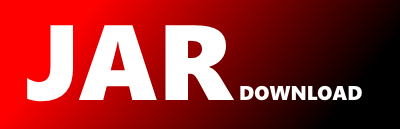
org.dbflute.infra.doc.hacomment.DfHacoMapPiece Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of dbflute-runtime Show documentation
Show all versions of dbflute-runtime Show documentation
The runtime library of DBFlute
/*
* Copyright 2014-2023 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND,
* either express or implied. See the License for the specific language
* governing permissions and limitations under the License.
*/
package org.dbflute.infra.doc.hacomment;
import java.time.LocalDateTime;
import java.util.ArrayList;
import java.util.LinkedHashMap;
import java.util.List;
import java.util.Map;
/**
* @author hakiba
*/
public class DfHacoMapPiece {
// ===================================================================================
// Attribute
// =========
protected final String diffCode;
protected final String diffDate;
protected final String hacomment;
protected final String diffComment;
protected final List authorList;
protected final String pieceCode;
protected final String pieceOwner;
protected final LocalDateTime pieceDatetime;
protected final List previousPieceList;
// ===================================================================================
// Constructor
// ===========
public DfHacoMapPiece(String diffCode, String diffDate, String hacomment, String diffComment, List authorList, String pieceCode,
String pieceOwner, LocalDateTime pieceDatetime, List previousPieceList) {
this.diffCode = diffCode;
this.diffDate = diffDate;
this.hacomment = hacomment;
this.diffComment = diffComment;
this.authorList = new ArrayList<>(authorList);
if (!authorList.contains(pieceOwner)) {
this.authorList.add(pieceOwner);
}
this.pieceCode = pieceCode;
this.pieceOwner = pieceOwner;
this.pieceDatetime = pieceDatetime;
this.previousPieceList = previousPieceList;
}
@SuppressWarnings("unchecked")
public DfHacoMapPiece(Map map) {
this.diffCode = (String) map.get("diffCode");
this.diffDate = (String) map.get("diffDate");
this.hacomment = (String) map.get("hacomment");
this.diffComment = (String) map.get("diffComment");
this.authorList = (List) map.get("authorList");
this.pieceCode = (String) map.get("pieceCode");
this.pieceOwner = (String) map.get("pieceOwner");
this.pieceDatetime = (LocalDateTime) map.get("pieceDatetime");
this.previousPieceList = (List) map.get("previousPieceList");
}
// ===================================================================================
// Converter
// =========
public Map convertToMap() {
Map map = new LinkedHashMap<>();
map.put("diffCode", this.diffCode);
map.put("diffDate", this.diffDate);
map.put("hacomment", this.hacomment);
map.put("diffComment", this.diffComment);
map.put("authorList", this.authorList);
map.put("pieceCode", this.pieceCode);
map.put("pieceOwner", this.pieceOwner);
map.put("pieceDatetime", this.pieceDatetime);
map.put("previousPieceList", this.previousPieceList);
return map;
}
// ===================================================================================
// Accessor
// ========
public String getDiffCode() {
return diffCode;
}
public String getDiffDate() {
return diffDate;
}
public String getHacomment() {
return hacomment;
}
public String getDiffComment() {
return diffComment;
}
public List getAuthorList() {
return authorList;
}
public String getPieceCode() {
return pieceCode;
}
public String getPieceOwner() {
return pieceOwner;
}
public LocalDateTime getPieceDatetime() {
return pieceDatetime;
}
public List getPreviousPieceList() {
return previousPieceList;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy