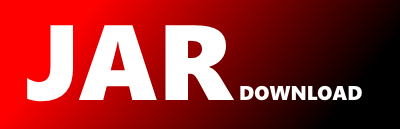
org.dbflute.outsidesql.executor.OutsideSqlAllFacadeExecutor Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of dbflute-runtime Show documentation
Show all versions of dbflute-runtime Show documentation
The runtime library of DBFlute
/*
* Copyright 2014-2023 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND,
* either express or implied. See the License for the specific language
* governing permissions and limitations under the License.
*/
package org.dbflute.outsidesql.executor;
import org.dbflute.bhv.exception.BehaviorExceptionThrower;
import org.dbflute.cbean.coption.StatementConfigCall;
import org.dbflute.cbean.result.ListResultBean;
import org.dbflute.cbean.result.PagingResultBean;
import org.dbflute.exception.IllegalConditionBeanOperationException;
import org.dbflute.jdbc.CursorHandler;
import org.dbflute.jdbc.StatementConfig;
import org.dbflute.optional.OptionalEntity;
import org.dbflute.outsidesql.OutsideSqlOption;
import org.dbflute.outsidesql.ProcedurePmb;
import org.dbflute.outsidesql.typed.AutoPagingHandlingPmb;
import org.dbflute.outsidesql.typed.CursorHandlingPmb;
import org.dbflute.outsidesql.typed.EntityHandlingPmb;
import org.dbflute.outsidesql.typed.ExecuteHandlingPmb;
import org.dbflute.outsidesql.typed.ListHandlingPmb;
import org.dbflute.outsidesql.typed.ManualPagingHandlingPmb;
import org.dbflute.outsidesql.typed.PagingHandlingPmb;
/**
* The all facade executor of outside-SQL.
*
* // main style
* memberBhv.outideSql().selectEntity(pmb); // OptionalEntity
* memberBhv.outideSql().selectList(pmb); // ListResultBean
* memberBhv.outideSql().selectPage(pmb); // PagingResultBean
* memberBhv.outideSql().selectPagedListOnly(pmb); // ListResultBean
* memberBhv.outideSql().selectCursor(pmb, handler); // (by handler)
* memberBhv.outideSql().execute(pmb); // int (updated count)
* memberBhv.outideSql().call(pmb); // void (pmb has OUT parameters)
*
* // traditional style
* memberBhv.outideSql().traditionalStyle().selectEntity(path, pmb, entityType);
* memberBhv.outideSql().traditionalStyle().selectList(path, pmb, entityType);
* memberBhv.outideSql().traditionalStyle().selectPage(path, pmb, entityType);
* memberBhv.outideSql().traditionalStyle().selectPagedListOnly(path, pmb, entityType);
* memberBhv.outideSql().traditionalStyle().selectCursor(path, pmb, handler);
* memberBhv.outideSql().traditionalStyle().execute(path, pmb);
*
* // options
* memberBhv.outideSql().removeBlockComment().selectList()
* memberBhv.outideSql().removeLineComment().selectList()
* memberBhv.outideSql().formatSql().selectList()
*
* @param The type of behavior.
* @author jflute
* @since 1.1.0 (2014/10/13)
*/
public class OutsideSqlAllFacadeExecutor {
// ===================================================================================
// Attribute
// =========
/** The basic executor of outside-SQL. (NotNull) */
protected final OutsideSqlBasicExecutor _basicExecutor;
// ===================================================================================
// Constructor
// ===========
public OutsideSqlAllFacadeExecutor(OutsideSqlBasicExecutor basicExecutor) {
_basicExecutor = basicExecutor;
}
// ===================================================================================
// Entity Select
// =============
/**
* Select entity by the outside-SQL. {Typed Interface}
* You can call this method by only a typed parameter-bean
* which is related to its own (outside-SQL) path and entity-type.
*
* SimpleMemberPmb pmb = new SimpleMemberPmb();
* pmb.setMemberId(3);
* SimpleMember member
* = memberBhv.outsideSql().selectEntity(pmb);
* if (member != null) {
* ... = member.get...();
* } else {
* ...
* }
*
* @param The type of entity.
* @param pmb The typed parameter-bean for entity handling. (NotNull)
* @return The optional entity selected by the outside-SQL. (NotNull: if no data, returns empty entity)
* @throws org.dbflute.exception.OutsideSqlNotFoundException When the outside-SQL is not found.
* @throws org.dbflute.exception.EntityDuplicatedException When the entity is duplicated.
*/
public OptionalEntity selectEntity(EntityHandlingPmb pmb) {
return OptionalEntity.ofNullable(_basicExecutor.entityHandling().selectEntity(pmb), () -> {
createBhvExThrower().throwSelectEntityAlreadyDeletedException(pmb);
});
}
// ===================================================================================
// List Select
// ===========
/**
* Select the list of the entity by the outsideSql. {Typed Interface}
* You can call this method by only a typed parameter-bean
* which is related to its own (outside-SQL) path and entity-type.
*
* SimpleMemberPmb pmb = new SimpleMemberPmb();
* pmb.setMemberName_PrefixSearch("S");
* ListResultBean<SimpleMember> memberList
* = memberBhv.outsideSql().selectList(pmb);
* for (SimpleMember member : memberList) {
* ... = member.get...();
* }
*
* It needs to use customize-entity and parameter-bean.
* The way to generate them is following:
*
* -- #df:entity#
* -- !df:pmb!
* -- !!Integer memberId!!
* -- !!String memberName!!
* -- !!...!!
*
* @param The type of entity for element.
* @param pmb The typed parameter-bean for list handling. (NotNull)
* @return The result bean of selected list. (NotNull)
* @throws org.dbflute.exception.OutsideSqlNotFoundException When the outsideSql is not found.
* @throws org.dbflute.exception.DangerousResultSizeException When the result size is over the specified safety size.
*/
public ListResultBean selectList(ListHandlingPmb pmb) {
return _basicExecutor.selectList(pmb);
}
// ===================================================================================
// Paging Select
// =============
/**
* Select page by the outside-SQL. {Typed Interface}
* (both count-select and paging-select are executed)
* You can call this method by only a typed parameter-bean
* which is related to its own (outside-SQL) path and entity-type.
*
* SimpleMemberPmb pmb = new SimpleMemberPmb();
* pmb.setMemberName_PrefixSearch("S");
* pmb.paging(20, 3); // 20 records per a page and current page number is 3
* PagingResultBean<SimpleMember> page
* = memberBhv.outsideSql().selectPage(pmb);
* int allRecordCount = page.getAllRecordCount();
* int allPageCount = page.getAllPageCount();
* boolean isExistPrePage = page.isExistPrePage();
* boolean isExistNextPage = page.isExistNextPage();
* ...
* for (SimpleMember member : page) {
* ... = member.get...();
* }
*
* The parameter-bean needs to extend SimplePagingBean.
* The way to generate it is following:
*
* -- !df:pmb extends Paging!
* -- !!Integer memberId!!
* -- !!...!!
*
* You can realize by pagingBean's isPaging() method on your 'Parameter Comment'.
* It returns false when it executes Count. And it returns true when it executes Paging.
*
* e.g. ManualPaging and MySQL
* /*IF pmb.isPaging()*/
* select member.MEMBER_ID
* , member.MEMBER_NAME
* , memberStatus.MEMBER_STATUS_NAME
* -- ELSE select count(*)
* /*END*/
* from MEMBER member
* /*IF pmb.isPaging()*/
* left outer join MEMBER_STATUS memberStatus
* on member.MEMBER_STATUS_CODE = memberStatus.MEMBER_STATUS_CODE
* /*END*/
* /*BEGIN*/
* where
* /*IF pmb.memberId != null*/
* member.MEMBER_ID = /*pmb.memberId*/'123'
* /*END*/
* /*IF pmb.memberName != null*/
* and member.MEMBER_NAME like /*pmb.memberName*/'Billy%'
* /*END*/
* /*END*/
* /*IF pmb.isPaging()*/
* order by member.UPDATE_DATETIME desc
* /*END*/
* /*IF pmb.isPaging()*/
* limit /*pmb.pageStartIndex*/80, /*pmb.fetchSize*/20
* /*END*/
*
* @param The type of entity.
* @param pmb The typed parameter-bean for paging handling. (NotNull)
* @return The result bean of paging. (NotNull)
* @throws org.dbflute.exception.OutsideSqlNotFoundException When the outside-SQL is not found.
* @throws org.dbflute.exception.DangerousResultSizeException When the result size is over the specified safety size.
*/
public PagingResultBean selectPage(PagingHandlingPmb pmb) {
if (pmb instanceof ManualPagingHandlingPmb) {
return _basicExecutor.manualPaging().selectPage((ManualPagingHandlingPmb) pmb);
} else if (pmb instanceof AutoPagingHandlingPmb) {
return _basicExecutor.autoPaging().selectPage((AutoPagingHandlingPmb) pmb);
} else {
String msg = "Unknown paging handling parameter-bean: " + pmb;
throw new IllegalStateException(msg);
}
}
/**
* Select list with paging by the outside-SQL. {Typed Interface}
* (count-select is not executed, only paging-select)
* You can call this method by only a typed parameter-bean
* which is related to its own (outside-SQL) path and entity-type.
*
* SimpleMemberPmb pmb = new SimpleMemberPmb();
* pmb.setMemberName_PrefixSearch("S");
* pmb.paging(20, 3); // 20 records per a page and current page number is 3
* ListResultBean<SimpleMember> memberList
* = memberBhv.outsideSql().selectPagedListOnly(pmb);
* for (SimpleMember member : memberList) {
* ... = member.get...();
* }
*
* The parameter-bean needs to extend SimplePagingBean.
* The way to generate it is following:
*
* -- !df:pmb extends Paging!
* -- !!Integer memberId!!
* -- !!...!!
*
* You don't need to use pagingBean's isPaging() method on your 'Parameter Comment'.
*
* e.g. ManualPaging and MySQL
* select member.MEMBER_ID
* , member.MEMBER_NAME
* , memberStatus.MEMBER_STATUS_NAME
* from MEMBER member
* left outer join MEMBER_STATUS memberStatus
* on member.MEMBER_STATUS_CODE = memberStatus.MEMBER_STATUS_CODE
* /*BEGIN*/
* where
* /*IF pmb.memberId != null*/
* member.MEMBER_ID = /*pmb.memberId*/'123'
* /*END*/
* /*IF pmb.memberName != null*/
* and member.MEMBER_NAME like /*pmb.memberName*/'Billy%'
* /*END*/
* /*END*/
* order by member.UPDATE_DATETIME desc
* limit /*pmb.pageStartIndex*/80, /*pmb.fetchSize*/20
*
* @param The type of entity.
* @param pmb The typed parameter-bean for paging handling. (NotNull)
* @return The result bean of paged list. (NotNull)
* @throws org.dbflute.exception.OutsideSqlNotFoundException When the outside-SQL is not found.
* @throws org.dbflute.exception.DangerousResultSizeException When the result size is over the specified safety size.
*/
public ListResultBean selectPagedListOnly(PagingHandlingPmb pmb) {
if (pmb instanceof ManualPagingHandlingPmb) {
return _basicExecutor.manualPaging().selectList((ManualPagingHandlingPmb) pmb);
} else if (pmb instanceof AutoPagingHandlingPmb) {
return _basicExecutor.autoPaging().selectList((AutoPagingHandlingPmb) pmb);
} else {
String msg = "Unknown paging handling parameter-bean: " + pmb;
throw new IllegalStateException(msg);
}
}
// ===================================================================================
// Cursor Select
// =============
/**
* Select the cursor of the entity by outside-SQL. {Typed Interface}
* You can call this method by only a typed parameter-bean
* which is related to its own (outside-SQL) path.
*
* SimpleMemberPmb pmb = new SimpleMemberPmb();
* pmb.setMemberName_PrefixSearch("S");
* memberBhv.outsideSql().selectCursor(pmb, new PurchaseSummaryMemberCursorHandler() {
* protected Object fetchCursor(PurchaseSummaryMemberCursor cursor) throws SQLException {
* while (cursor.next()) {
* Integer memberId = cursor.getMemberId();
* String memberName = cursor.getMemberName();
* ...
* }
* return null;
* }
* });
*
* It needs to use type-safe-cursor instead of customize-entity.
* The way to generate it is following:
*
* -- #df:entity#
* -- +cursor+
*
* @param The type of entity, might be void.
* @param pmb The typed parameter-bean for cursor handling. (NotNull)
* @param handler The handler of cursor called back with result set. (NotNull)
* @return The result object that the cursor handler returns. (NullAllowed)
* @throws org.dbflute.exception.OutsideSqlNotFoundException When the outside-SQL is not found.
*/
public Object selectCursor(CursorHandlingPmb pmb, CursorHandler handler) {
return _basicExecutor.cursorHandling().selectCursor(pmb, handler);
}
// ===================================================================================
// Execute
// =======
/**
* Execute the outsideSql. (insert, update, delete, etc...) {Typed Interface}
* You can call this method by only a typed parameter-bean
* which is related to its own (outside-SQL) path.
*
* SimpleMemberPmb pmb = new SimpleMemberPmb();
* pmb.setMemberId(3);
* int count = memberBhv.outsideSql().execute(pmb);
*
* @param pmb The parameter-bean. Allowed types are Bean object and Map object. (NullAllowed)
* @return The count of execution.
* @throws org.dbflute.exception.OutsideSqlNotFoundException When the outsideSql is not found.
*/
public int execute(ExecuteHandlingPmb pmb) {
return _basicExecutor.execute(pmb);
}
// [DBFlute-0.7.5]
// ===================================================================================
// Procedure Call
// ==============
/**
* Call the procedure.
*
* SpInOutParameterPmb pmb = new SpInOutParameterPmb();
* pmb.setVInVarchar("foo");
* pmb.setVInOutVarchar("bar");
* memberBhv.outsideSql().call(pmb);
* String outVar = pmb.getVOutVarchar();
*
* It needs to use parameter-bean for procedure (ProcedurePmb).
* The way to generate is to set the option of DBFlute property and execute Sql2Entity.
* @param pmb The parameter-bean for procedure. (NotNull)
*/
public void call(ProcedurePmb pmb) {
_basicExecutor.call(pmb);
}
// [DBFlute-1.1.0]
// ===================================================================================
// Traditional Style
// =================
public OutsideSqlTraditionalExecutor traditionalStyle() {
return new OutsideSqlTraditionalExecutor(_basicExecutor);
}
// ===================================================================================
// Option
// ======
// -----------------------------------------------------
// Remove from SQL
// ---------------
/**
* Set up remove-block-comment for this outsideSql.
* @return this. (NotNull)
*/
public OutsideSqlAllFacadeExecutor removeBlockComment() {
_basicExecutor.removeBlockComment();
return this;
}
/**
* Set up remove-line-comment for this outsideSql.
* @return this. (NotNull)
*/
public OutsideSqlAllFacadeExecutor removeLineComment() {
_basicExecutor.removeLineComment();
return this;
}
// -----------------------------------------------------
// Format SQL
// ----------
/**
* Set up format-SQL for this outsideSql.
* (For example, empty lines removed)
* @return this. (NotNull)
*/
public OutsideSqlAllFacadeExecutor formatSql() {
_basicExecutor.formatSql();
return this;
}
// -----------------------------------------------------
// StatementConfig
// ---------------
/**
* Configure statement JDBC options. e.g. queryTimeout, fetchSize, ... (only one-time call)
*
* memberBhv.outsideSql().configure(conf -> conf.queryTimeout(3)).selectList(...);
*
* @param confLambda The callback for configuration of statement. (NotNull)
* @return this. (NotNull)
*/
public OutsideSqlAllFacadeExecutor configure(StatementConfigCall confLambda) {
if (confLambda == null) {
throw new IllegalArgumentException("The argument 'confLambda' should not be null.");
}
assertStatementConfigNotDuplicated(confLambda);
_basicExecutor.configure(createStatementConfig(confLambda));
return this;
}
protected void assertStatementConfigNotDuplicated(StatementConfigCall configCall) {
final OutsideSqlOption option = _basicExecutor.getOutsideSqlOption();
if (option != null) {
final StatementConfig existingConfig = option.getStatementConfig();
if (existingConfig != null) {
String msg = "Already registered the configuration: existing=" + existingConfig + ", new=" + configCall;
throw new IllegalConditionBeanOperationException(msg);
}
}
}
protected StatementConfig createStatementConfig(StatementConfigCall configCall) {
if (configCall == null) {
throw new IllegalArgumentException("The argument 'confLambda' should not be null.");
}
final StatementConfig config = newStatementConfig();
configCall.callback(config);
return config;
}
protected StatementConfig newStatementConfig() {
return new StatementConfig();
}
// ===================================================================================
// Exception Helper
// ================
protected BehaviorExceptionThrower createBhvExThrower() {
return _basicExecutor.createBhvExThrower();
}
// ===================================================================================
// Accessor
// ========
public OutsideSqlBasicExecutor xbasicExecutor() { // for compatible use
return _basicExecutor;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy