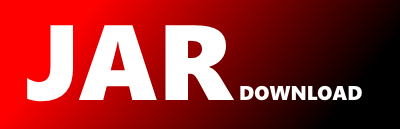
org.dbunit.dataset.xml.FlatXmlDataSet Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of dbunit Show documentation
Show all versions of dbunit Show documentation
dbUnit is a JUnit extension (also usable from Ant and Maven) targeted for database-driven projects that, among other things, puts your database into a known state between test runs. This is an excellent way to avoid the myriad of problems that can occur when one test case corrupts the database and causes subsequent tests to fail or exacerbate the damage.
/*
*
* The DbUnit Database Testing Framework
* Copyright (C)2002-2004, DbUnit.org
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
*
*/
package org.dbunit.dataset.xml;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.dbunit.dataset.CachedDataSet;
import org.dbunit.dataset.DataSetException;
import org.dbunit.dataset.IDataSet;
import org.xml.sax.InputSource;
import java.io.File;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import java.io.Reader;
import java.io.Writer;
import java.net.URL;
/**
* Reads and writes flat XML dataset document. Each XML element corresponds to a table row.
* Each XML element name corresponds to a table name. The XML attributes
* correspond to table columns.
*
* Flat XML dataset document sample:
*
*
* <!DOCTYPE dataset SYSTEM "my-dataset.dtd">
* <dataset>
* <TEST_TABLE COL0="row 0 col 0"
* COL1="row 0 col 1"
* COL2="row 0 col 2"/>
* <TEST_TABLE COL1="row 1 col 1"/>
* <SECOND_TABLE COL0="row 0 col 0"
* COL1="row 0 col 1" />
* <EMPTY_TABLE/>
* </dataset>
*
* To specify null values, omit corresponding attribute.
* In the above example, missing COL0 and COL2 attributes of TEST_TABLE second row represents null values.
*
* Table metadata is deduced from the first row of each table by default.
* Beware that DbUnit may think a table misses some columns if the first row of that table has one or more null values.
* You can do one of the following things to avoid this:
*
* - Use a DTD. DbUnit will use the columns declared in the DTD as table metadata.
* DbUnit only supports external system URI. The URI can be absolute or relative.
*
* - Since DBUnit 2.3.0 there is a functionality called "column sensing" which basically
* reads in the whole XML into a buffer and dynamically adds new columns as they appear.
* It can be used as demonstrated in the following example:
*
* boolean enableColumnSensing = true;
* IDataSet dataSet = new FlatXmlDataSet(
* new File("src/xml/flatXmlTableTest.xml"), false, enableColumnSensing);
*
*
*
*
*
* @author Manuel Laflamme
* @version $Revision: 917 $
* @since Mar 12, 2002
*/
public class FlatXmlDataSet extends CachedDataSet
{
/**
* Logger for this class
*/
private static final Logger logger = LoggerFactory.getLogger(FlatXmlDataSet.class);
/**
* Creates an FlatXmlDataSet object with the specified InputSource.
*/
public FlatXmlDataSet(InputSource source) throws IOException, DataSetException
{
super(new FlatXmlProducer(source));
}
/**
* Creates an FlatXmlDataSet object with the specified xml file.
* Relative DOCTYPE uri are resolved from the xml file path.
*
* @param xmlFile the xml file
*/
public FlatXmlDataSet(File xmlFile) throws IOException, DataSetException
{
this(xmlFile, true);
}
/**
* Creates an FlatXmlDataSet object with the specified xml file.
* Relative DOCTYPE uri are resolved from the xml file path.
*
* @param xmlFile the xml file
* @param dtdMetadata if false
do not use DTD as metadata
*/
public FlatXmlDataSet(File xmlFile, boolean dtdMetadata)
throws IOException, DataSetException
{
this(xmlFile.toURL(), dtdMetadata);
}
/**
* Creates an FlatXmlDataSet object with the specified xml file.
* Relative DOCTYPE uri are resolved from the xml file path.
*
* @param xmlFile the xml file
* @param dtdMetadata if false
do not use DTD as metadata
* @param columnSensing Whether or not the columns should be sensed automatically. Every XML row
* is scanned for columns that have not been there in a previous column.
*/
public FlatXmlDataSet(File xmlFile, boolean dtdMetadata, boolean columnSensing)
throws IOException, DataSetException
{
this(xmlFile.toURL(), dtdMetadata, columnSensing);
}
/**
* Creates an FlatXmlDataSet object with the specified xml file.
* Relative DOCTYPE uri are resolved from the xml file path.
*
* @param xmlFile the xml file
* @param dtdMetadata if false
do not use DTD as metadata
* @param columnSensing Whether or not the columns should be sensed automatically. Every XML row
* is scanned for columns that have not been there in a previous column.
* @param caseSensitiveTableNames Whether or not this dataset should use case sensitive table names
*/
public FlatXmlDataSet(File xmlFile, boolean dtdMetadata, boolean columnSensing, boolean caseSensitiveTableNames)
throws IOException, DataSetException
{
this(xmlFile.toURL(), dtdMetadata, columnSensing, caseSensitiveTableNames);
}
/**
* Creates an FlatXmlDataSet object with the specified xml URL.
* Relative DOCTYPE uri are resolved from the xml file path.
*
* @param xmlUrl the xml URL
*/
public FlatXmlDataSet(URL xmlUrl) throws IOException, DataSetException
{
this(xmlUrl, true);
}
/**
* Creates an FlatXmlDataSet object with the specified xml URL.
* Relative DOCTYPE uri are resolved from the xml file path.
*
* @param xmlUrl the xml URL
* @param dtdMetadata if false
do not use DTD as metadata
*/
public FlatXmlDataSet(URL xmlUrl, boolean dtdMetadata)
throws IOException, DataSetException
{
this(xmlUrl, dtdMetadata, false);
}
/**
* Creates an FlatXmlDataSet object with the specified xml URL.
* Relative DOCTYPE uri are resolved from the xml file path.
*
* @param xmlUrl the xml URL
* @param dtdMetadata if false
do not use DTD as metadata
* @param columnSensing Whether or not the columns should be sensed automatically. Every XML row
* is scanned for columns that have not been there in a previous column.
*/
public FlatXmlDataSet(URL xmlUrl, boolean dtdMetadata, boolean columnSensing)
throws IOException, DataSetException
{
super(new FlatXmlProducer(
new InputSource(xmlUrl.toString()), dtdMetadata, columnSensing));
}
/**
* Creates an FlatXmlDataSet object with the specified xml file.
* Relative DOCTYPE uri are resolved from the xml file path.
*
* @param xmlUrl the xml file
* @param dtdMetadata if false
do not use DTD as metadata
* @param columnSensing Whether or not the columns should be sensed automatically. Every XML row
* is scanned for columns that have not been there in a previous column.
* @param caseSensitiveTableNames Whether or not this dataset should use case sensitive table names
*/
public FlatXmlDataSet(URL xmlUrl, boolean dtdMetadata, boolean columnSensing, boolean caseSensitiveTableNames)
throws IOException, DataSetException
{
super(new FlatXmlProducer(
new InputSource(xmlUrl.toString()), dtdMetadata, columnSensing, caseSensitiveTableNames),
caseSensitiveTableNames);
}
/**
* Creates an FlatXmlDataSet object with the specified xml reader.
* Relative DOCTYPE uri are resolved from the current working directory.
*
* @param xmlReader the xml reader
*/
public FlatXmlDataSet(Reader xmlReader) throws IOException, DataSetException
{
this(xmlReader, true);
}
/**
* Creates an FlatXmlDataSet object with the specified xml reader.
* Relative DOCTYPE uri are resolved from the current working directory.
*
* @param xmlReader the xml reader
* @param dtdMetadata if false
do not use DTD as metadata
*/
public FlatXmlDataSet(Reader xmlReader, boolean dtdMetadata)
throws IOException, DataSetException
{
super(new FlatXmlProducer(new InputSource(xmlReader), dtdMetadata));
}
/**
* Creates an FlatXmlDataSet object with the specified xml and dtd readers.
*
* @param xmlReader the xml reader
* @param dtdReader the dtd reader
*/
public FlatXmlDataSet(Reader xmlReader, Reader dtdReader)
throws IOException, DataSetException
{
this(xmlReader, new FlatDtdDataSet(dtdReader));
}
/**
* Creates an FlatXmlDataSet object with the specified xml reader.
*
* @param xmlReader the xml reader
* @param metaDataSet the dataset used as metadata source.
*/
public FlatXmlDataSet(Reader xmlReader, IDataSet metaDataSet)
throws IOException, DataSetException
{
super(new FlatXmlProducer(new InputSource(xmlReader), metaDataSet));
}
/**
* Creates an FlatXmlDataSet object with the specified xml input stream.
* Relative DOCTYPE uri are resolved from the current working directory.
*
* @param xmlStream the xml input stream
*/
public FlatXmlDataSet(InputStream xmlStream) throws IOException, DataSetException
{
this(xmlStream, true);
}
/**
* Creates an FlatXmlDataSet object with the specified xml input stream.
* Relative DOCTYPE uri are resolved from the current working directory.
*
* @param xmlStream the xml input stream
* @param dtdMetadata if false
do not use DTD as metadata
*/
public FlatXmlDataSet(InputStream xmlStream, boolean dtdMetadata)
throws IOException, DataSetException
{
super(new FlatXmlProducer(new InputSource(xmlStream), dtdMetadata));
}
/**
* Creates an FlatXmlDataSet object with the specified xml and dtd input
* stream.
*
* @param xmlStream the xml input stream
* @param dtdStream the dtd input stream
*/
public FlatXmlDataSet(InputStream xmlStream, InputStream dtdStream)
throws IOException, DataSetException
{
this(xmlStream, new FlatDtdDataSet(dtdStream));
}
/**
* Creates an FlatXmlDataSet object with the specified xml input stream.
*
* @param xmlStream the xml input stream
* @param metaDataSet the dataset used as metadata source.
*/
public FlatXmlDataSet(InputStream xmlStream, IDataSet metaDataSet)
throws IOException, DataSetException
{
super(new FlatXmlProducer(new InputSource(xmlStream), metaDataSet));
}
/**
* Write the specified dataset to the specified output stream as xml.
*/
public static void write(IDataSet dataSet, OutputStream out)
throws IOException, DataSetException
{
logger.debug("write(dataSet={}, out={}) - start", dataSet, out);
FlatXmlWriter datasetWriter = new FlatXmlWriter(out);
datasetWriter.setIncludeEmptyTable(true);
datasetWriter.write(dataSet);
}
/**
* Write the specified dataset to the specified writer as xml.
*/
public static void write(IDataSet dataSet, Writer writer)
throws IOException, DataSetException
{
logger.debug("write(dataSet={}, writer={}) - start", dataSet, writer);
write(dataSet, writer, null);
}
/**
* Write the specified dataset to the specified writer as xml.
*/
public static void write(IDataSet dataSet, Writer writer, String encoding)
throws IOException, DataSetException
{
if (logger.isDebugEnabled())
{
logger.debug("write(dataSet={}, writer={}, encoding={}) - start",
new Object[]{ dataSet, writer, encoding });
}
FlatXmlWriter datasetWriter = new FlatXmlWriter(writer, encoding);
datasetWriter.setIncludeEmptyTable(true);
datasetWriter.write(dataSet);
}
/**
* Write a DTD for the specified dataset to the specified output.
* @deprecated use {@link FlatDtdDataSet#write}
*/
public static void writeDtd(IDataSet dataSet, OutputStream out)
throws IOException, DataSetException
{
logger.debug("writeDtd(dataSet={}, out={}) - start", dataSet, out);
FlatDtdDataSet.write(dataSet, out);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy