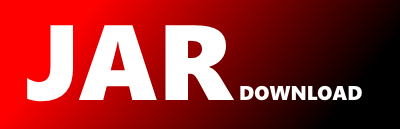
org.devocative.artemis.Immutable Maven / Gradle / Ivy
The newest version!
package org.devocative.artemis;
import java.util.*;
public class Immutable {
public static Map create(Map map) {
final Map result = new HashMap<>();
for (Map.Entry entry : map.entrySet()) {
final Object value = entry.getValue();
if (value instanceof Map) {
result.put(entry.getKey(), create((Map) value));
} else if (value instanceof List) {
result.put(entry.getKey(), create((List) value));
} else {
result.put(entry.getKey(), value);
}
}
return Collections.unmodifiableMap(result);
}
public static List> create(List> list) {
final List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy