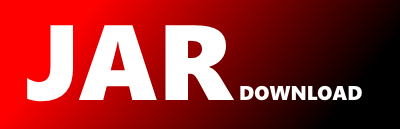
schema.flow-plan.graphql Maven / Gradle / Ivy
#
# DeltaFi - Data transformation and enrichment platform
#
# Copyright 2022 DeltaFi Contributors
#
# Licensed under the Apache License, Version 2.0 (the "License");
# you may not use this file except in compliance with the License.
# You may obtain a copy of the License at
#
# http://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS,
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
# See the License for the specific language governing permissions and
# limitations under the License.
#
enum DATA_TYPE {
STRING
BOOLEAN
NUMBER
}
type Variable {
name: String!
description: String!
dataType: DATA_TYPE!
required: Boolean!
defaultValue: String
value: String
}
input VariableInput {
name: String!
description: String!
dataType: DATA_TYPE!
required: Boolean!
defaultValue: String
value: String
}
type PluginVariables {
sourcePlugin: PluginCoordinates!
variables: [Variable]!
}
input PluginVariablesInput {
sourcePlugin: PluginCoordinatesInput!
variables: [VariableInput]!
}
type IngressFlowPlan {
name: String!
description: String!
sourcePlugin: PluginCoordinates!
type: String!
transformActions: [TransformActionConfiguration]
loadAction: LoadActionConfiguration!
}
type EgressFlowPlan {
name: String!
description: String!
sourcePlugin: PluginCoordinates!
egressAction: EgressActionConfiguration!
formatAction: FormatActionConfiguration!
validateActions: [ValidateActionConfiguration]
includeIngressFlows: [String]
excludeIngressFlows: [String]
}
type EnrichFlowPlan {
name: String!
description: String!
sourcePlugin: PluginCoordinates!
enrichActions: [EnrichActionConfiguration]!
}
input IngressFlowPlanInput {
name: String!
description: String!
sourcePlugin: PluginCoordinatesInput!
type: String!
transformActions: [TransformActionConfigurationInput]
loadAction: LoadActionConfigurationInput!
}
input EgressFlowPlanInput {
name: String!
description: String!
sourcePlugin: PluginCoordinatesInput!
egressAction: EgressActionConfigurationInput!
formatAction: FormatActionConfigurationInput!
validateActions: [ValidateActionConfigurationInput]
includeIngressFlows: [String]
excludeIngressFlows: [String]
}
input EnrichFlowPlanInput {
name: String!
description: String!
sourcePlugin: PluginCoordinatesInput!
enrichActions: [EnrichActionConfigurationInput]!
}
enum FlowState {
STOPPED
RUNNING
INVALID
}
enum FlowErrorType {
UNRESOLVED_VARIABLE
UNREGISTERED_ACTION
INACTIVE_ACTION
INVALID_ACTION_PARAMETERS
INVALID_CONFIG
}
type FlowConfigError {
configName: String!
errorType: FlowErrorType!
message: String!
}
type FlowStatus {
state: FlowState!
errors: [FlowConfigError]
}
type Flows {
sourcePlugin: PluginCoordinates!
variables: [Variable]
ingressFlows: [IngressFlow]
enrichFlows: [EnrichFlow]
egressFlows: [EgressFlow]
}
type SystemFlows {
ingress: [IngressFlow]!
enrich: [EnrichFlow]!
egress: [EgressFlow]!
}
type SystemFlowPlans {
ingressPlans: [IngressFlowPlan]!
enrichPlans: [EnrichFlowPlan]!
egressPlans: [EgressFlowPlan]!
}
type IngressFlow {
name: String!
description: String!
sourcePlugin: PluginCoordinates!
flowStatus: FlowStatus!
type: String!
transformActions: [TransformActionConfiguration]
loadAction: LoadActionConfiguration!
variables: [Variable]!
}
type EnrichFlow {
name: String!
description: String!
sourcePlugin: PluginCoordinates!
flowStatus: FlowStatus!
enrichActions: [EnrichActionConfiguration]!
variables: [Variable]!
}
type EgressFlow {
name: String!
description: String!
sourcePlugin: PluginCoordinates!
flowStatus: FlowStatus!
egressAction: EgressActionConfiguration!
formatAction: FormatActionConfiguration!
validateActions: [ValidateActionConfiguration]
includeIngressFlows: [String]
excludeIngressFlows: [String]
variables: [Variable]!
}
type ActionFamily {
family: String!
actionNames: [String]!
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy