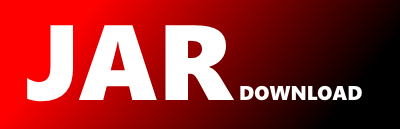
org.dellroad.querystream.jpa.PathStreamImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of querystream-jpa Show documentation
Show all versions of querystream-jpa Show documentation
Build JPA Criteria queries using a Stream-like API
The newest version!
/*
* Copyright (C) 2018 Archie L. Cobbs. All rights reserved.
*/
package org.dellroad.querystream.jpa;
import jakarta.persistence.EntityManager;
import jakarta.persistence.FlushModeType;
import jakarta.persistence.LockModeType;
import jakarta.persistence.Parameter;
import jakarta.persistence.TemporalType;
import jakarta.persistence.criteria.AbstractQuery;
import jakarta.persistence.criteria.Expression;
import jakarta.persistence.criteria.JoinType;
import jakarta.persistence.criteria.Order;
import jakarta.persistence.criteria.Path;
import jakarta.persistence.criteria.Root;
import jakarta.persistence.criteria.Selection;
import jakarta.persistence.metamodel.PluralAttribute;
import jakarta.persistence.metamodel.SingularAttribute;
import java.util.Calendar;
import java.util.Date;
import java.util.List;
import java.util.Map;
import java.util.function.Consumer;
import java.util.function.Function;
import org.dellroad.querystream.jpa.querytype.SearchType;
class PathStreamImpl> extends ExprStreamImpl implements PathStream {
// Constructors
PathStreamImpl(EntityManager entityManager, SearchType queryType,
QueryConfigurer, X, ? extends S> configurer, QueryInfo queryInfo) {
super(entityManager, queryType, configurer, queryInfo);
}
@Override
public PathStream> cast(Class type) {
return new PathStreamImpl>(this.getEntityManager(), new SearchType<>(type),
(builder, query) -> builder.treat(this.configure(builder, query), type), this.queryInfo);
}
// Narrowing overrides (SearchStreamImpl)
@Override
PathStream create(EntityManager entityManager, SearchType queryType,
QueryConfigurer, X, ? extends S> configurer, QueryInfo queryInfo) {
return new PathStreamImpl<>(entityManager, queryType, configurer, queryInfo);
}
@Override
PathValue toValue() {
return this.toValue(false);
}
@Override
PathValue toValue(boolean forceLimit) {
return new PathValueImpl<>(this.entityManager, this.queryType,
this.configurer, forceLimit ? this.queryInfo.withMaxResults(1) : this.queryInfo);
}
@Override
public PathStream distinct() {
return (PathStream)super.distinct();
}
@Override
public PathStream orderBy(Ref, ? extends Expression>> ref, boolean asc) {
return (PathStream)super.orderBy(ref, asc);
}
@Override
public PathStream orderBy(SingularAttribute super X, ?> attribute, boolean asc) {
return (PathStream)super.orderBy(attribute, asc);
}
@Override
public PathStream orderBy(SingularAttribute super X, ?> attribute1, boolean asc1,
SingularAttribute super X, ?> attribute2, boolean asc2) {
return (PathStream)super.orderBy(attribute1, asc1, attribute2, asc2);
}
@Override
public PathStream orderBy(SingularAttribute super X, ?> attribute1, boolean asc1,
SingularAttribute super X, ?> attribute2, boolean asc2, SingularAttribute super X, ?> attribute3, boolean asc3) {
return (PathStream)super.orderBy(attribute1, asc1, attribute2, asc2, attribute3, asc3);
}
@Override
public PathStream orderBy(Function super S, ? extends Expression>> orderExprFunction, boolean asc) {
return (PathStream)super.orderBy(orderExprFunction, asc);
}
@Override
public PathStream orderBy(Order... orders) {
return (PathStream)super.orderBy(orders);
}
@Override
public PathStream orderByMulti(Function super S, ? extends List extends Order>> orderListFunction) {
return (PathStream)super.orderByMulti(orderListFunction);
}
@Override
public PathStream thenOrderBy(Ref, ? extends Expression>> ref, boolean asc) {
return (PathStream)super.thenOrderBy(ref, asc);
}
@Override
public PathStream thenOrderBy(Order... orders) {
return (PathStream)super.thenOrderBy(orders);
}
@Override
public PathStream thenOrderBy(SingularAttribute super X, ?> attribute, boolean asc) {
return (PathStream)super.thenOrderBy(attribute, asc);
}
@Override
public PathStream thenOrderBy(Function super S, ? extends Expression>> orderExprFunction, boolean asc) {
return (PathStream)super.thenOrderBy(orderExprFunction, asc);
}
@Override
public PathStream groupBy(Ref, ? extends Expression>> ref) {
return (PathStream)super.groupBy(ref);
}
@Override
public PathStream groupBy(SingularAttribute super X, ?> attribute) {
return (PathStream)super.groupBy(attribute);
}
@Override
public PathStream groupBy(Function super S, ? extends Expression>> groupFunction) {
return (PathStream)super.groupBy(groupFunction);
}
@Override
public PathStream groupByMulti(Function super S, ? extends List>> groupFunction) {
return (PathStream)super.groupByMulti(groupFunction);
}
@Override
public PathStream having(Function super S, ? extends Expression> havingFunction) {
return (PathStream)super.having(havingFunction);
}
@Override
public PathValue findAny() {
return (PathValue)super.findAny();
}
@Override
public PathValue findFirst() {
return (PathValue)super.findFirst();
}
@Override
public PathValue findSingle() {
return (PathValue)super.findSingle();
}
@Override
public PathStream addRoot(Ref> ref, Class type) {
return (PathStream)super.addRoot(ref, type);
}
@Override
public PathStream fetch(SingularAttribute super X, ?> attribute) {
return (PathStream)super.fetch(attribute);
}
@Override
public PathStream fetch(SingularAttribute super X, ?> attribute, JoinType joinType) {
return (PathStream)super.fetch(attribute, joinType);
}
@Override
public PathStream fetch(PluralAttribute super X, ?, ?> attribute) {
return (PathStream)super.fetch(attribute);
}
@Override
public PathStream fetch(PluralAttribute super X, ?, ?> attribute, JoinType joinType) {
return (PathStream)super.fetch(attribute, joinType);
}
// Narrowing overrides (QueryStreamImpl)
@Override
public PathStream bind(Ref ref) {
return (PathStream)super.bind(ref);
}
@Override
public PathStream peek(Consumer super S> peeker) {
return (PathStream)super.peek(peeker);
}
@Override
public > PathStream bind(
Ref ref, Function super S, ? extends S2> refFunction) {
return (PathStream)super.bind(ref, refFunction);
}
@Override
public PathStream filter(SingularAttribute super X, Boolean> attribute) {
return (PathStream)super.filter(attribute);
}
@Override
public PathStream filter(Function super S, ? extends Expression> predicateBuilder) {
return (PathStream)super.filter(predicateBuilder);
}
@Override
public PathStream limit(int limit) {
return (PathStream)super.limit(limit);
}
@Override
public PathStream skip(int skip) {
return (PathStream)super.skip(skip);
}
@Override
public PathStream withFlushMode(FlushModeType flushMode) {
return (PathStream)super.withFlushMode(flushMode);
}
@Override
public PathStream withLockMode(LockModeType lockMode) {
return (PathStream)super.withLockMode(lockMode);
}
@Override
public PathStream withHint(String name, Object value) {
return (PathStream)super.withHint(name, value);
}
@Override
public PathStream withHints(Map hints) {
return (PathStream)super.withHints(hints);
}
@Override
public PathStream withParam(Parameter parameter, T value) {
return (PathStream)super.withParam(parameter, value);
}
@Override
public PathStream withParam(Parameter parameter, Date value, TemporalType temporalType) {
return (PathStream)super.withParam(parameter, value, temporalType);
}
@Override
public PathStream withParam(Parameter parameter, Calendar value, TemporalType temporalType) {
return (PathStream)super.withParam(parameter, value, temporalType);
}
@Override
public PathStream withParams(Iterable extends ParamBinding>> params) {
return (PathStream)super.withParams(params);
}
@Override
public PathStream withLoadGraph(String name) {
return (PathStream)super.withLoadGraph(name);
}
@Override
public PathStream withFetchGraph(String name) {
return (PathStream)super.withFetchGraph(name);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy