-upper. (NotNull and Required LINK)
* @return The list of Page number link. (NotNull)
*/
public List buildPageNumberLinkList(PageNumberLinkSetupper manyArgLambda) {
final List pageNumberList = createPageNumberList();
final List pageNumberLinkList = new ArrayList();
for (Integer pageNumber : pageNumberList) {
pageNumberLinkList.add(manyArgLambda.setup(pageNumber, pageNumber.equals(_currentPageNumber)));
}
return pageNumberLinkList;
}
/**
* Create the list of page number.
*
* e.g. group-size=10, current-page=8
* page.setPageGroupSize(10);
* List<Integer> numberList = page.pageGroup().createPageNumberList();
*
* // 8 / 23 pages (453 records)
* // 1 2 3 4 5 6 7 8 9 10 next
*
* @return The list of page number. (NotNull)
*/
public List createPageNumberList() {
assertPageGroupValid();
if (_cachedPageNumberList != null) {
return _cachedPageNumberList;
}
final int pageGroupSize = _pageGroupOption.getPageGroupSize();
final int allPageCount = _allPageCount;
final int currentPageGroupStartPageNumber = calculateStartPageNumber();
if (!(currentPageGroupStartPageNumber > 0)) {
String msg = "currentPageGroupStartPageNumber should be greater than 0. {> 0} But:";
msg = msg + " currentPageGroupStartPageNumber=" + currentPageGroupStartPageNumber;
throw new IllegalStateException(msg);
}
final int nextPageGroupStartPageNumber = currentPageGroupStartPageNumber + pageGroupSize;
final List resultList = new ArrayList();
for (int i = currentPageGroupStartPageNumber; i < nextPageGroupStartPageNumber && i <= allPageCount; i++) {
resultList.add(Integer.valueOf(i));
}
_cachedPageNumberList = resultList;
return _cachedPageNumberList;
}
/**
* Calculate start page number.
* @return Start page number.
*/
protected int calculateStartPageNumber() {
assertPageGroupValid();
final int pageGroupSize = _pageGroupOption.getPageGroupSize();
final int currentPageNumber = _currentPageNumber;
int currentPageGroupNumber = (currentPageNumber / pageGroupSize);
if ((currentPageNumber % pageGroupSize) == 0) {
currentPageGroupNumber--;
}
final int currentPageGroupStartPageNumber = (pageGroupSize * currentPageGroupNumber) + 1;
if (!(currentPageNumber >= currentPageGroupStartPageNumber)) {
String msg = "currentPageNumber should be greater equal currentPageGroupStartPageNumber. But:";
msg = msg + " currentPageNumber=" + currentPageNumber;
msg = msg + " currentPageGroupStartPageNumber=" + currentPageGroupStartPageNumber;
throw new IllegalStateException(msg);
}
return currentPageGroupStartPageNumber;
}
// ===================================================================================
// Determination
// =============
/**
* Does the previous group exist?
* Using values are currentPageNumber and pageGroupSize.
*
* e.g. group-size=10, current-page=12
* 12 / 23 pages (453 records)
* previous 11 12 13 14 15 16 17 18 19 20 next
*
* // this method returns existence of 10
*
* @return The determination, true or false.
*/
public boolean existsPreviousGroup() {
assertPageGroupValid();
return (_currentPageNumber > _pageGroupOption.getPageGroupSize());
}
/**
* Does the next group exist?
* Using values are currentPageNumber and pageGroupSize and allPageCount.
*
* e.g. group-size=10, current-page=12
* 12 / 23 pages (453 records)
* previous 11 12 13 14 15 16 17 18 19 20 next
*
* // this method returns existence of 21
*
* @return The determination, true or false.
*/
public boolean existsNextGroup() {
assertPageGroupValid();
final int currentStartPageNumber = calculateStartPageNumber();
if (!(currentStartPageNumber > 0)) {
String msg = "currentStartPageNumber should be greater than 0. {> 0} But:";
msg = msg + " currentStartPageNumber=" + currentStartPageNumber;
throw new IllegalStateException(msg);
}
final int nextStartPageNumber = currentStartPageNumber + _pageGroupOption.getPageGroupSize();
return (nextStartPageNumber <= _allPageCount);
}
/**
* Is existing previous page-group?
* Using values are currentPageNumber and pageGroupSize.
*
* e.g. group-size=10, current-page=12
* 12 / 23 pages (453 records)
* previous 11 12 13 14 15 16 17 18 19 20 next
*
* // this method returns existence of 10
*
* @return The determination, true or false.
* @deprecated use existsPreviousGroup()
*/
public boolean isExistPrePageGroup() {
return existsPreviousGroup();
}
/**
* Is existing next page-group?
* Using values are currentPageNumber and pageGroupSize and allPageCount.
*
* e.g. group-size=10, current-page=12
* 12 / 23 pages (453 records)
* previous 11 12 13 14 15 16 17 18 19 20 next
*
* // this method returns existence of 21
*
* @return The determination, true or false.
* @deprecated use existsNextGroup()
*/
public boolean isExistNextPageGroup() {
return existsNextGroup();
}
// ===================================================================================
// Assist Helper
// =============
protected int[] convertListToIntArray(List ls) {
final int[] resultArray = new int[ls.size()];
int arrayIndex = 0;
for (int pageNumber : resultArray) {
resultArray[arrayIndex] = pageNumber;
arrayIndex++;
}
return resultArray;
}
protected void assertPageGroupValid() {
if (_pageGroupOption == null) {
String msg = "The pageGroupOption should not be null. Please call setPageGroupOption().";
throw new IllegalStateException(msg);
}
if (_pageGroupOption.getPageGroupSize() == 0) {
String msg = "The pageGroupSize should be greater than 1. But the value is zero.";
msg = msg + " pageGroupSize=" + _pageGroupOption.getPageGroupSize();
throw new IllegalStateException(msg);
}
if (_pageGroupOption.getPageGroupSize() == 1) {
String msg = "The pageGroupSize should be greater than 1. But the value is one.";
msg = msg + " pageGroupSize=" + _pageGroupOption.getPageGroupSize();
throw new IllegalStateException(msg);
}
}
// ===================================================================================
// Basic Override
// ==============
/**
* @return The view string of all attribute values. (NotNull)
*/
@Override
public String toString() {
final StringBuilder sb = new StringBuilder();
sb.append("{");
sb.append("currentPageNumber=").append(_currentPageNumber);
sb.append(", allPageCount=").append(_allPageCount);
sb.append(", pageGroupOption=").append(_pageGroupOption);
sb.append("}");
return sb.toString();
}
// ===================================================================================
// Accessor
// ========
public void setCurrentPageNumber(int currentPageNumber) {
_currentPageNumber = currentPageNumber;
}
public void setAllPageCount(int allPageCount) {
_allPageCount = allPageCount;
}
public PageGroupOption getPageGroupOption() {
return _pageGroupOption;
}
public void setPageGroupOption(PageGroupOption pageGroupOption) {
_pageGroupOption = pageGroupOption;
}
// -----------------------------------------------------
// Calculated Property
// -------------------
/**
* Get the value of previousGroupNearestPageNumber that is calculated.
* You should use this.existsPreviousGroup() before calling this. (call only when true)
*
* e.g. group-size=10, current-page=12
* 12 / 23 pages (453 records)
* previous 11 12 13 14 15 16 17 18 19 20 next
*
* // this method returns 10
*
* @return The number of previous group-nearest page.
*/
public int getPreviousGroupNearestPageNumber() {
if (!existsPreviousGroup()) {
String msg = "The previous page range should exist when you use previousGroupNearestPageNumber:";
msg = msg + " currentPageNumber=" + _currentPageNumber + " allPageCount=" + _allPageCount;
msg = msg + " pageGroupOption=" + _pageGroupOption;
throw new IllegalStateException(msg);
}
return createPageNumberList().get(0) - 1;
}
/**
* Get the value of nextGroupNearestPageNumber that is calculated.
* You should use this.existsNextGroup() before calling this. (call only when true)
*
* e.g. group-size=10, current-page=12
* 12 / 23 pages (453 records)
* previous 11 12 13 14 15 16 17 18 19 20 next
*
* // this method returns 21
*
* @return The number of next group-nearest page.
*/
public int getNextGroupNearestPageNumber() {
if (!existsNextGroup()) {
String msg = "The next page range should exist when you use nextGroupNearestPageNumber:";
msg = msg + " currentPageNumber=" + _currentPageNumber + " allPageCount=" + _allPageCount;
msg = msg + " pageGroupOption=" + _pageGroupOption;
throw new IllegalStateException(msg);
}
final List ls = createPageNumberList();
return ls.get(ls.size() - 1) + 1;
}
/**
* Get the value of preGroupNearestPageNumber that is calculated.
* You should use this.existsPreviousGroup() before calling this. (call only when true)
*
* e.g. group-size=10, current-page=12
* 12 / 23 pages (453 records)
* previous 11 12 13 14 15 16 17 18 19 20 next
*
* // this method returns 10
*
* @return The value of preGroupNearestPageNumber.
* @deprecated use getPreviousGroupNearestPageNumber()
*/
public int getPreGroupNearestPageNumber() {
return getPreviousGroupNearestPageNumber();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy