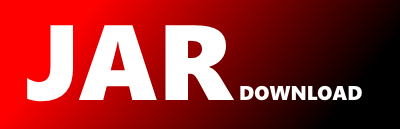
org.dellroad.stuff.vaadin7.EnumContainer Maven / Gradle / Ivy
Show all versions of dellroad-stuff-vaadin7 Show documentation
/*
* Copyright (C) 2022 Archie L. Cobbs. All rights reserved.
*/
package org.dellroad.stuff.vaadin7;
import java.util.List;
import org.dellroad.stuff.java.EnumUtil;
/**
* Container backed by the instances of an {@link Enum} type.
*
*
* Instances will auto-detect properties defined by {@link ProvidesProperty @ProvidesProperty} annotations (if any),
* and will also pre-define the following properties:
*
* - {@value #NAME_PROPERTY} - {@link String} property derived from {@link Enum#name Enum.name()}
* - {@value #VALUE_PROPERTY} - type {@code T} property that returns the {@link Enum} instance value itself
* - {@value #ORDINAL_PROPERTY} - {@link Integer} property derived from {@link Enum#ordinal Enum.ordinal()}
* - {@value #TO_STRING_PROPERTY} - {@link String} property derived from {@link Enum#toString}
*
*
* @param enum type
* @see EnumComboBox
*/
@SuppressWarnings("serial")
public class EnumContainer> extends SelfKeyedContainer {
public static final String NAME_PROPERTY = "name";
public static final String VALUE_PROPERTY = "value";
public static final String ORDINAL_PROPERTY = "ordinal";
public static final String TO_STRING_PROPERTY = "toString";
private static final PropertyDef NAME_PROPERTY_DEF = new PropertyDef<>(NAME_PROPERTY, String.class);
@SuppressWarnings("rawtypes")
private static final PropertyDef VALUE_PROPERTY_DEF = new PropertyDef<>(VALUE_PROPERTY, Enum.class);
private static final PropertyDef ORDINAL_PROPERTY_DEF = new PropertyDef<>(ORDINAL_PROPERTY, Integer.class);
private static final PropertyDef TO_STRING_PROPERTY_DEF = new PropertyDef<>(TO_STRING_PROPERTY, String.class);
/**
* Constructor.
*
* @param type enum type
* @throws IllegalArgumentException if {@code type} is null
* @throws IllegalArgumentException if {@code type} has a {@link ProvidesProperty @ProvidesProperty}-annotated method
* with no {@linkplain ProvidesProperty#value property name specified} has a name which cannot be interpreted as a bean
* property "getter" method
* @throws IllegalArgumentException if {@code type} has two {@link ProvidesProperty @ProvidesProperty}-annotated
* fields or methods with the same {@linkplain ProvidesProperty#value property name}
*/
public EnumContainer(final Class type) {
super(type);
this.setProperty(NAME_PROPERTY_DEF);
this.setProperty(VALUE_PROPERTY_DEF);
this.setProperty(ORDINAL_PROPERTY_DEF);
this.setProperty(TO_STRING_PROPERTY_DEF);
this.load(this.getExposedValues(type));
}
/**
* Get the {@link Enum} values to expose in this container in the desired order.
*
*
* The implementation in {@link EnumContainer} returns all values in their natural order.
* Subclasses may override to filter and/or re-order.
*
* @param type enum class instance
* @return instances to include in container
*/
protected List getExposedValues(Class type) {
return EnumUtil.getValues(type);
}
@Override
public V getPropertyValue(T value, PropertyDef propertyDef) {
if (propertyDef.equals(VALUE_PROPERTY_DEF))
return propertyDef.getType().cast(value);
if (propertyDef.equals(VALUE_PROPERTY_DEF))
return propertyDef.getType().cast(value);
if (propertyDef.equals(ORDINAL_PROPERTY_DEF))
return propertyDef.getType().cast(value.ordinal());
if (propertyDef.equals(TO_STRING_PROPERTY_DEF))
return propertyDef.getType().cast(value.toString());
return super.getPropertyValue(value, propertyDef);
}
}