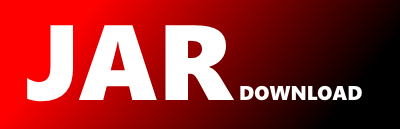
org.dbflute.optional.OptionalObject Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of dbflute-runtime Show documentation
Show all versions of dbflute-runtime Show documentation
The runtime library of DBFlute
/*
* Copyright 2014-2023 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND,
* either express or implied. See the License for the specific language
* governing permissions and limitations under the License.
*/
package org.dbflute.optional;
import java.util.stream.Stream;
import org.dbflute.helper.function.IndependentProcessor;
/**
* @param The type of object.
* @author jflute
* @since 1.1.0-sp1 (2015/01/19 Monday)
*/
public class OptionalObject extends BaseOptional {
// ===================================================================================
// Definition
// ==========
private static final long serialVersionUID = 1L; // basically cannot use (for optional entity)
protected static final OptionalObject
© 2015 - 2025 Weber Informatics LLC | Privacy Policy