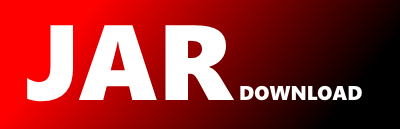
org.dellroad.msrp.Endpoint Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of msrp4j Show documentation
Show all versions of msrp4j Show documentation
msrp4j is a Java library for the MSRP protocol defined by RFC 4975.
/*
* Copyright (C) 2014 Archie L. Cobbs. All rights reserved.
*/
package org.dellroad.msrp;
import java.net.InetSocketAddress;
/**
* Combination of a host and port.
*
*
* Instances are immutable.
*
*/
public class Endpoint {
private final String host;
private final int port;
/**
* Constructor.
*
* @param host remote host
* @param port TCP port
* @throws IllegalArgumentException if {@code host} is null
* @throws IllegalArgumentException if {@code port} is not in the range 1-65535
*/
public Endpoint(String host, int port) {
if (host == null)
throw new IllegalArgumentException("null host");
if (port < 1 || port > 65535)
throw new IllegalArgumentException("invalid port " + port);
this.host = host;
this.port = port;
}
public String getHost() {
return this.host;
}
public int getPort() {
return this.port;
}
/**
* Convert this instance to a {@link InetSocketAddress}. This may result in a DNS lookup.
* If the lookup fails, the returned {@link InetSocketAddress} will be unresolved.
*/
public InetSocketAddress toSocketAddress() {
return new InetSocketAddress(this.host, this.port);
}
@Override
public String toString() {
return this.host + ":" + this.port;
}
@Override
public boolean equals(Object obj) {
if (obj == this)
return true;
if (obj == null || obj.getClass() != this.getClass())
return false;
final Endpoint that = (Endpoint)obj;
return this.host.equals(that.host) && this.port == that.port;
}
@Override
public int hashCode() {
return this.host.hashCode() ^ this.port;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy