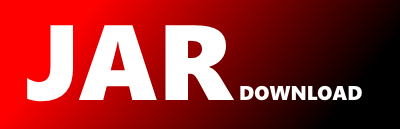
org.deephacks.graphene.Query Maven / Gradle / Ivy
/**
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.deephacks.graphene;
import com.google.common.base.Optional;
import com.sleepycat.je.Cursor;
import org.deephacks.graphene.ResultSet.DefaultResultSet;
public abstract class Query {
/**
* Set the position of the first result to retrieve. If this parameter is not
* set, the query starts from the absolute first instance.
*
* @param firstResult beginTransaction position of the first result.
* @return the same query instance
*/
public abstract Query setFirstResult(Object firstResult);
/**
* Set the position of the last result to retrieve. If this parameter is not
* set, the query continue until there are no more results or maxResults have been
* reached.
*
* @param firstResult beginTransaction position of the first result.
* @return the same query instance
*/
public abstract Query setLastResult(Object firstResult);
/**
* Set the maximum number of results to retrieve.
*
* @param maxResults max number of instances to fetch (all by default)
* @return the same query instance
*/
public abstract Query setMaxResults(int maxResults);
public abstract ResultSet retrieve();
static class DefaultQuery extends Query {
private Object firstResult;
private Object lastResult;
private int maxResults = Integer.MAX_VALUE;
private final Class entityClass;
private final Optional criteria;
private final EntityRepository repository;
DefaultQuery(Class entityClass, EntityRepository repository, Criteria criteria) {
this.entityClass = entityClass;
this.criteria = Optional.fromNullable(criteria);
this.repository = repository;
}
DefaultQuery(Class entityClass, EntityRepository repository) {
this.entityClass = entityClass;
this.criteria = Optional.absent();
this.repository = repository;
}
@Override
public Query setFirstResult(Object firstResult) {
this.firstResult = firstResult;
return this;
}
@Override
public Query setLastResult(Object lastResult) {
this.lastResult = lastResult;
return this;
}
@Override
public Query setMaxResults(int maxResults) {
this.maxResults = maxResults;
return this;
}
@Override
public ResultSet retrieve() {
Cursor cursor = repository.openPrimaryCursor();
return new DefaultResultSet<>(entityClass, firstResult, lastResult, maxResults, criteria, cursor);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy