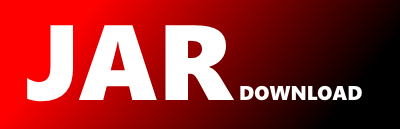
org.devocative.adroit.cache.LRUCache Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of adroit Show documentation
Show all versions of adroit Show documentation
Utility classes specially used in Demeter project
package org.devocative.adroit.cache;
import org.devocative.adroit.ObjectUtil;
import java.util.*;
public class LRUCache implements ICache {
private int capacity;
private long missHitCount;
private final Map map;
private IMissedHitHandler missedHitHandler;
// ------------------------------
public LRUCache(int capacity) {
this.capacity = capacity;
map = Collections.synchronizedMap(new CacheMap());
}
// ------------------------------
@Override
public int getCapacity() {
return capacity;
}
@Override
public void setCapacity(int capacity) {
this.capacity = capacity;
}
@Override
public long getMissHitCount() {
return missHitCount;
}
@Override
public int getSize() {
return map.size();
}
@Override
public Set getKeys() {
return Collections.unmodifiableSet(map.keySet());
}
@Override
public Collection getValues() {
return Collections.unmodifiableCollection(map.values());
}
@Override
public Set> getEntries() {
return Collections.unmodifiableSet(map.entrySet());
}
@Override
public void setMissedHitHandler(IMissedHitHandler missedHitHandler) {
this.missedHitHandler = missedHitHandler;
}
// ------------------------------
@Override
public void put(K key, V value) {
if (!map.containsKey(key) && map.size() >= capacity) {
missHitCount++;
}
map.put(key, value);
}
@Override
public synchronized void update(K key, V value) {
if (map.containsKey(key)) {
map.put(key, value);
}
}
@Override
public V remove(K key) {
return map.remove(key);
}
@Override
public void clear() {
map.clear();
missHitCount = 0;
}
// ---------------
@Override
public synchronized V get(K key) {
callMissedHitHandler(key);
return map.get(key);
}
@Override
public boolean containsKey(K key) {
return map.containsKey(key);
}
@Override
public boolean containsKeyOrFetch(K key) {
callMissedHitHandler(key);
return map.containsKey(key);
}
@Override
public V findByProperty(String property, Object value) {
for (V v : map.values()) {
Object propertyValue = ObjectUtil.getPropertyValue(v, property, true);
if (propertyValue != null && propertyValue.equals(value)) {
return v;
}
}
missHitCount++;
return null;
}
// ------------------------------
private void callMissedHitHandler(K key) {
if (missedHitHandler != null && !map.containsKey(key)) {
V v = missedHitHandler.loadForCache(key);
if (v != null) {
put(key, v);
}
}
}
// ------------------------------
private class CacheMap extends LinkedHashMap {
private static final long serialVersionUID = -3979783834148388956L;
public CacheMap() {
super(capacity, 0.75f, true);
}
@Override
protected boolean removeEldestEntry(Map.Entry eldest) {
return size() > capacity;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy