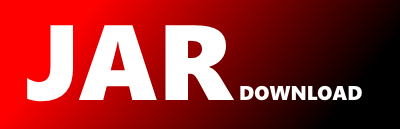
org.deephacks.westty.tests.ClusterMessages Maven / Gradle / Ivy
The newest version!
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: src/main/resources/META-INF/cluster.proto
package org.deephacks.westty.tests;
public final class ClusterMessages {
private ClusterMessages() {}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistry registry) {
}
public interface AsyncSendRequestOrBuilder
extends com.google.protobuf.MessageOrBuilder {
// optional uint32 protoType = 200;
boolean hasProtoType();
int getProtoType();
// required string msg = 1;
boolean hasMsg();
String getMsg();
}
public static final class AsyncSendRequest extends
com.google.protobuf.GeneratedMessage
implements AsyncSendRequestOrBuilder {
// Use AsyncSendRequest.newBuilder() to construct.
private AsyncSendRequest(Builder builder) {
super(builder);
}
private AsyncSendRequest(boolean noInit) {}
private static final AsyncSendRequest defaultInstance;
public static AsyncSendRequest getDefaultInstance() {
return defaultInstance;
}
public AsyncSendRequest getDefaultInstanceForType() {
return defaultInstance;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.deephacks.westty.tests.ClusterMessages.internal_static_westty_tests_AsyncSendRequest_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.deephacks.westty.tests.ClusterMessages.internal_static_westty_tests_AsyncSendRequest_fieldAccessorTable;
}
private int bitField0_;
// optional uint32 protoType = 200;
public static final int PROTOTYPE_FIELD_NUMBER = 200;
private int protoType_;
public boolean hasProtoType() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
public int getProtoType() {
return protoType_;
}
// required string msg = 1;
public static final int MSG_FIELD_NUMBER = 1;
private java.lang.Object msg_;
public boolean hasMsg() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
public String getMsg() {
java.lang.Object ref = msg_;
if (ref instanceof String) {
return (String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
String s = bs.toStringUtf8();
if (com.google.protobuf.Internal.isValidUtf8(bs)) {
msg_ = s;
}
return s;
}
}
private com.google.protobuf.ByteString getMsgBytes() {
java.lang.Object ref = msg_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((String) ref);
msg_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private void initFields() {
protoType_ = 0;
msg_ = "";
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
if (!hasMsg()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeBytes(1, getMsgBytes());
}
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeUInt32(200, protoType_);
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(1, getMsgBytes());
}
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(200, protoType_);
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static org.deephacks.westty.tests.ClusterMessages.AsyncSendRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data).buildParsed();
}
public static org.deephacks.westty.tests.ClusterMessages.AsyncSendRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data, extensionRegistry)
.buildParsed();
}
public static org.deephacks.westty.tests.ClusterMessages.AsyncSendRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data).buildParsed();
}
public static org.deephacks.westty.tests.ClusterMessages.AsyncSendRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data, extensionRegistry)
.buildParsed();
}
public static org.deephacks.westty.tests.ClusterMessages.AsyncSendRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return newBuilder().mergeFrom(input).buildParsed();
}
public static org.deephacks.westty.tests.ClusterMessages.AsyncSendRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return newBuilder().mergeFrom(input, extensionRegistry)
.buildParsed();
}
public static org.deephacks.westty.tests.ClusterMessages.AsyncSendRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
Builder builder = newBuilder();
if (builder.mergeDelimitedFrom(input)) {
return builder.buildParsed();
} else {
return null;
}
}
public static org.deephacks.westty.tests.ClusterMessages.AsyncSendRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
Builder builder = newBuilder();
if (builder.mergeDelimitedFrom(input, extensionRegistry)) {
return builder.buildParsed();
} else {
return null;
}
}
public static org.deephacks.westty.tests.ClusterMessages.AsyncSendRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return newBuilder().mergeFrom(input).buildParsed();
}
public static org.deephacks.westty.tests.ClusterMessages.AsyncSendRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return newBuilder().mergeFrom(input, extensionRegistry)
.buildParsed();
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(org.deephacks.westty.tests.ClusterMessages.AsyncSendRequest prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder
implements org.deephacks.westty.tests.ClusterMessages.AsyncSendRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.deephacks.westty.tests.ClusterMessages.internal_static_westty_tests_AsyncSendRequest_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.deephacks.westty.tests.ClusterMessages.internal_static_westty_tests_AsyncSendRequest_fieldAccessorTable;
}
// Construct using org.deephacks.westty.tests.ClusterMessages.AsyncSendRequest.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
protoType_ = 0;
bitField0_ = (bitField0_ & ~0x00000001);
msg_ = "";
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.deephacks.westty.tests.ClusterMessages.AsyncSendRequest.getDescriptor();
}
public org.deephacks.westty.tests.ClusterMessages.AsyncSendRequest getDefaultInstanceForType() {
return org.deephacks.westty.tests.ClusterMessages.AsyncSendRequest.getDefaultInstance();
}
public org.deephacks.westty.tests.ClusterMessages.AsyncSendRequest build() {
org.deephacks.westty.tests.ClusterMessages.AsyncSendRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
private org.deephacks.westty.tests.ClusterMessages.AsyncSendRequest buildParsed()
throws com.google.protobuf.InvalidProtocolBufferException {
org.deephacks.westty.tests.ClusterMessages.AsyncSendRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(
result).asInvalidProtocolBufferException();
}
return result;
}
public org.deephacks.westty.tests.ClusterMessages.AsyncSendRequest buildPartial() {
org.deephacks.westty.tests.ClusterMessages.AsyncSendRequest result = new org.deephacks.westty.tests.ClusterMessages.AsyncSendRequest(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.protoType_ = protoType_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.msg_ = msg_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.deephacks.westty.tests.ClusterMessages.AsyncSendRequest) {
return mergeFrom((org.deephacks.westty.tests.ClusterMessages.AsyncSendRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.deephacks.westty.tests.ClusterMessages.AsyncSendRequest other) {
if (other == org.deephacks.westty.tests.ClusterMessages.AsyncSendRequest.getDefaultInstance()) return this;
if (other.hasProtoType()) {
setProtoType(other.getProtoType());
}
if (other.hasMsg()) {
setMsg(other.getMsg());
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
if (!hasMsg()) {
return false;
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder(
this.getUnknownFields());
while (true) {
int tag = input.readTag();
switch (tag) {
case 0:
this.setUnknownFields(unknownFields.build());
onChanged();
return this;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
this.setUnknownFields(unknownFields.build());
onChanged();
return this;
}
break;
}
case 10: {
bitField0_ |= 0x00000002;
msg_ = input.readBytes();
break;
}
case 1600: {
bitField0_ |= 0x00000001;
protoType_ = input.readUInt32();
break;
}
}
}
}
private int bitField0_;
// optional uint32 protoType = 200;
private int protoType_ ;
public boolean hasProtoType() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
public int getProtoType() {
return protoType_;
}
public Builder setProtoType(int value) {
bitField0_ |= 0x00000001;
protoType_ = value;
onChanged();
return this;
}
public Builder clearProtoType() {
bitField0_ = (bitField0_ & ~0x00000001);
protoType_ = 0;
onChanged();
return this;
}
// required string msg = 1;
private java.lang.Object msg_ = "";
public boolean hasMsg() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
public String getMsg() {
java.lang.Object ref = msg_;
if (!(ref instanceof String)) {
String s = ((com.google.protobuf.ByteString) ref).toStringUtf8();
msg_ = s;
return s;
} else {
return (String) ref;
}
}
public Builder setMsg(String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
msg_ = value;
onChanged();
return this;
}
public Builder clearMsg() {
bitField0_ = (bitField0_ & ~0x00000002);
msg_ = getDefaultInstance().getMsg();
onChanged();
return this;
}
void setMsg(com.google.protobuf.ByteString value) {
bitField0_ |= 0x00000002;
msg_ = value;
onChanged();
}
// @@protoc_insertion_point(builder_scope:westty.tests.AsyncSendRequest)
}
static {
defaultInstance = new AsyncSendRequest(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:westty.tests.AsyncSendRequest)
}
public interface GetSendRequestOrBuilder
extends com.google.protobuf.MessageOrBuilder {
// optional uint32 protoType = 201;
boolean hasProtoType();
int getProtoType();
}
public static final class GetSendRequest extends
com.google.protobuf.GeneratedMessage
implements GetSendRequestOrBuilder {
// Use GetSendRequest.newBuilder() to construct.
private GetSendRequest(Builder builder) {
super(builder);
}
private GetSendRequest(boolean noInit) {}
private static final GetSendRequest defaultInstance;
public static GetSendRequest getDefaultInstance() {
return defaultInstance;
}
public GetSendRequest getDefaultInstanceForType() {
return defaultInstance;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.deephacks.westty.tests.ClusterMessages.internal_static_westty_tests_GetSendRequest_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.deephacks.westty.tests.ClusterMessages.internal_static_westty_tests_GetSendRequest_fieldAccessorTable;
}
private int bitField0_;
// optional uint32 protoType = 201;
public static final int PROTOTYPE_FIELD_NUMBER = 201;
private int protoType_;
public boolean hasProtoType() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
public int getProtoType() {
return protoType_;
}
private void initFields() {
protoType_ = 0;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeUInt32(201, protoType_);
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(201, protoType_);
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static org.deephacks.westty.tests.ClusterMessages.GetSendRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data).buildParsed();
}
public static org.deephacks.westty.tests.ClusterMessages.GetSendRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data, extensionRegistry)
.buildParsed();
}
public static org.deephacks.westty.tests.ClusterMessages.GetSendRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data).buildParsed();
}
public static org.deephacks.westty.tests.ClusterMessages.GetSendRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data, extensionRegistry)
.buildParsed();
}
public static org.deephacks.westty.tests.ClusterMessages.GetSendRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return newBuilder().mergeFrom(input).buildParsed();
}
public static org.deephacks.westty.tests.ClusterMessages.GetSendRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return newBuilder().mergeFrom(input, extensionRegistry)
.buildParsed();
}
public static org.deephacks.westty.tests.ClusterMessages.GetSendRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
Builder builder = newBuilder();
if (builder.mergeDelimitedFrom(input)) {
return builder.buildParsed();
} else {
return null;
}
}
public static org.deephacks.westty.tests.ClusterMessages.GetSendRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
Builder builder = newBuilder();
if (builder.mergeDelimitedFrom(input, extensionRegistry)) {
return builder.buildParsed();
} else {
return null;
}
}
public static org.deephacks.westty.tests.ClusterMessages.GetSendRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return newBuilder().mergeFrom(input).buildParsed();
}
public static org.deephacks.westty.tests.ClusterMessages.GetSendRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return newBuilder().mergeFrom(input, extensionRegistry)
.buildParsed();
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(org.deephacks.westty.tests.ClusterMessages.GetSendRequest prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder
implements org.deephacks.westty.tests.ClusterMessages.GetSendRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.deephacks.westty.tests.ClusterMessages.internal_static_westty_tests_GetSendRequest_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.deephacks.westty.tests.ClusterMessages.internal_static_westty_tests_GetSendRequest_fieldAccessorTable;
}
// Construct using org.deephacks.westty.tests.ClusterMessages.GetSendRequest.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
protoType_ = 0;
bitField0_ = (bitField0_ & ~0x00000001);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.deephacks.westty.tests.ClusterMessages.GetSendRequest.getDescriptor();
}
public org.deephacks.westty.tests.ClusterMessages.GetSendRequest getDefaultInstanceForType() {
return org.deephacks.westty.tests.ClusterMessages.GetSendRequest.getDefaultInstance();
}
public org.deephacks.westty.tests.ClusterMessages.GetSendRequest build() {
org.deephacks.westty.tests.ClusterMessages.GetSendRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
private org.deephacks.westty.tests.ClusterMessages.GetSendRequest buildParsed()
throws com.google.protobuf.InvalidProtocolBufferException {
org.deephacks.westty.tests.ClusterMessages.GetSendRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(
result).asInvalidProtocolBufferException();
}
return result;
}
public org.deephacks.westty.tests.ClusterMessages.GetSendRequest buildPartial() {
org.deephacks.westty.tests.ClusterMessages.GetSendRequest result = new org.deephacks.westty.tests.ClusterMessages.GetSendRequest(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.protoType_ = protoType_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.deephacks.westty.tests.ClusterMessages.GetSendRequest) {
return mergeFrom((org.deephacks.westty.tests.ClusterMessages.GetSendRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.deephacks.westty.tests.ClusterMessages.GetSendRequest other) {
if (other == org.deephacks.westty.tests.ClusterMessages.GetSendRequest.getDefaultInstance()) return this;
if (other.hasProtoType()) {
setProtoType(other.getProtoType());
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder(
this.getUnknownFields());
while (true) {
int tag = input.readTag();
switch (tag) {
case 0:
this.setUnknownFields(unknownFields.build());
onChanged();
return this;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
this.setUnknownFields(unknownFields.build());
onChanged();
return this;
}
break;
}
case 1608: {
bitField0_ |= 0x00000001;
protoType_ = input.readUInt32();
break;
}
}
}
}
private int bitField0_;
// optional uint32 protoType = 201;
private int protoType_ ;
public boolean hasProtoType() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
public int getProtoType() {
return protoType_;
}
public Builder setProtoType(int value) {
bitField0_ |= 0x00000001;
protoType_ = value;
onChanged();
return this;
}
public Builder clearProtoType() {
bitField0_ = (bitField0_ & ~0x00000001);
protoType_ = 0;
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:westty.tests.GetSendRequest)
}
static {
defaultInstance = new GetSendRequest(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:westty.tests.GetSendRequest)
}
public interface GetSendResponseOrBuilder
extends com.google.protobuf.MessageOrBuilder {
// optional uint32 protoType = 202;
boolean hasProtoType();
int getProtoType();
// repeated string serverMsg = 1;
java.util.List getServerMsgList();
int getServerMsgCount();
String getServerMsg(int index);
}
public static final class GetSendResponse extends
com.google.protobuf.GeneratedMessage
implements GetSendResponseOrBuilder {
// Use GetSendResponse.newBuilder() to construct.
private GetSendResponse(Builder builder) {
super(builder);
}
private GetSendResponse(boolean noInit) {}
private static final GetSendResponse defaultInstance;
public static GetSendResponse getDefaultInstance() {
return defaultInstance;
}
public GetSendResponse getDefaultInstanceForType() {
return defaultInstance;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.deephacks.westty.tests.ClusterMessages.internal_static_westty_tests_GetSendResponse_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.deephacks.westty.tests.ClusterMessages.internal_static_westty_tests_GetSendResponse_fieldAccessorTable;
}
private int bitField0_;
// optional uint32 protoType = 202;
public static final int PROTOTYPE_FIELD_NUMBER = 202;
private int protoType_;
public boolean hasProtoType() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
public int getProtoType() {
return protoType_;
}
// repeated string serverMsg = 1;
public static final int SERVERMSG_FIELD_NUMBER = 1;
private com.google.protobuf.LazyStringList serverMsg_;
public java.util.List
getServerMsgList() {
return serverMsg_;
}
public int getServerMsgCount() {
return serverMsg_.size();
}
public String getServerMsg(int index) {
return serverMsg_.get(index);
}
private void initFields() {
protoType_ = 0;
serverMsg_ = com.google.protobuf.LazyStringArrayList.EMPTY;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
for (int i = 0; i < serverMsg_.size(); i++) {
output.writeBytes(1, serverMsg_.getByteString(i));
}
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeUInt32(202, protoType_);
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
{
int dataSize = 0;
for (int i = 0; i < serverMsg_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeBytesSizeNoTag(serverMsg_.getByteString(i));
}
size += dataSize;
size += 1 * getServerMsgList().size();
}
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(202, protoType_);
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static org.deephacks.westty.tests.ClusterMessages.GetSendResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data).buildParsed();
}
public static org.deephacks.westty.tests.ClusterMessages.GetSendResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data, extensionRegistry)
.buildParsed();
}
public static org.deephacks.westty.tests.ClusterMessages.GetSendResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data).buildParsed();
}
public static org.deephacks.westty.tests.ClusterMessages.GetSendResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data, extensionRegistry)
.buildParsed();
}
public static org.deephacks.westty.tests.ClusterMessages.GetSendResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return newBuilder().mergeFrom(input).buildParsed();
}
public static org.deephacks.westty.tests.ClusterMessages.GetSendResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return newBuilder().mergeFrom(input, extensionRegistry)
.buildParsed();
}
public static org.deephacks.westty.tests.ClusterMessages.GetSendResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
Builder builder = newBuilder();
if (builder.mergeDelimitedFrom(input)) {
return builder.buildParsed();
} else {
return null;
}
}
public static org.deephacks.westty.tests.ClusterMessages.GetSendResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
Builder builder = newBuilder();
if (builder.mergeDelimitedFrom(input, extensionRegistry)) {
return builder.buildParsed();
} else {
return null;
}
}
public static org.deephacks.westty.tests.ClusterMessages.GetSendResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return newBuilder().mergeFrom(input).buildParsed();
}
public static org.deephacks.westty.tests.ClusterMessages.GetSendResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return newBuilder().mergeFrom(input, extensionRegistry)
.buildParsed();
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(org.deephacks.westty.tests.ClusterMessages.GetSendResponse prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder
implements org.deephacks.westty.tests.ClusterMessages.GetSendResponseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.deephacks.westty.tests.ClusterMessages.internal_static_westty_tests_GetSendResponse_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.deephacks.westty.tests.ClusterMessages.internal_static_westty_tests_GetSendResponse_fieldAccessorTable;
}
// Construct using org.deephacks.westty.tests.ClusterMessages.GetSendResponse.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
protoType_ = 0;
bitField0_ = (bitField0_ & ~0x00000001);
serverMsg_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.deephacks.westty.tests.ClusterMessages.GetSendResponse.getDescriptor();
}
public org.deephacks.westty.tests.ClusterMessages.GetSendResponse getDefaultInstanceForType() {
return org.deephacks.westty.tests.ClusterMessages.GetSendResponse.getDefaultInstance();
}
public org.deephacks.westty.tests.ClusterMessages.GetSendResponse build() {
org.deephacks.westty.tests.ClusterMessages.GetSendResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
private org.deephacks.westty.tests.ClusterMessages.GetSendResponse buildParsed()
throws com.google.protobuf.InvalidProtocolBufferException {
org.deephacks.westty.tests.ClusterMessages.GetSendResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(
result).asInvalidProtocolBufferException();
}
return result;
}
public org.deephacks.westty.tests.ClusterMessages.GetSendResponse buildPartial() {
org.deephacks.westty.tests.ClusterMessages.GetSendResponse result = new org.deephacks.westty.tests.ClusterMessages.GetSendResponse(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.protoType_ = protoType_;
if (((bitField0_ & 0x00000002) == 0x00000002)) {
serverMsg_ = new com.google.protobuf.UnmodifiableLazyStringList(
serverMsg_);
bitField0_ = (bitField0_ & ~0x00000002);
}
result.serverMsg_ = serverMsg_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.deephacks.westty.tests.ClusterMessages.GetSendResponse) {
return mergeFrom((org.deephacks.westty.tests.ClusterMessages.GetSendResponse)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.deephacks.westty.tests.ClusterMessages.GetSendResponse other) {
if (other == org.deephacks.westty.tests.ClusterMessages.GetSendResponse.getDefaultInstance()) return this;
if (other.hasProtoType()) {
setProtoType(other.getProtoType());
}
if (!other.serverMsg_.isEmpty()) {
if (serverMsg_.isEmpty()) {
serverMsg_ = other.serverMsg_;
bitField0_ = (bitField0_ & ~0x00000002);
} else {
ensureServerMsgIsMutable();
serverMsg_.addAll(other.serverMsg_);
}
onChanged();
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder(
this.getUnknownFields());
while (true) {
int tag = input.readTag();
switch (tag) {
case 0:
this.setUnknownFields(unknownFields.build());
onChanged();
return this;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
this.setUnknownFields(unknownFields.build());
onChanged();
return this;
}
break;
}
case 10: {
ensureServerMsgIsMutable();
serverMsg_.add(input.readBytes());
break;
}
case 1616: {
bitField0_ |= 0x00000001;
protoType_ = input.readUInt32();
break;
}
}
}
}
private int bitField0_;
// optional uint32 protoType = 202;
private int protoType_ ;
public boolean hasProtoType() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
public int getProtoType() {
return protoType_;
}
public Builder setProtoType(int value) {
bitField0_ |= 0x00000001;
protoType_ = value;
onChanged();
return this;
}
public Builder clearProtoType() {
bitField0_ = (bitField0_ & ~0x00000001);
protoType_ = 0;
onChanged();
return this;
}
// repeated string serverMsg = 1;
private com.google.protobuf.LazyStringList serverMsg_ = com.google.protobuf.LazyStringArrayList.EMPTY;
private void ensureServerMsgIsMutable() {
if (!((bitField0_ & 0x00000002) == 0x00000002)) {
serverMsg_ = new com.google.protobuf.LazyStringArrayList(serverMsg_);
bitField0_ |= 0x00000002;
}
}
public java.util.List
getServerMsgList() {
return java.util.Collections.unmodifiableList(serverMsg_);
}
public int getServerMsgCount() {
return serverMsg_.size();
}
public String getServerMsg(int index) {
return serverMsg_.get(index);
}
public Builder setServerMsg(
int index, String value) {
if (value == null) {
throw new NullPointerException();
}
ensureServerMsgIsMutable();
serverMsg_.set(index, value);
onChanged();
return this;
}
public Builder addServerMsg(String value) {
if (value == null) {
throw new NullPointerException();
}
ensureServerMsgIsMutable();
serverMsg_.add(value);
onChanged();
return this;
}
public Builder addAllServerMsg(
java.lang.Iterable values) {
ensureServerMsgIsMutable();
super.addAll(values, serverMsg_);
onChanged();
return this;
}
public Builder clearServerMsg() {
serverMsg_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
return this;
}
void addServerMsg(com.google.protobuf.ByteString value) {
ensureServerMsgIsMutable();
serverMsg_.add(value);
onChanged();
}
// @@protoc_insertion_point(builder_scope:westty.tests.GetSendResponse)
}
static {
defaultInstance = new GetSendResponse(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:westty.tests.GetSendResponse)
}
public interface AsyncPublishRequestOrBuilder
extends com.google.protobuf.MessageOrBuilder {
// optional uint32 protoType = 203;
boolean hasProtoType();
int getProtoType();
// required string msg = 1;
boolean hasMsg();
String getMsg();
}
public static final class AsyncPublishRequest extends
com.google.protobuf.GeneratedMessage
implements AsyncPublishRequestOrBuilder {
// Use AsyncPublishRequest.newBuilder() to construct.
private AsyncPublishRequest(Builder builder) {
super(builder);
}
private AsyncPublishRequest(boolean noInit) {}
private static final AsyncPublishRequest defaultInstance;
public static AsyncPublishRequest getDefaultInstance() {
return defaultInstance;
}
public AsyncPublishRequest getDefaultInstanceForType() {
return defaultInstance;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.deephacks.westty.tests.ClusterMessages.internal_static_westty_tests_AsyncPublishRequest_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.deephacks.westty.tests.ClusterMessages.internal_static_westty_tests_AsyncPublishRequest_fieldAccessorTable;
}
private int bitField0_;
// optional uint32 protoType = 203;
public static final int PROTOTYPE_FIELD_NUMBER = 203;
private int protoType_;
public boolean hasProtoType() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
public int getProtoType() {
return protoType_;
}
// required string msg = 1;
public static final int MSG_FIELD_NUMBER = 1;
private java.lang.Object msg_;
public boolean hasMsg() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
public String getMsg() {
java.lang.Object ref = msg_;
if (ref instanceof String) {
return (String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
String s = bs.toStringUtf8();
if (com.google.protobuf.Internal.isValidUtf8(bs)) {
msg_ = s;
}
return s;
}
}
private com.google.protobuf.ByteString getMsgBytes() {
java.lang.Object ref = msg_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((String) ref);
msg_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private void initFields() {
protoType_ = 0;
msg_ = "";
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
if (!hasMsg()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeBytes(1, getMsgBytes());
}
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeUInt32(203, protoType_);
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(1, getMsgBytes());
}
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(203, protoType_);
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static org.deephacks.westty.tests.ClusterMessages.AsyncPublishRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data).buildParsed();
}
public static org.deephacks.westty.tests.ClusterMessages.AsyncPublishRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data, extensionRegistry)
.buildParsed();
}
public static org.deephacks.westty.tests.ClusterMessages.AsyncPublishRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data).buildParsed();
}
public static org.deephacks.westty.tests.ClusterMessages.AsyncPublishRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data, extensionRegistry)
.buildParsed();
}
public static org.deephacks.westty.tests.ClusterMessages.AsyncPublishRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return newBuilder().mergeFrom(input).buildParsed();
}
public static org.deephacks.westty.tests.ClusterMessages.AsyncPublishRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return newBuilder().mergeFrom(input, extensionRegistry)
.buildParsed();
}
public static org.deephacks.westty.tests.ClusterMessages.AsyncPublishRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
Builder builder = newBuilder();
if (builder.mergeDelimitedFrom(input)) {
return builder.buildParsed();
} else {
return null;
}
}
public static org.deephacks.westty.tests.ClusterMessages.AsyncPublishRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
Builder builder = newBuilder();
if (builder.mergeDelimitedFrom(input, extensionRegistry)) {
return builder.buildParsed();
} else {
return null;
}
}
public static org.deephacks.westty.tests.ClusterMessages.AsyncPublishRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return newBuilder().mergeFrom(input).buildParsed();
}
public static org.deephacks.westty.tests.ClusterMessages.AsyncPublishRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return newBuilder().mergeFrom(input, extensionRegistry)
.buildParsed();
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(org.deephacks.westty.tests.ClusterMessages.AsyncPublishRequest prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder
implements org.deephacks.westty.tests.ClusterMessages.AsyncPublishRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.deephacks.westty.tests.ClusterMessages.internal_static_westty_tests_AsyncPublishRequest_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.deephacks.westty.tests.ClusterMessages.internal_static_westty_tests_AsyncPublishRequest_fieldAccessorTable;
}
// Construct using org.deephacks.westty.tests.ClusterMessages.AsyncPublishRequest.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
protoType_ = 0;
bitField0_ = (bitField0_ & ~0x00000001);
msg_ = "";
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.deephacks.westty.tests.ClusterMessages.AsyncPublishRequest.getDescriptor();
}
public org.deephacks.westty.tests.ClusterMessages.AsyncPublishRequest getDefaultInstanceForType() {
return org.deephacks.westty.tests.ClusterMessages.AsyncPublishRequest.getDefaultInstance();
}
public org.deephacks.westty.tests.ClusterMessages.AsyncPublishRequest build() {
org.deephacks.westty.tests.ClusterMessages.AsyncPublishRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
private org.deephacks.westty.tests.ClusterMessages.AsyncPublishRequest buildParsed()
throws com.google.protobuf.InvalidProtocolBufferException {
org.deephacks.westty.tests.ClusterMessages.AsyncPublishRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(
result).asInvalidProtocolBufferException();
}
return result;
}
public org.deephacks.westty.tests.ClusterMessages.AsyncPublishRequest buildPartial() {
org.deephacks.westty.tests.ClusterMessages.AsyncPublishRequest result = new org.deephacks.westty.tests.ClusterMessages.AsyncPublishRequest(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.protoType_ = protoType_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.msg_ = msg_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.deephacks.westty.tests.ClusterMessages.AsyncPublishRequest) {
return mergeFrom((org.deephacks.westty.tests.ClusterMessages.AsyncPublishRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.deephacks.westty.tests.ClusterMessages.AsyncPublishRequest other) {
if (other == org.deephacks.westty.tests.ClusterMessages.AsyncPublishRequest.getDefaultInstance()) return this;
if (other.hasProtoType()) {
setProtoType(other.getProtoType());
}
if (other.hasMsg()) {
setMsg(other.getMsg());
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
if (!hasMsg()) {
return false;
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder(
this.getUnknownFields());
while (true) {
int tag = input.readTag();
switch (tag) {
case 0:
this.setUnknownFields(unknownFields.build());
onChanged();
return this;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
this.setUnknownFields(unknownFields.build());
onChanged();
return this;
}
break;
}
case 10: {
bitField0_ |= 0x00000002;
msg_ = input.readBytes();
break;
}
case 1624: {
bitField0_ |= 0x00000001;
protoType_ = input.readUInt32();
break;
}
}
}
}
private int bitField0_;
// optional uint32 protoType = 203;
private int protoType_ ;
public boolean hasProtoType() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
public int getProtoType() {
return protoType_;
}
public Builder setProtoType(int value) {
bitField0_ |= 0x00000001;
protoType_ = value;
onChanged();
return this;
}
public Builder clearProtoType() {
bitField0_ = (bitField0_ & ~0x00000001);
protoType_ = 0;
onChanged();
return this;
}
// required string msg = 1;
private java.lang.Object msg_ = "";
public boolean hasMsg() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
public String getMsg() {
java.lang.Object ref = msg_;
if (!(ref instanceof String)) {
String s = ((com.google.protobuf.ByteString) ref).toStringUtf8();
msg_ = s;
return s;
} else {
return (String) ref;
}
}
public Builder setMsg(String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
msg_ = value;
onChanged();
return this;
}
public Builder clearMsg() {
bitField0_ = (bitField0_ & ~0x00000002);
msg_ = getDefaultInstance().getMsg();
onChanged();
return this;
}
void setMsg(com.google.protobuf.ByteString value) {
bitField0_ |= 0x00000002;
msg_ = value;
onChanged();
}
// @@protoc_insertion_point(builder_scope:westty.tests.AsyncPublishRequest)
}
static {
defaultInstance = new AsyncPublishRequest(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:westty.tests.AsyncPublishRequest)
}
public interface GetPublishRequestOrBuilder
extends com.google.protobuf.MessageOrBuilder {
// optional uint32 protoType = 204;
boolean hasProtoType();
int getProtoType();
}
public static final class GetPublishRequest extends
com.google.protobuf.GeneratedMessage
implements GetPublishRequestOrBuilder {
// Use GetPublishRequest.newBuilder() to construct.
private GetPublishRequest(Builder builder) {
super(builder);
}
private GetPublishRequest(boolean noInit) {}
private static final GetPublishRequest defaultInstance;
public static GetPublishRequest getDefaultInstance() {
return defaultInstance;
}
public GetPublishRequest getDefaultInstanceForType() {
return defaultInstance;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.deephacks.westty.tests.ClusterMessages.internal_static_westty_tests_GetPublishRequest_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.deephacks.westty.tests.ClusterMessages.internal_static_westty_tests_GetPublishRequest_fieldAccessorTable;
}
private int bitField0_;
// optional uint32 protoType = 204;
public static final int PROTOTYPE_FIELD_NUMBER = 204;
private int protoType_;
public boolean hasProtoType() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
public int getProtoType() {
return protoType_;
}
private void initFields() {
protoType_ = 0;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeUInt32(204, protoType_);
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(204, protoType_);
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static org.deephacks.westty.tests.ClusterMessages.GetPublishRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data).buildParsed();
}
public static org.deephacks.westty.tests.ClusterMessages.GetPublishRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data, extensionRegistry)
.buildParsed();
}
public static org.deephacks.westty.tests.ClusterMessages.GetPublishRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data).buildParsed();
}
public static org.deephacks.westty.tests.ClusterMessages.GetPublishRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data, extensionRegistry)
.buildParsed();
}
public static org.deephacks.westty.tests.ClusterMessages.GetPublishRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return newBuilder().mergeFrom(input).buildParsed();
}
public static org.deephacks.westty.tests.ClusterMessages.GetPublishRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return newBuilder().mergeFrom(input, extensionRegistry)
.buildParsed();
}
public static org.deephacks.westty.tests.ClusterMessages.GetPublishRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
Builder builder = newBuilder();
if (builder.mergeDelimitedFrom(input)) {
return builder.buildParsed();
} else {
return null;
}
}
public static org.deephacks.westty.tests.ClusterMessages.GetPublishRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
Builder builder = newBuilder();
if (builder.mergeDelimitedFrom(input, extensionRegistry)) {
return builder.buildParsed();
} else {
return null;
}
}
public static org.deephacks.westty.tests.ClusterMessages.GetPublishRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return newBuilder().mergeFrom(input).buildParsed();
}
public static org.deephacks.westty.tests.ClusterMessages.GetPublishRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return newBuilder().mergeFrom(input, extensionRegistry)
.buildParsed();
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(org.deephacks.westty.tests.ClusterMessages.GetPublishRequest prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder
implements org.deephacks.westty.tests.ClusterMessages.GetPublishRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.deephacks.westty.tests.ClusterMessages.internal_static_westty_tests_GetPublishRequest_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.deephacks.westty.tests.ClusterMessages.internal_static_westty_tests_GetPublishRequest_fieldAccessorTable;
}
// Construct using org.deephacks.westty.tests.ClusterMessages.GetPublishRequest.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
protoType_ = 0;
bitField0_ = (bitField0_ & ~0x00000001);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.deephacks.westty.tests.ClusterMessages.GetPublishRequest.getDescriptor();
}
public org.deephacks.westty.tests.ClusterMessages.GetPublishRequest getDefaultInstanceForType() {
return org.deephacks.westty.tests.ClusterMessages.GetPublishRequest.getDefaultInstance();
}
public org.deephacks.westty.tests.ClusterMessages.GetPublishRequest build() {
org.deephacks.westty.tests.ClusterMessages.GetPublishRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
private org.deephacks.westty.tests.ClusterMessages.GetPublishRequest buildParsed()
throws com.google.protobuf.InvalidProtocolBufferException {
org.deephacks.westty.tests.ClusterMessages.GetPublishRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(
result).asInvalidProtocolBufferException();
}
return result;
}
public org.deephacks.westty.tests.ClusterMessages.GetPublishRequest buildPartial() {
org.deephacks.westty.tests.ClusterMessages.GetPublishRequest result = new org.deephacks.westty.tests.ClusterMessages.GetPublishRequest(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.protoType_ = protoType_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.deephacks.westty.tests.ClusterMessages.GetPublishRequest) {
return mergeFrom((org.deephacks.westty.tests.ClusterMessages.GetPublishRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.deephacks.westty.tests.ClusterMessages.GetPublishRequest other) {
if (other == org.deephacks.westty.tests.ClusterMessages.GetPublishRequest.getDefaultInstance()) return this;
if (other.hasProtoType()) {
setProtoType(other.getProtoType());
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder(
this.getUnknownFields());
while (true) {
int tag = input.readTag();
switch (tag) {
case 0:
this.setUnknownFields(unknownFields.build());
onChanged();
return this;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
this.setUnknownFields(unknownFields.build());
onChanged();
return this;
}
break;
}
case 1632: {
bitField0_ |= 0x00000001;
protoType_ = input.readUInt32();
break;
}
}
}
}
private int bitField0_;
// optional uint32 protoType = 204;
private int protoType_ ;
public boolean hasProtoType() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
public int getProtoType() {
return protoType_;
}
public Builder setProtoType(int value) {
bitField0_ |= 0x00000001;
protoType_ = value;
onChanged();
return this;
}
public Builder clearProtoType() {
bitField0_ = (bitField0_ & ~0x00000001);
protoType_ = 0;
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:westty.tests.GetPublishRequest)
}
static {
defaultInstance = new GetPublishRequest(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:westty.tests.GetPublishRequest)
}
public interface GetPublishResponseOrBuilder
extends com.google.protobuf.MessageOrBuilder {
// optional uint32 protoType = 205;
boolean hasProtoType();
int getProtoType();
// repeated string serverMsg = 1;
java.util.List getServerMsgList();
int getServerMsgCount();
String getServerMsg(int index);
}
public static final class GetPublishResponse extends
com.google.protobuf.GeneratedMessage
implements GetPublishResponseOrBuilder {
// Use GetPublishResponse.newBuilder() to construct.
private GetPublishResponse(Builder builder) {
super(builder);
}
private GetPublishResponse(boolean noInit) {}
private static final GetPublishResponse defaultInstance;
public static GetPublishResponse getDefaultInstance() {
return defaultInstance;
}
public GetPublishResponse getDefaultInstanceForType() {
return defaultInstance;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.deephacks.westty.tests.ClusterMessages.internal_static_westty_tests_GetPublishResponse_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.deephacks.westty.tests.ClusterMessages.internal_static_westty_tests_GetPublishResponse_fieldAccessorTable;
}
private int bitField0_;
// optional uint32 protoType = 205;
public static final int PROTOTYPE_FIELD_NUMBER = 205;
private int protoType_;
public boolean hasProtoType() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
public int getProtoType() {
return protoType_;
}
// repeated string serverMsg = 1;
public static final int SERVERMSG_FIELD_NUMBER = 1;
private com.google.protobuf.LazyStringList serverMsg_;
public java.util.List
getServerMsgList() {
return serverMsg_;
}
public int getServerMsgCount() {
return serverMsg_.size();
}
public String getServerMsg(int index) {
return serverMsg_.get(index);
}
private void initFields() {
protoType_ = 0;
serverMsg_ = com.google.protobuf.LazyStringArrayList.EMPTY;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
for (int i = 0; i < serverMsg_.size(); i++) {
output.writeBytes(1, serverMsg_.getByteString(i));
}
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeUInt32(205, protoType_);
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
{
int dataSize = 0;
for (int i = 0; i < serverMsg_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeBytesSizeNoTag(serverMsg_.getByteString(i));
}
size += dataSize;
size += 1 * getServerMsgList().size();
}
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(205, protoType_);
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static org.deephacks.westty.tests.ClusterMessages.GetPublishResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data).buildParsed();
}
public static org.deephacks.westty.tests.ClusterMessages.GetPublishResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data, extensionRegistry)
.buildParsed();
}
public static org.deephacks.westty.tests.ClusterMessages.GetPublishResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data).buildParsed();
}
public static org.deephacks.westty.tests.ClusterMessages.GetPublishResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data, extensionRegistry)
.buildParsed();
}
public static org.deephacks.westty.tests.ClusterMessages.GetPublishResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return newBuilder().mergeFrom(input).buildParsed();
}
public static org.deephacks.westty.tests.ClusterMessages.GetPublishResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return newBuilder().mergeFrom(input, extensionRegistry)
.buildParsed();
}
public static org.deephacks.westty.tests.ClusterMessages.GetPublishResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
Builder builder = newBuilder();
if (builder.mergeDelimitedFrom(input)) {
return builder.buildParsed();
} else {
return null;
}
}
public static org.deephacks.westty.tests.ClusterMessages.GetPublishResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
Builder builder = newBuilder();
if (builder.mergeDelimitedFrom(input, extensionRegistry)) {
return builder.buildParsed();
} else {
return null;
}
}
public static org.deephacks.westty.tests.ClusterMessages.GetPublishResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return newBuilder().mergeFrom(input).buildParsed();
}
public static org.deephacks.westty.tests.ClusterMessages.GetPublishResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return newBuilder().mergeFrom(input, extensionRegistry)
.buildParsed();
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(org.deephacks.westty.tests.ClusterMessages.GetPublishResponse prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder
implements org.deephacks.westty.tests.ClusterMessages.GetPublishResponseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.deephacks.westty.tests.ClusterMessages.internal_static_westty_tests_GetPublishResponse_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.deephacks.westty.tests.ClusterMessages.internal_static_westty_tests_GetPublishResponse_fieldAccessorTable;
}
// Construct using org.deephacks.westty.tests.ClusterMessages.GetPublishResponse.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
protoType_ = 0;
bitField0_ = (bitField0_ & ~0x00000001);
serverMsg_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.deephacks.westty.tests.ClusterMessages.GetPublishResponse.getDescriptor();
}
public org.deephacks.westty.tests.ClusterMessages.GetPublishResponse getDefaultInstanceForType() {
return org.deephacks.westty.tests.ClusterMessages.GetPublishResponse.getDefaultInstance();
}
public org.deephacks.westty.tests.ClusterMessages.GetPublishResponse build() {
org.deephacks.westty.tests.ClusterMessages.GetPublishResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
private org.deephacks.westty.tests.ClusterMessages.GetPublishResponse buildParsed()
throws com.google.protobuf.InvalidProtocolBufferException {
org.deephacks.westty.tests.ClusterMessages.GetPublishResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(
result).asInvalidProtocolBufferException();
}
return result;
}
public org.deephacks.westty.tests.ClusterMessages.GetPublishResponse buildPartial() {
org.deephacks.westty.tests.ClusterMessages.GetPublishResponse result = new org.deephacks.westty.tests.ClusterMessages.GetPublishResponse(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.protoType_ = protoType_;
if (((bitField0_ & 0x00000002) == 0x00000002)) {
serverMsg_ = new com.google.protobuf.UnmodifiableLazyStringList(
serverMsg_);
bitField0_ = (bitField0_ & ~0x00000002);
}
result.serverMsg_ = serverMsg_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.deephacks.westty.tests.ClusterMessages.GetPublishResponse) {
return mergeFrom((org.deephacks.westty.tests.ClusterMessages.GetPublishResponse)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.deephacks.westty.tests.ClusterMessages.GetPublishResponse other) {
if (other == org.deephacks.westty.tests.ClusterMessages.GetPublishResponse.getDefaultInstance()) return this;
if (other.hasProtoType()) {
setProtoType(other.getProtoType());
}
if (!other.serverMsg_.isEmpty()) {
if (serverMsg_.isEmpty()) {
serverMsg_ = other.serverMsg_;
bitField0_ = (bitField0_ & ~0x00000002);
} else {
ensureServerMsgIsMutable();
serverMsg_.addAll(other.serverMsg_);
}
onChanged();
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder(
this.getUnknownFields());
while (true) {
int tag = input.readTag();
switch (tag) {
case 0:
this.setUnknownFields(unknownFields.build());
onChanged();
return this;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
this.setUnknownFields(unknownFields.build());
onChanged();
return this;
}
break;
}
case 10: {
ensureServerMsgIsMutable();
serverMsg_.add(input.readBytes());
break;
}
case 1640: {
bitField0_ |= 0x00000001;
protoType_ = input.readUInt32();
break;
}
}
}
}
private int bitField0_;
// optional uint32 protoType = 205;
private int protoType_ ;
public boolean hasProtoType() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
public int getProtoType() {
return protoType_;
}
public Builder setProtoType(int value) {
bitField0_ |= 0x00000001;
protoType_ = value;
onChanged();
return this;
}
public Builder clearProtoType() {
bitField0_ = (bitField0_ & ~0x00000001);
protoType_ = 0;
onChanged();
return this;
}
// repeated string serverMsg = 1;
private com.google.protobuf.LazyStringList serverMsg_ = com.google.protobuf.LazyStringArrayList.EMPTY;
private void ensureServerMsgIsMutable() {
if (!((bitField0_ & 0x00000002) == 0x00000002)) {
serverMsg_ = new com.google.protobuf.LazyStringArrayList(serverMsg_);
bitField0_ |= 0x00000002;
}
}
public java.util.List
getServerMsgList() {
return java.util.Collections.unmodifiableList(serverMsg_);
}
public int getServerMsgCount() {
return serverMsg_.size();
}
public String getServerMsg(int index) {
return serverMsg_.get(index);
}
public Builder setServerMsg(
int index, String value) {
if (value == null) {
throw new NullPointerException();
}
ensureServerMsgIsMutable();
serverMsg_.set(index, value);
onChanged();
return this;
}
public Builder addServerMsg(String value) {
if (value == null) {
throw new NullPointerException();
}
ensureServerMsgIsMutable();
serverMsg_.add(value);
onChanged();
return this;
}
public Builder addAllServerMsg(
java.lang.Iterable values) {
ensureServerMsgIsMutable();
super.addAll(values, serverMsg_);
onChanged();
return this;
}
public Builder clearServerMsg() {
serverMsg_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
return this;
}
void addServerMsg(com.google.protobuf.ByteString value) {
ensureServerMsgIsMutable();
serverMsg_.add(value);
onChanged();
}
// @@protoc_insertion_point(builder_scope:westty.tests.GetPublishResponse)
}
static {
defaultInstance = new GetPublishResponse(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:westty.tests.GetPublishResponse)
}
private static com.google.protobuf.Descriptors.Descriptor
internal_static_westty_tests_AsyncSendRequest_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_westty_tests_AsyncSendRequest_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_westty_tests_GetSendRequest_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_westty_tests_GetSendRequest_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_westty_tests_GetSendResponse_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_westty_tests_GetSendResponse_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_westty_tests_AsyncPublishRequest_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_westty_tests_AsyncPublishRequest_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_westty_tests_GetPublishRequest_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_westty_tests_GetPublishRequest_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_westty_tests_GetPublishResponse_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_westty_tests_GetPublishResponse_fieldAccessorTable;
public static com.google.protobuf.Descriptors.FileDescriptor
getDescriptor() {
return descriptor;
}
private static com.google.protobuf.Descriptors.FileDescriptor
descriptor;
static {
java.lang.String[] descriptorData = {
"\n)src/main/resources/META-INF/cluster.pr" +
"oto\022\014westty.tests\"3\n\020AsyncSendRequest\022\022\n" +
"\tprotoType\030\310\001 \001(\r\022\013\n\003msg\030\001 \002(\t\"$\n\016GetSen" +
"dRequest\022\022\n\tprotoType\030\311\001 \001(\r\"8\n\017GetSendR" +
"esponse\022\022\n\tprotoType\030\312\001 \001(\r\022\021\n\tserverMsg" +
"\030\001 \003(\t\"6\n\023AsyncPublishRequest\022\022\n\tprotoTy" +
"pe\030\313\001 \001(\r\022\013\n\003msg\030\001 \002(\t\"\'\n\021GetPublishRequ" +
"est\022\022\n\tprotoType\030\314\001 \001(\r\";\n\022GetPublishRes" +
"ponse\022\022\n\tprotoType\030\315\001 \001(\r\022\021\n\tserverMsg\030\001" +
" \003(\tB-\n\032org.deephacks.westty.testsB\017Clus",
"terMessages"
};
com.google.protobuf.Descriptors.FileDescriptor.InternalDescriptorAssigner assigner =
new com.google.protobuf.Descriptors.FileDescriptor.InternalDescriptorAssigner() {
public com.google.protobuf.ExtensionRegistry assignDescriptors(
com.google.protobuf.Descriptors.FileDescriptor root) {
descriptor = root;
internal_static_westty_tests_AsyncSendRequest_descriptor =
getDescriptor().getMessageTypes().get(0);
internal_static_westty_tests_AsyncSendRequest_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_westty_tests_AsyncSendRequest_descriptor,
new java.lang.String[] { "ProtoType", "Msg", },
org.deephacks.westty.tests.ClusterMessages.AsyncSendRequest.class,
org.deephacks.westty.tests.ClusterMessages.AsyncSendRequest.Builder.class);
internal_static_westty_tests_GetSendRequest_descriptor =
getDescriptor().getMessageTypes().get(1);
internal_static_westty_tests_GetSendRequest_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_westty_tests_GetSendRequest_descriptor,
new java.lang.String[] { "ProtoType", },
org.deephacks.westty.tests.ClusterMessages.GetSendRequest.class,
org.deephacks.westty.tests.ClusterMessages.GetSendRequest.Builder.class);
internal_static_westty_tests_GetSendResponse_descriptor =
getDescriptor().getMessageTypes().get(2);
internal_static_westty_tests_GetSendResponse_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_westty_tests_GetSendResponse_descriptor,
new java.lang.String[] { "ProtoType", "ServerMsg", },
org.deephacks.westty.tests.ClusterMessages.GetSendResponse.class,
org.deephacks.westty.tests.ClusterMessages.GetSendResponse.Builder.class);
internal_static_westty_tests_AsyncPublishRequest_descriptor =
getDescriptor().getMessageTypes().get(3);
internal_static_westty_tests_AsyncPublishRequest_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_westty_tests_AsyncPublishRequest_descriptor,
new java.lang.String[] { "ProtoType", "Msg", },
org.deephacks.westty.tests.ClusterMessages.AsyncPublishRequest.class,
org.deephacks.westty.tests.ClusterMessages.AsyncPublishRequest.Builder.class);
internal_static_westty_tests_GetPublishRequest_descriptor =
getDescriptor().getMessageTypes().get(4);
internal_static_westty_tests_GetPublishRequest_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_westty_tests_GetPublishRequest_descriptor,
new java.lang.String[] { "ProtoType", },
org.deephacks.westty.tests.ClusterMessages.GetPublishRequest.class,
org.deephacks.westty.tests.ClusterMessages.GetPublishRequest.Builder.class);
internal_static_westty_tests_GetPublishResponse_descriptor =
getDescriptor().getMessageTypes().get(5);
internal_static_westty_tests_GetPublishResponse_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_westty_tests_GetPublishResponse_descriptor,
new java.lang.String[] { "ProtoType", "ServerMsg", },
org.deephacks.westty.tests.ClusterMessages.GetPublishResponse.class,
org.deephacks.westty.tests.ClusterMessages.GetPublishResponse.Builder.class);
return null;
}
};
com.google.protobuf.Descriptors.FileDescriptor
.internalBuildGeneratedFileFrom(descriptorData,
new com.google.protobuf.Descriptors.FileDescriptor[] {
}, assigner);
}
// @@protoc_insertion_point(outer_class_scope)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy